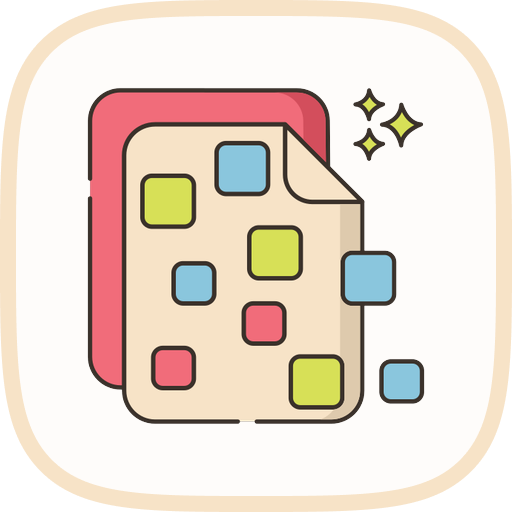
Array Attributes and Dtype
This tutorial will explore NumPy array attributes, focusing on the dtype attribute. NumPy is a powerful library for numerical computing in Python, and the NumPy array is a core data structure for this library.
NumPyPython
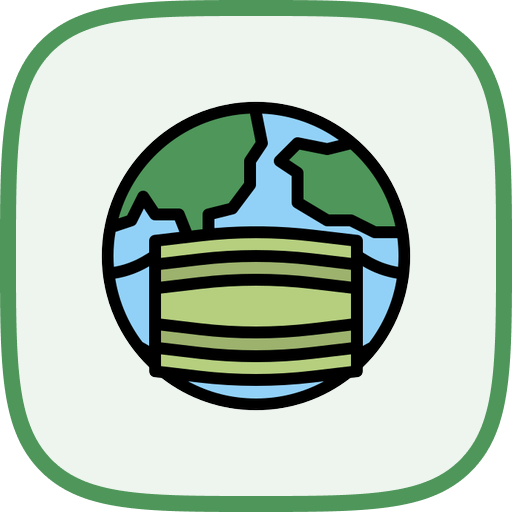
Gravitational Simulation of Earth and Super Jupiter
In this project, we will develop a gravitational simulation using Python, showcasing the interaction between Earth and a hypothetical 'Super Jupiter,' a planet with 500 times the mass of Jupiter. This simulation aims to demonstrate the impact of such a massive body on Earth's motion, considering the immense gravitational forces at play. This project suits students and hobbyists passionate about physics, astronomy, and computational simulations. To achieve this, we will employ Python libraries like NumPy for numerical calculations and Matplotlib for visualizing the dynamic movements of the planets.
MatplotlibNumPyPython
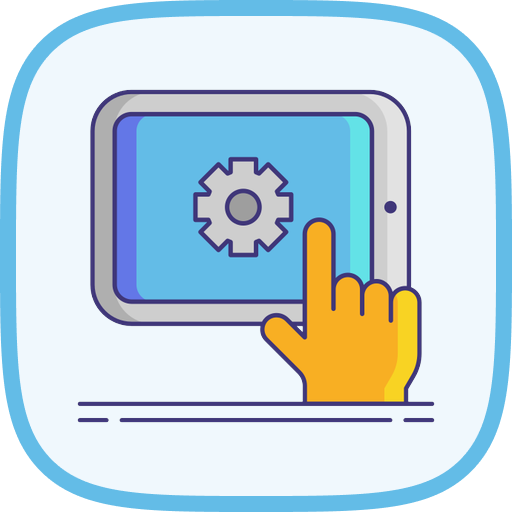
Using the Add Function
In this tutorial, we will go through the steps to use the add() function of the NumPy library. The add() function can concatenate the elements of two arrays. However, it requires that both arrays are of the same shape.
