Introduction
In this project, you will learn how to use MyBatis to retrieve course information from a database and map the results to a custom Java object. You will also learn how to handle inconsistencies between the database table structure and the entity class properties.
👀 Preview
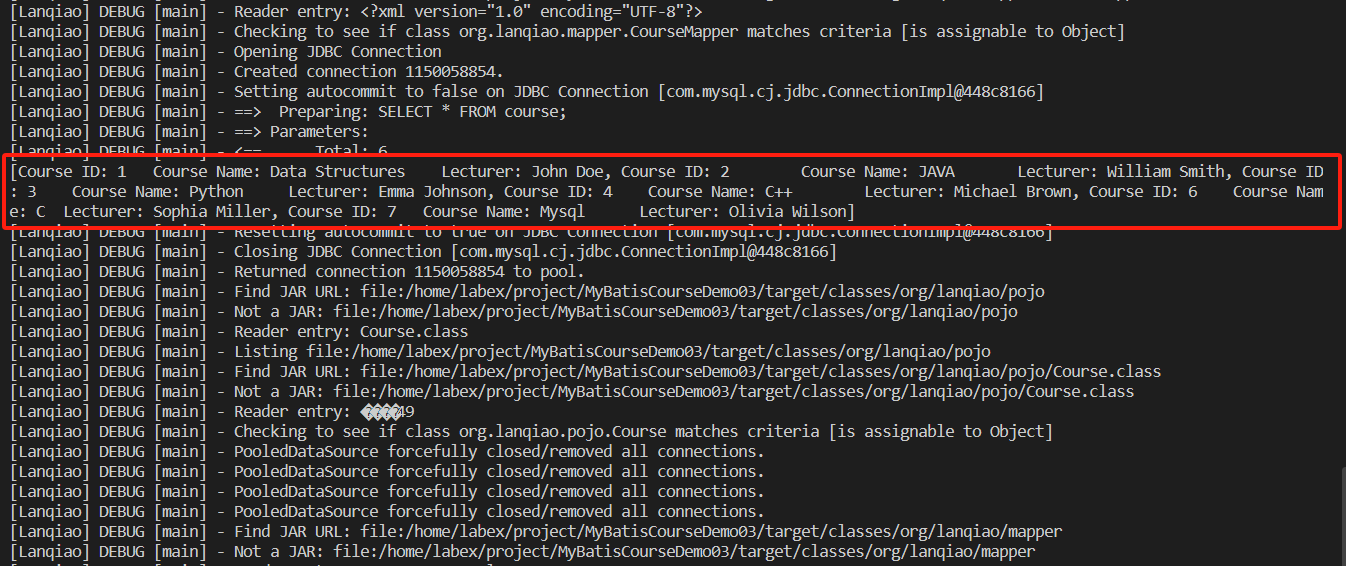
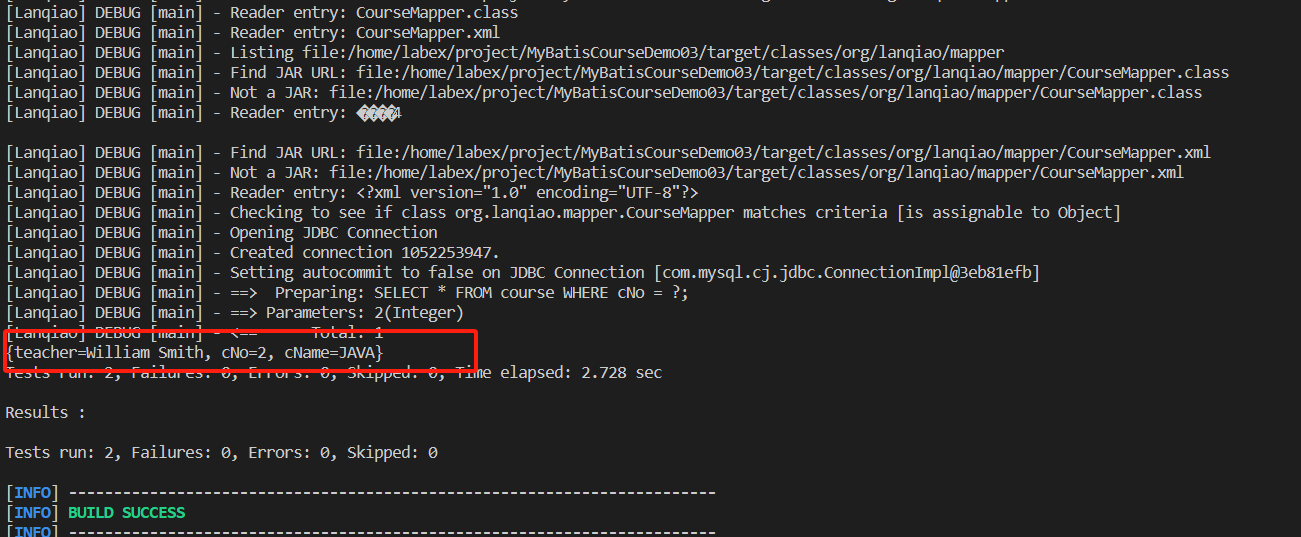
🎯 Tasks
In this project, you will learn:
- How to modify the properties and methods of an entity class to match the database table structure.
- How to configure the mapper interface to define the methods for querying course information.
- How to configure the mapper XML file to define the SQL queries and the result mapping.
- How to implement test cases to verify the functionality of the mapper.
🏆 Achievements
After completing this project, you will be able to:
- Use MyBatis to interact with a database and retrieve data.
- Use
resultMap
to handle inconsistencies between database table structure and entity class properties. - Write test cases to verify the functionality of a MyBatis mapper.