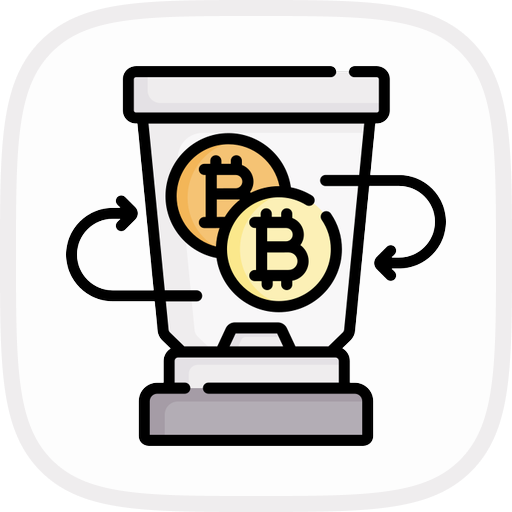
Pandas Series Bfill Method
In this lab, we will learn about the Python Pandas Series bfill() method. This method is used to fill missing values or null values in a pandas Series backward. It returns a new Series with the missing values filled, or None if the inplace parameter is set to True.
PandasPython
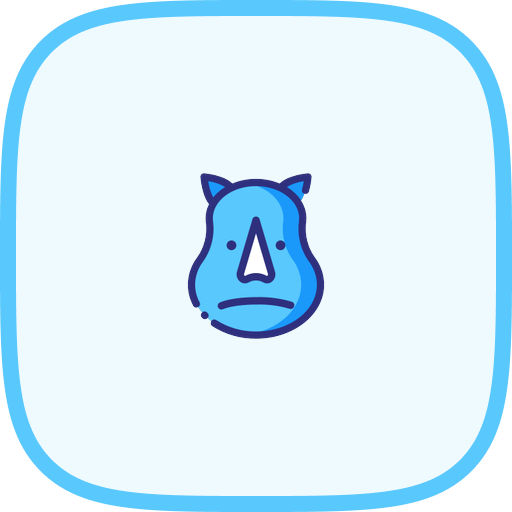
Pandas Series Astype Method
The astype() method in Python's pandas library is used to convert the data type of a pandas Series object. It allows us to change the data type of the Series to a specified data type. This lab will guide you through the usage of the astype() method in pandas.
PandasPython
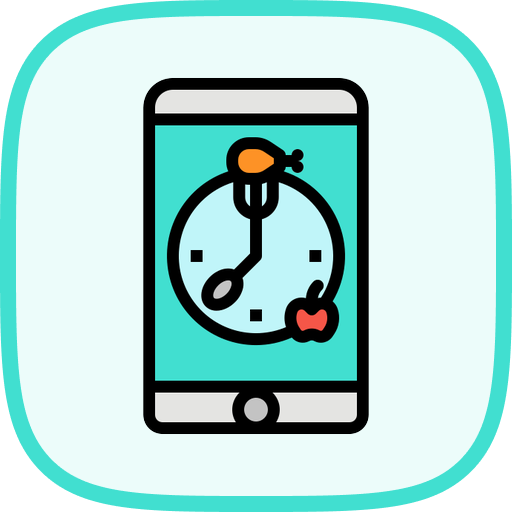
Pandas Series At_time Method
In this lab, we will explore the at_time() method in the Pandas library for Python. This method allows us to select specific values from a Pandas Series based on a particular time of the day.
PandasPython
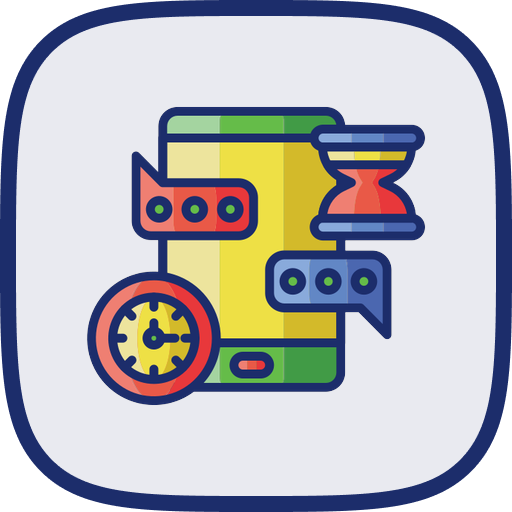
Pandas Series Between_time Method
The Pandas between_time() method is used to select values between specified times of the day in a Pandas Series object. It returns a new Series object consisting of the values within the specified time range. If the index is not a DatetimeIndex, it raises a TypeError.