NumPyPython
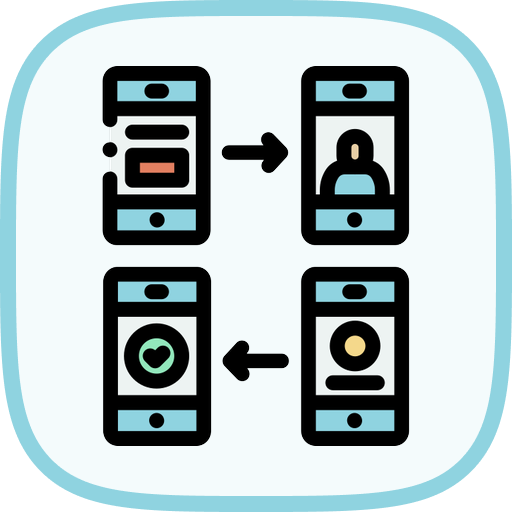
NumPy Join Function
In this lab, we will learn how to use the join() function of the NumPy library in Python. The join() function adds a separator character or string to any given string or array of strings. It is similar to the str.join() function in Python.
PythonNumPy
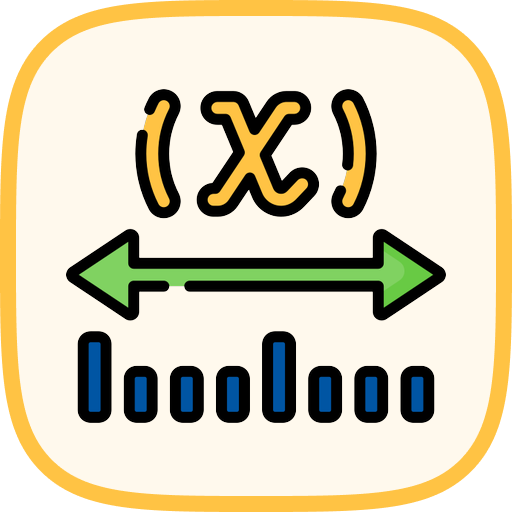
Numpy Multiply Function
In this lab, we will learn about the multiply() function in the Numpy library. This function is used to repeat a string element in an ndarray by n number of times, where n is any integer value. This function performs in an element-wise manner, covering all the array elements.
PythonNumPy
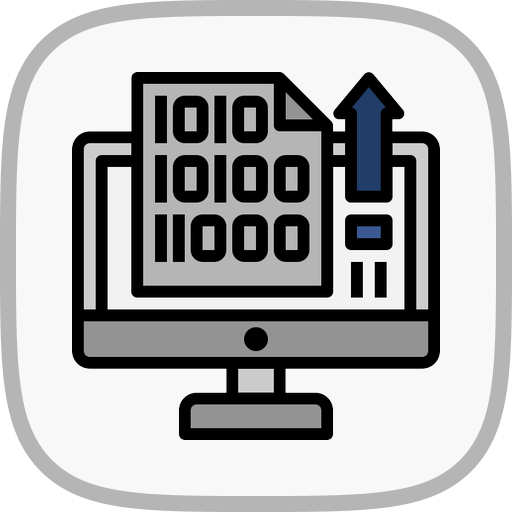
Creating a NumPy Array Using Existing Data
NumPy is a popular Python Library that provides support for Arrays. It provides various ways to create an array from the existing data. In this lab, we will learn how to create an array using existing data.
PythonNumPy
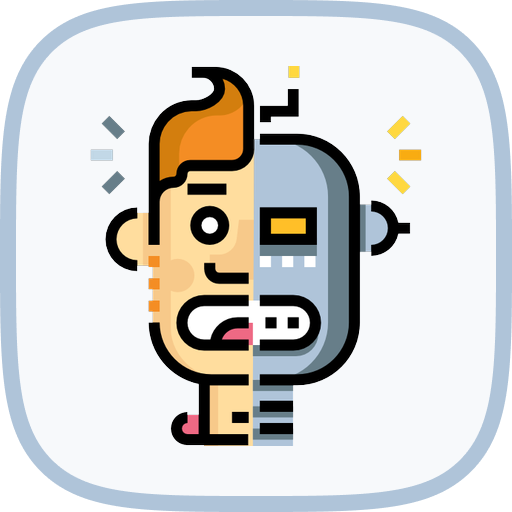
Simple Handwritten Character Recognition Classifier
In this project, you will learn how to build a simple handwritten character recognition classifier using the DIGITS dataset provided by the scikit-learn library. Handwritten character recognition is a classic problem in machine learning, and this project will guide you through the process of creating a classifier that can accurately predict the digit represented in a handwritten character image.
