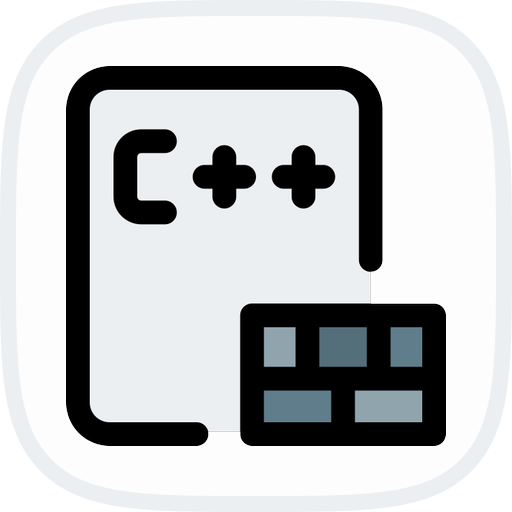
File Type Statistics Using C
This chapter is based on the file and directory interfaces of Linux. This project revolves around the nature of the file system, using the lstat function and directory operations to implement a program for recursively counting file types. It provides a convenient way to gain a deep understanding of the composition of file types in the Linux file system. Additionally, the file type counting program developed in this project can be used in practical learning and work environments.
C
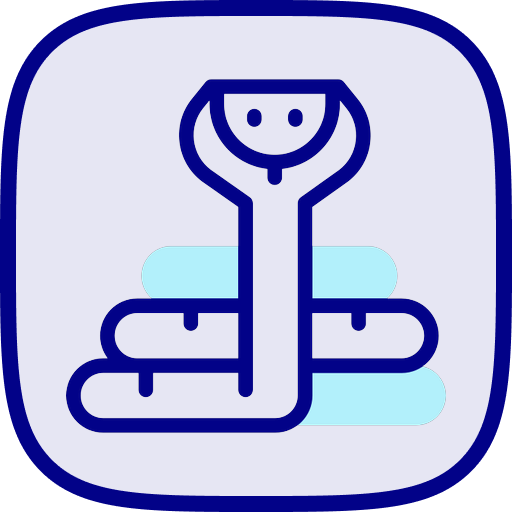
Creating a Snake Game in C
In this project, you will learn how to create a simple snake game in C using the ncurses library. This classic game involves controlling a snake to eat food, grow longer, while avoiding collisions with walls and itself. The functionality of the game is broken down into several key components: initialization, game loop, snake movement, collision detection, and so on. By the end of this project, you will have a basic snake game that can be run on a terminal.
C
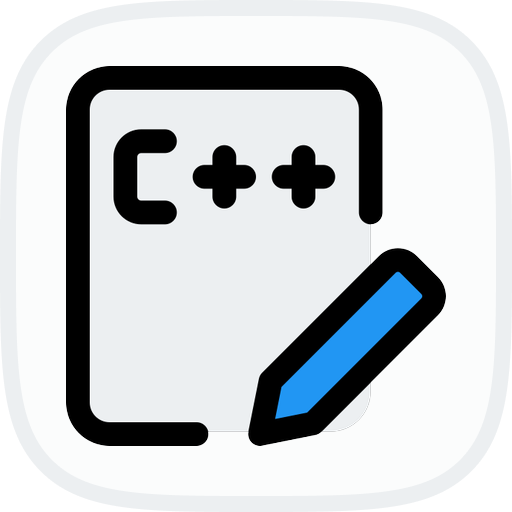
Making a Simple Calculator Using C
In this project, we will learn how to create a simple calculator using the C programming language. The calculator will be capable of performing basic arithmetic operations such as addition, subtraction, multiplication, and division. We will also implement checks to ensure that the user input is valid and handle cases where the input leads to undefined behavior.
