Introduction
In this project, you will learn how to create a web-based PowerPoint (PPT) presentation using front-end technologies. This type of PPT is more convenient for dissemination and viewing, and can make full use of the layout and interaction capabilities of front-end technologies.
👀 Preview
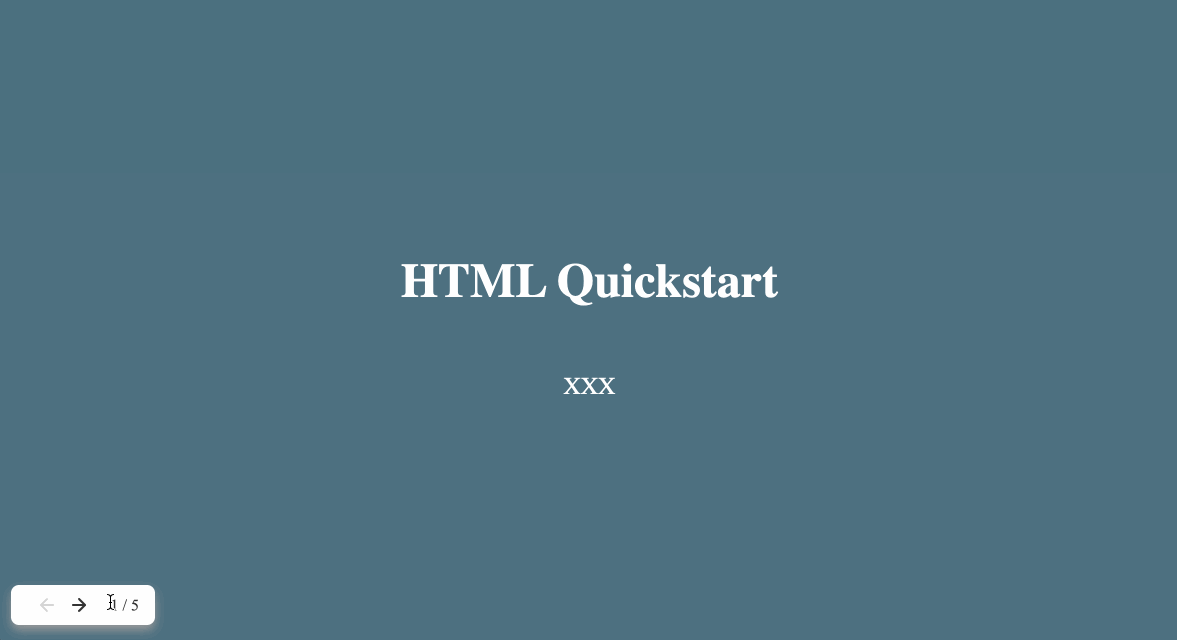
🎯 Tasks
In this project, you will learn:
- How to implement the
switchPage
function to switch between PPT pages - How to handle user input, such as keyboard presses and button clicks, to navigate through the PPT pages
- How to implement the
goLeft
andgoRight
functions to handle the navigation between the PPT pages - How to add content to the PPT slides to teach basic HTML knowledge
🏆 Achievements
After completing this project, you will be able to:
- Create a web-based PowerPoint (PPT) presentation using front-end technologies
- Manipulate the DOM to display and hide PPT pages
- Handle user input and update the UI accordingly
- Structure and present HTML-related content in a PPT format