Machine LearningPythonscikit-learnNumPy
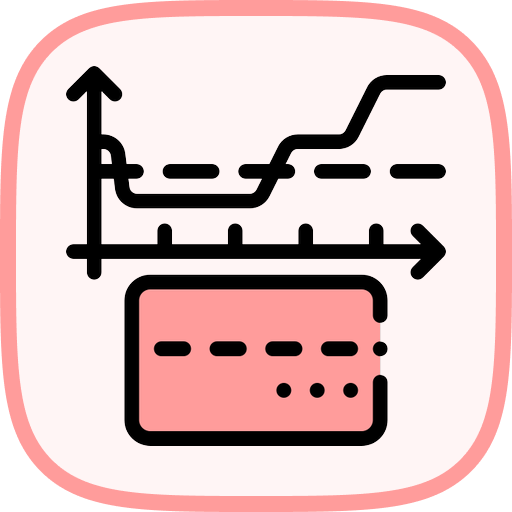
Linear Regression Fitting and Plotting
In this project, you will learn how to perform linear regression on a set of data points and visualize the results using Matplotlib. Linear regression is a fundamental machine learning technique used to model the relationship between a dependent variable (y) and one or more independent variables (x).
Machine LearningNumPyMatplotlibPython
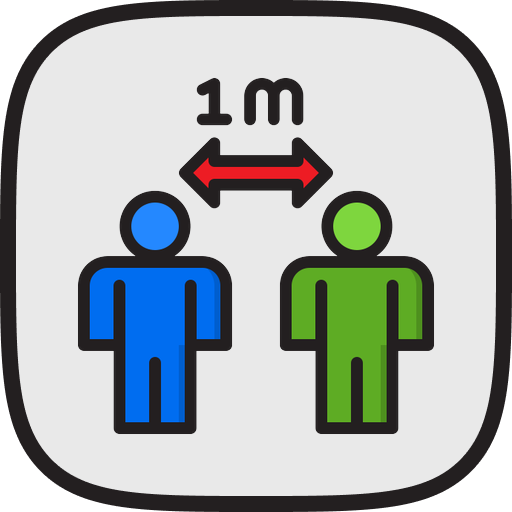
Implementing Minkowski Distance Metric
In this project, you will learn how to implement the Minkowski distance function, a generalized distance metric that includes commonly used distance measures like Euclidean and Manhattan distances. You will also learn how to test and refine the function, as well as how to integrate it into a larger project.
PythonNumPyMachine Learning
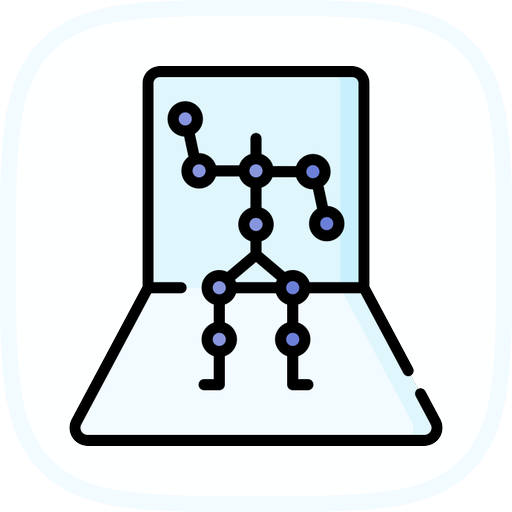
K-Nearest Neighbors Regression Algorithm Implementation
In this project, you will learn how to implement the K-Nearest Neighbors (KNN) regression algorithm using Python. KNN is a widely used machine learning method, commonly used for classification problems. However, it can also be applied to regression tasks, where the goal is to predict a continuous target value.
PythonMachine LearningNumPy
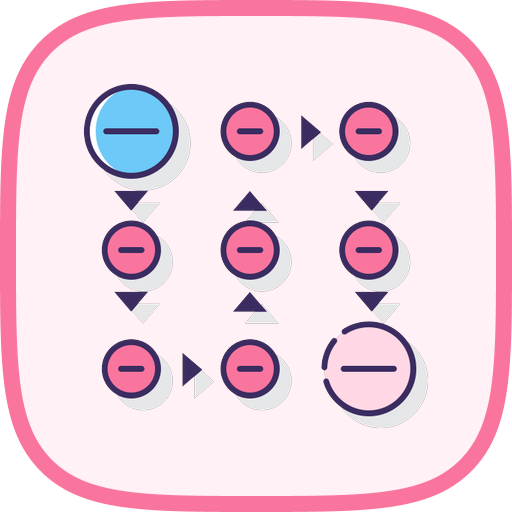
Create Confusion Matrix
In this project, you will learn how to implement a confusion matrix, which is a fundamental tool for evaluating the performance of a classification model. The confusion matrix provides a detailed breakdown of the model's predictions, allowing you to identify areas for improvement and gain valuable insights into the model's strengths and weaknesses.