C
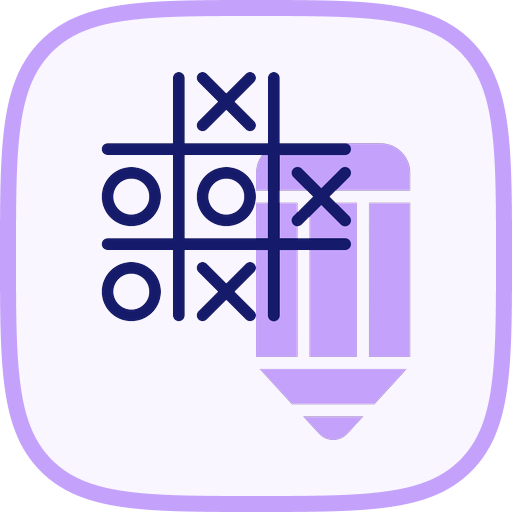
Creating a Simple Tic-Tac-Toe Game in C
In this project, you will learn how to create a simple Tic-Tac-Toe game in C. The game will be played between two players taking turns to mark the spaces in a 3x3 grid. The first player to have three of their marks in a row, column, or diagonal is the winner. If all spaces are filled and no player has three marks in a row, the game is a draw.
C
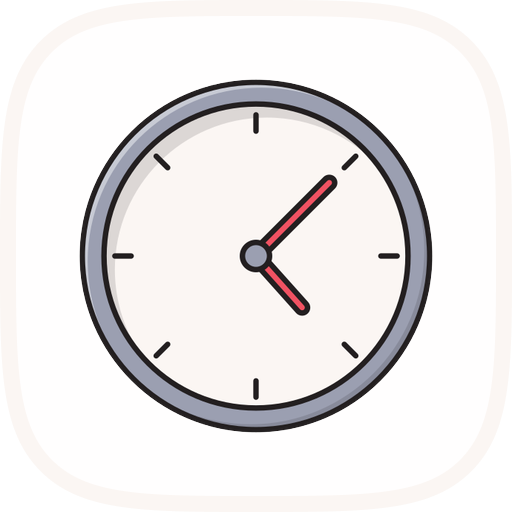
Creating a Simple Clock Animation Using OpenGL
In this project, we will create a simple clock animation using OpenGL and GLUT (Graphics Library Utility Toolkit). This animation will display a clock with moving clock hands to represent the current time. The clock will update in real-time, simulating the movement of the hour, minute, and second hands. We will start by setting up the project files and then proceed with the necessary code.
C
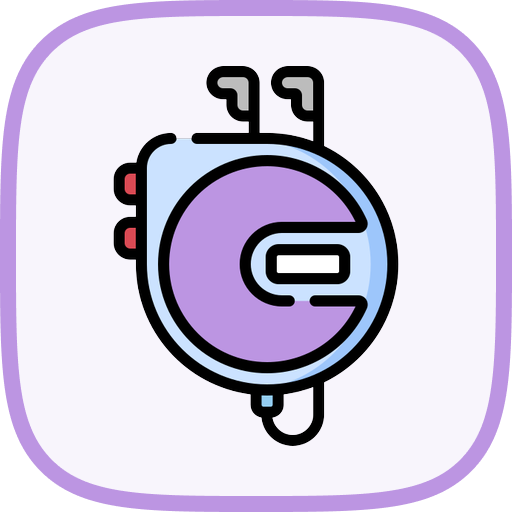
Creating a Gomoku Game in C
In this project, we will create a simple text-based Gomoku game using the C programming language. Gomoku is a two-player strategy board game where the objective is to be the first to get five consecutive pieces in a row, either horizontally, vertically, or diagonally. We will develop this game using a 15x15 game board.
C
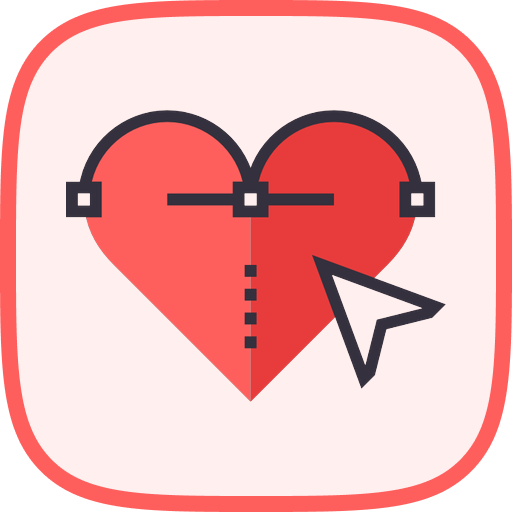
Creating a Dynamic Heart Animation with C
In this project, you will learn how to create a mesmerizing dynamic heart animation using the C programming language. The project utilizes the X Window System to render animated visuals. By following the step-by-step instructions, you will set up the project, generate data, and create a captivating animation that brings a dynamic heart to life on your screen.