PandasPython
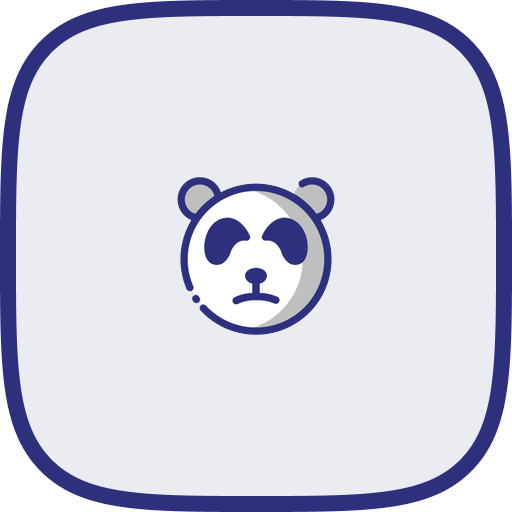
Your First Pandas Lab
Hi there, welcome to LabEx! In this first lab, you'll learn the classic 'Hello, World!' program in Pandas.
PandasPython
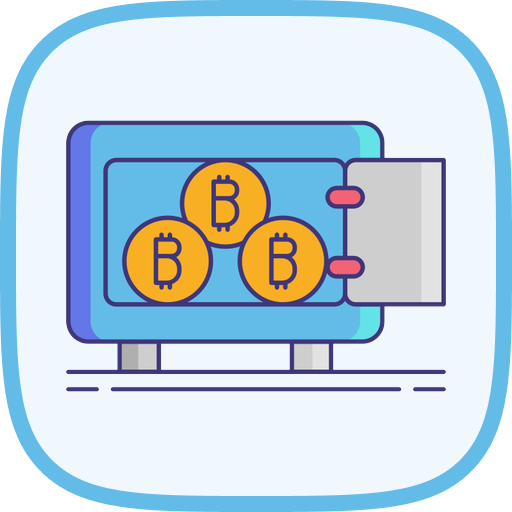
Pandas Series Bool Method
In this lab, we will learn how to use the Series.bool() method in Python Pandas. This method allows us to check whether a Series contains a single boolean value or not. It returns the boolean value present in the Series or raises an error if the Series contains more than one element or if the element is not boolean.
PandasPython
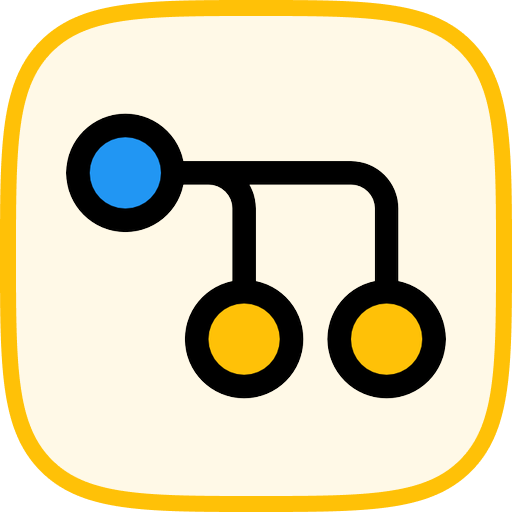
Pandas Series Clip Method
In this lab, we will learn about the clip() method in the Python Pandas library. The clip() method is used to limit or trim the values in a Pandas Series by specifying upper and lower thresholds. It replaces values that are outside the clip boundaries and can also be used to modify the Series in place.
PythonPandas
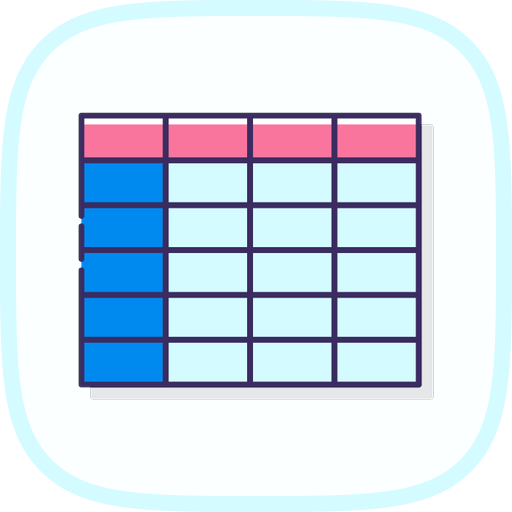
Combining Data Tables in Pandas
In this lab, we will work with air quality data to explore how to combine multiple tables using Python's Pandas library. We will be using the concat and merge functions to perform these operations. This lab will help you understand how to concatenate and merge data frames effectively.