Machine LearningPythonNumPy
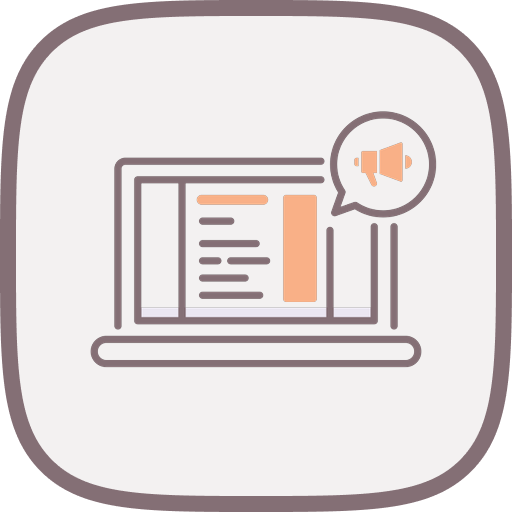
NumPy Advanced Topics
This lab will cover some of the advanced features of NumPy, including linear algebra, random number generation, and masked arrays.
NumPyPython
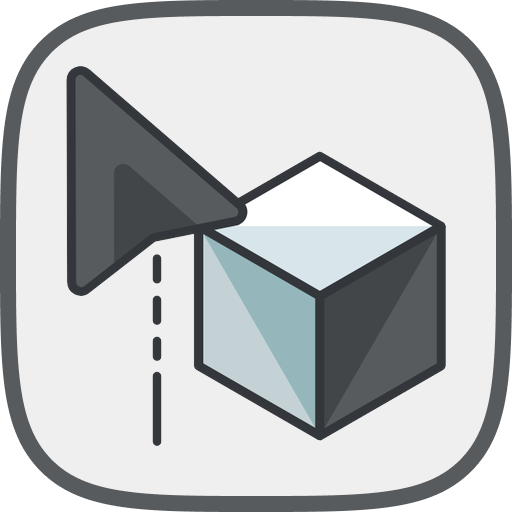
NumPy Shape Manipulation
In this lab, you will learn the NumPy shape manipulation functions that allow you to manipulate the shape of NumPy arrays.
NumPyPython
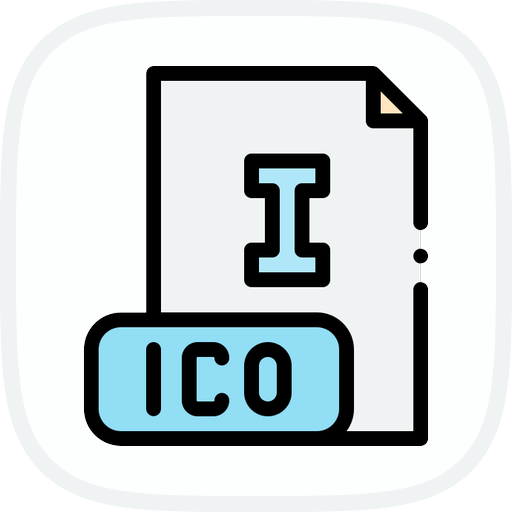
NumPy File IO
In this lab, you will learn how to use NumPy to read and write arrays to files. NumPy provides several functions for file input and output that make it easy to work with large datasets.