C
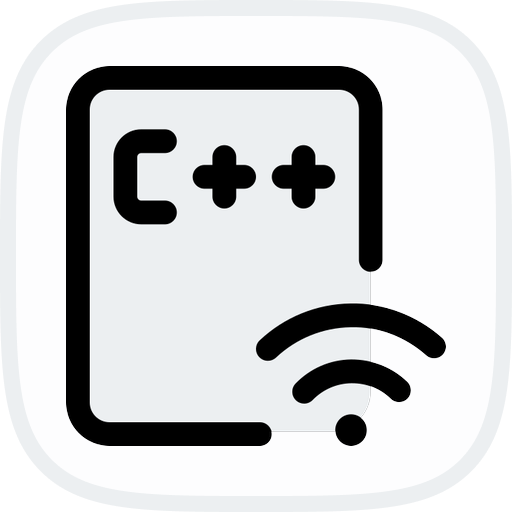
Creating Terminal Code Rain with C and Ncurses
In this project, you will learn how to create a simple code rain using the ncurses library in the C programming language. Ncurses is a library that facilitates text-based user interfaces in the terminal. This project will guide you through setting up the project, initializing the necessary components, and implementing the code rain.
C
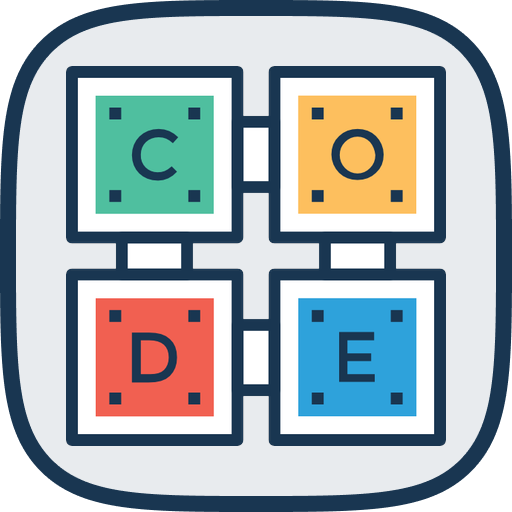
Creating a 2048 Game in C
2048 is a popular number puzzle game where the goal is to reach the 2048 tile by merging adjacent tiles with the same number. In this project, you'll learn how to create a simple 2048 game in C. We'll provide step-by-step instructions to build the game, from initializing the board to implementing game logic and running the game.
C
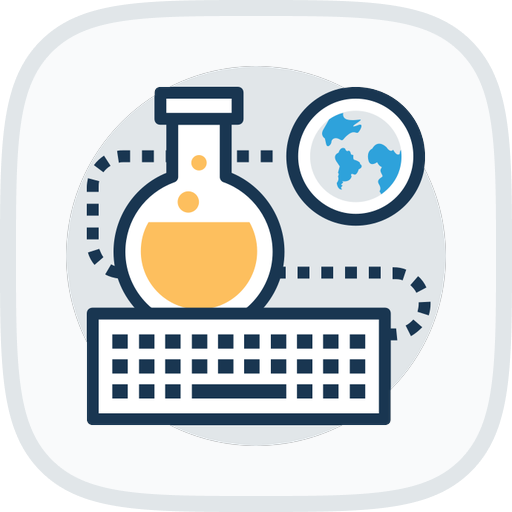
Your First C Lab
Dive into C programming with this beginner-friendly lab. Learn to write, compile, and run your first C programs, covering basics like output, variables, and string manipulation.