PythonPandas
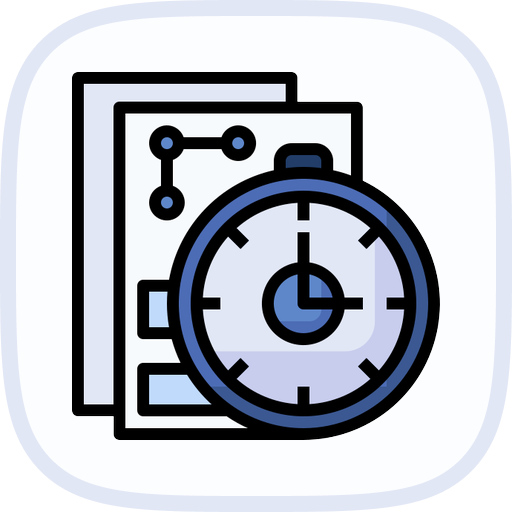
Handling Time Series Data
This lab will guide you through handling time series data using the Python package, Pandas. We will be working with air quality data for this tutorial. You will learn how to convert strings into datetime objects, perform operations on these datetime objects, resample time series to another frequency, and more.
PythonPandas
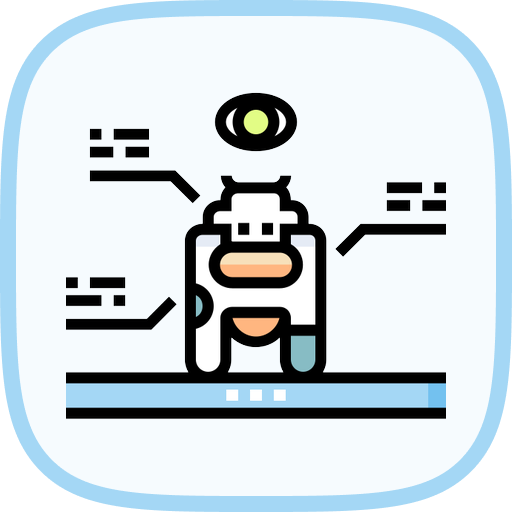
Titanic Passenger Data Analysis with Pandas
In this lab, we will learn how to use Python's Pandas library to calculate summary statistics for data. We will use the Titanic dataset, which contains data on passengers from the Titanic shipwreck. We will learn how to calculate summary statistics, aggregate statistics, and count the number of records by category.
PythonPandas
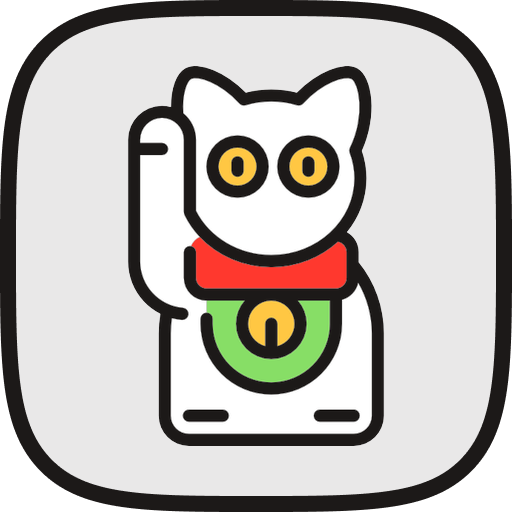
Introduction to Pandas
In this lab, we will introduce you to the basics of pandas, a powerful data manipulation library in Python. We will guide you through various tasks such as importing pandas, creating and viewing data, data selection, operations and much more.
PythonPandas
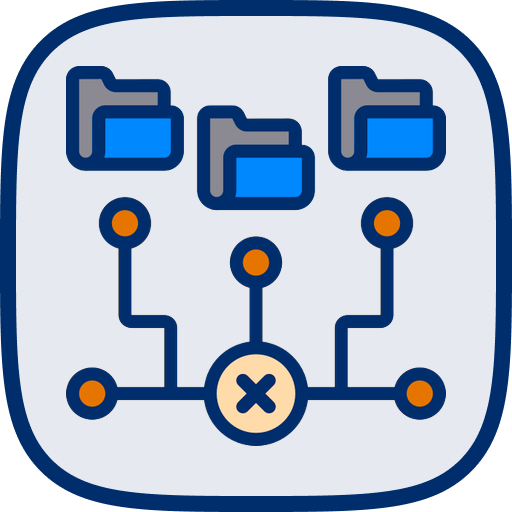
Working with Nullable Boolean Data
In this lab, we will explore the Nullable Boolean data type, provided by the Pandas library in Python. We will learn how to use this feature in indexing and logical operations, and how it differs from traditional boolean operations due to the presence of 'NA' values.