NumPyPython
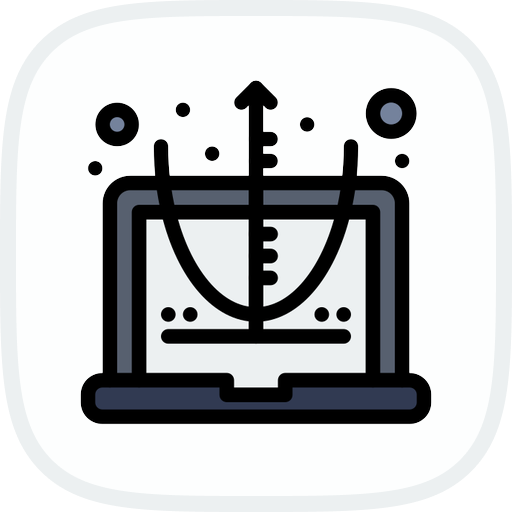
NumPy Upper Function
In this lab, you will learn how to use the upper() function in the char module of the NumPy library. This function is used to convert all lowercase characters of a string to uppercase. If there are no lowercase characters in the given string, it returns the original string. We will cover the syntax required to use this function, its returned values, and provide examples of its use.
PythonNumPy
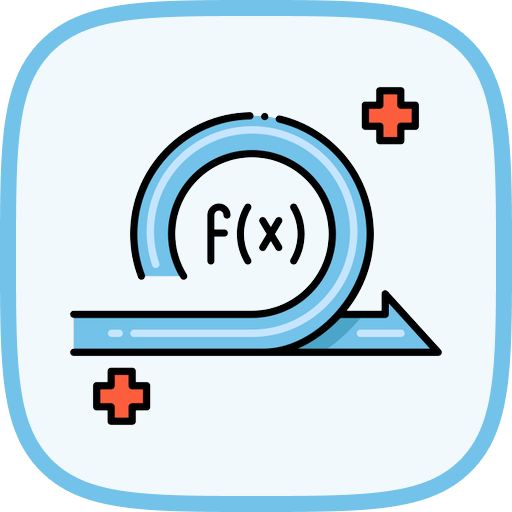
NumPy Trunc Function
In this lab, we will learn about the NumPy trunc() function in the Python language. We will understand its syntax, parameters, and how to use this function to return the truncated value of input array elements. We will also see some examples to have a better understanding.
NumPyPython
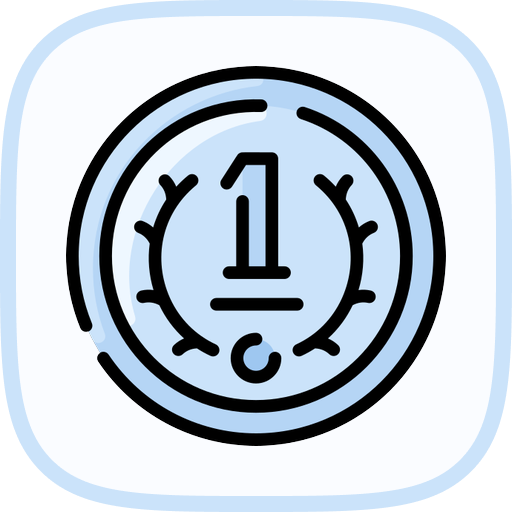
Your First NumPy Lab
Hi there, welcome to LabEx! In this first lab, you'll learn the classic 'Hello, World!' program in NumPy.
NumPyPython
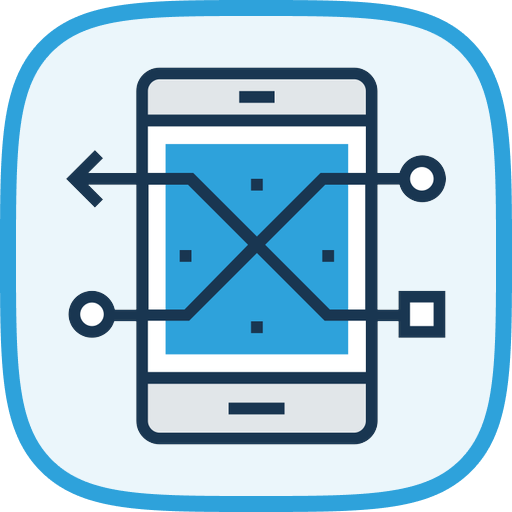
Numpy Reshape Function
The reshape() function in the NumPy library is mainly used to change the shape of an array without changing its underlying data. It helps in providing a new shape to an array that can be useful based on your use case. In this lab, we will cover the basic usage of the reshape() function and the different parameters and values it uses.
NumPyPython