C
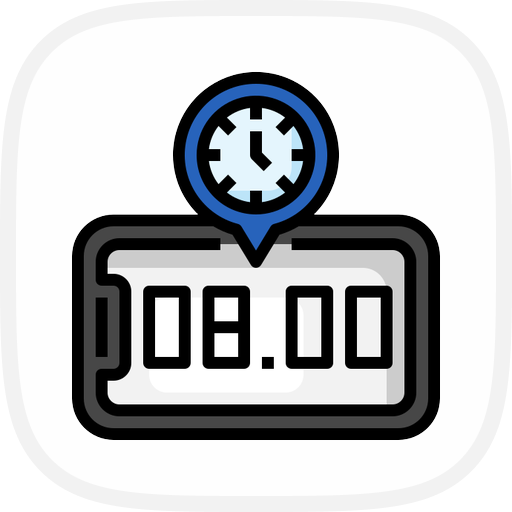
Create a Simple Stopwatch App Using GTK
In this project, we will create a simple stopwatch application using the GTK library in C. This stopwatch will have a start/pause button and a reset button to control the stopwatch timer. We will break down the process into several steps, starting with setting up the project files and ending with running the stopwatch application.
C
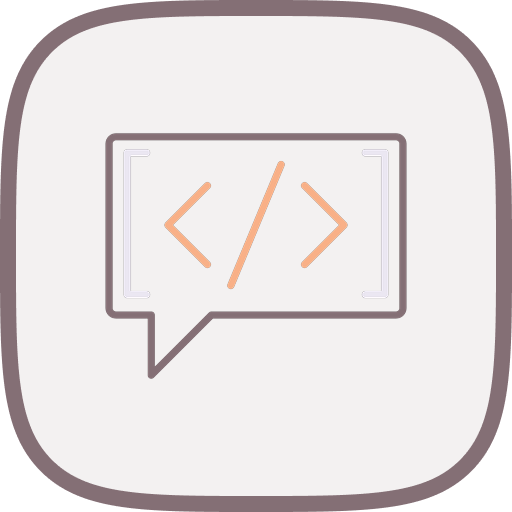
Parsing Command Line Arguments in C
In this project, you will learn how to parse and handle command line arguments in a C program. This is a fundamental skill in software development, as many programs accept and process command line arguments to customize their behavior.
C
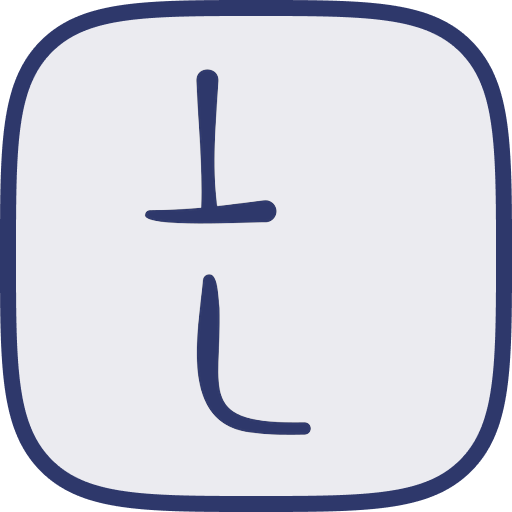
Reverse a String Using Pointer
Pointers provide direct access to memory, and by using pointers, we can access and manipulate the values and addresses of variables and arrays in memory. The program we will build in this lab will use pointer variables to reverse a given string.
C
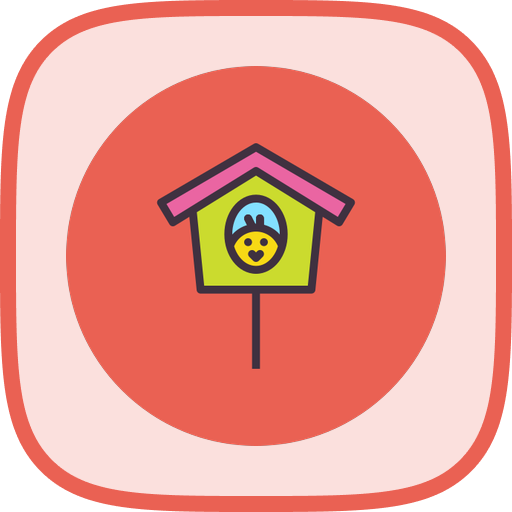
Building Flappy Bird Using C
Flappy Bird is a popular and addictive mobile game that gained immense popularity for its simple yet challenging gameplay. In this project, we will learn how to implement our own version of Flappy Bird using the C programming language.
C
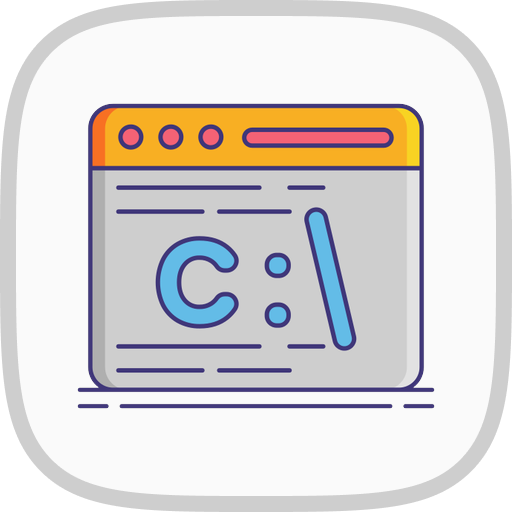
Use of Gets Function in C Programming
In C Programming language, gets() function is used to take input from the user. Unlike scanf(), gets() reads a whole line of text, stops reading when Enter key is pressed, and does not discard the newline character.
C
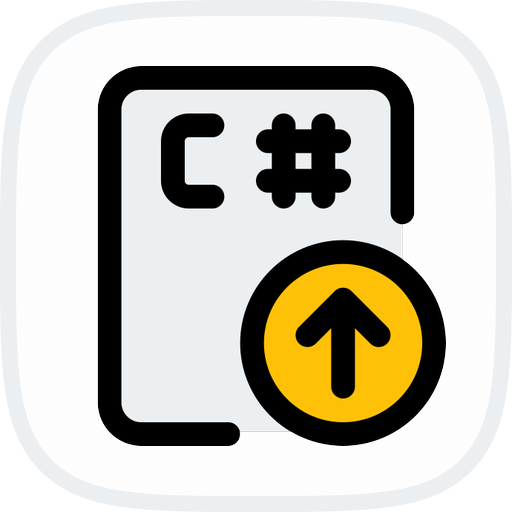
Sum of N Input Numbers in C
This lab will guide you on how to write a program in C that sums up N input numbers. We will use a loop to get the sum of all input numbers and display the result.
C
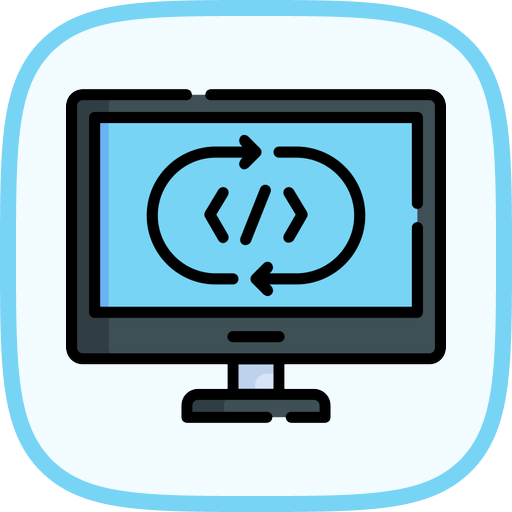
Creating While Loop Programs in C
In this lab, we will learn how to create a while loop program in C language. A while loop is used in programming to execute a block of code repeatedly until the specified condition becomes false. This loop is used when we don't know beforehand how many times the loop will run.
C
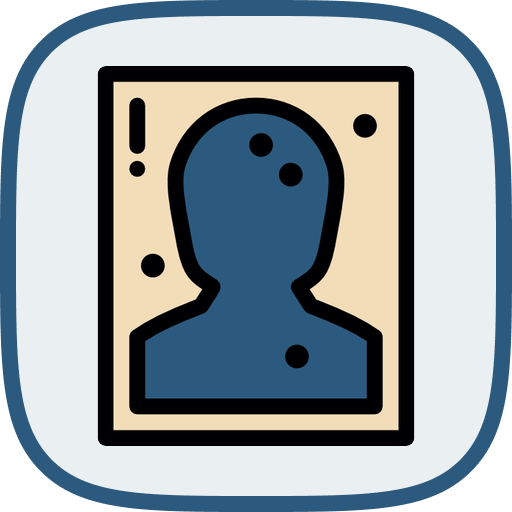
Reversing Case of Character
This lab will guide you through the process of writing a C program to reverse the case of input character. This program will take a user input character and convert it to its opposite case (lowercase to uppercase or uppercase to lowercase).
C