PythonPandas
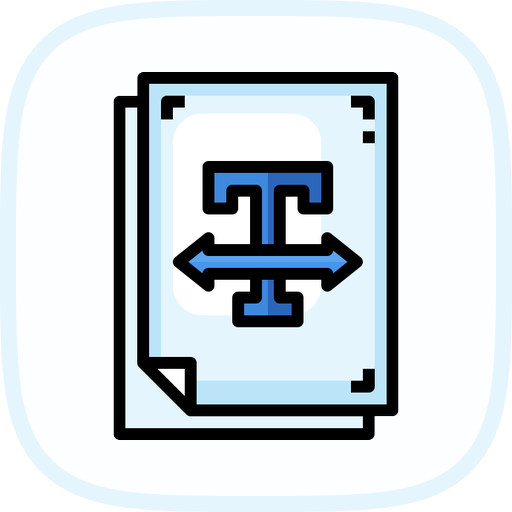
Pandas Copy-On-Write Implementation Guide
This lab provides a step-by-step guide on understanding and implementing the concept of Copy-On-Write (CoW) in Python Pandas. CoW is an optimization strategy that enhances performance and memory usage by delaying copies as long as possible. It also helps in avoiding accidental modifications of more than one object.
PythonPandas
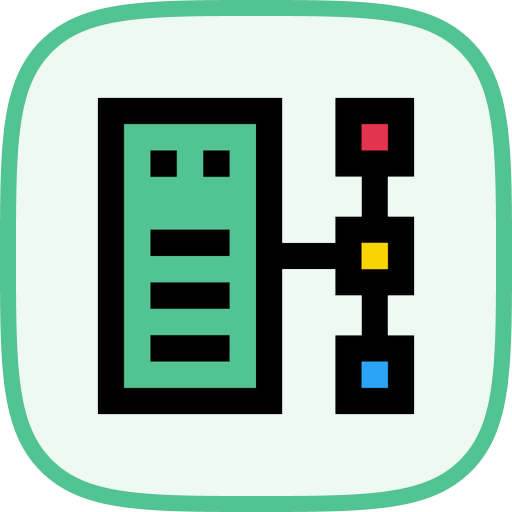
Working with Data Structures in Pandas
Pandas is a powerful Python library for data manipulation and analysis. Its fundamental data structures, Series and DataFrame, allow you to store and manipulate structured data. This lab will provide a step-by-step guide on how to work with these data structures, from creation to manipulation and alignment.
PythonPandas
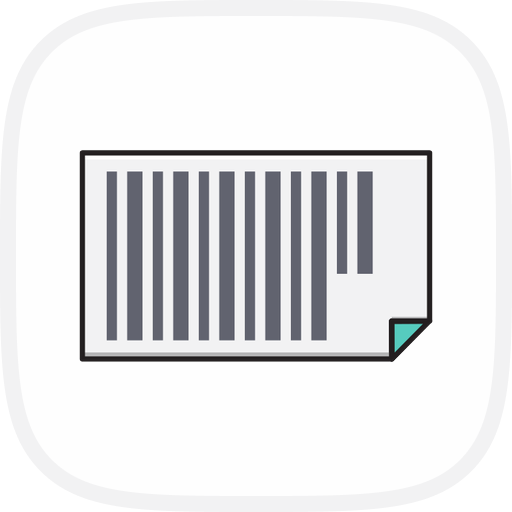
Handling Duplicate Labels
In this lab, we will learn how to handle duplicate labels in pandas. Pandas is a powerful data manipulation library in Python. Often, we encounter data with duplicate row or column labels, and it's crucial to understand how to detect and handle these duplicates.
PythonPandas
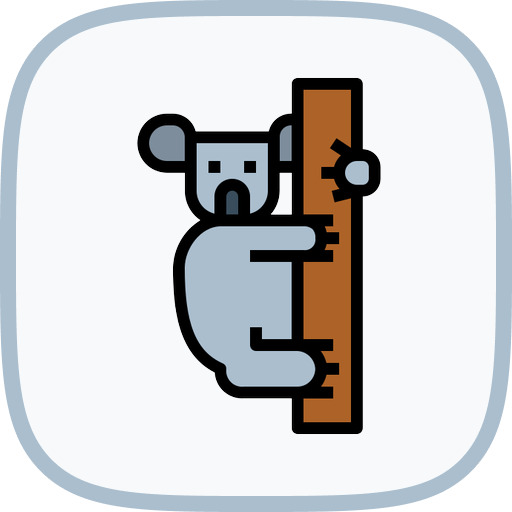
Working with Columns in Pandas
In this lab, we will learn how to work with columns in Pandas. We will explore how to create new columns derived from existing ones, apply mathematical and logical operations on columns, rename column labels, and perform column-wise operations using the apply method.
PythonPandas
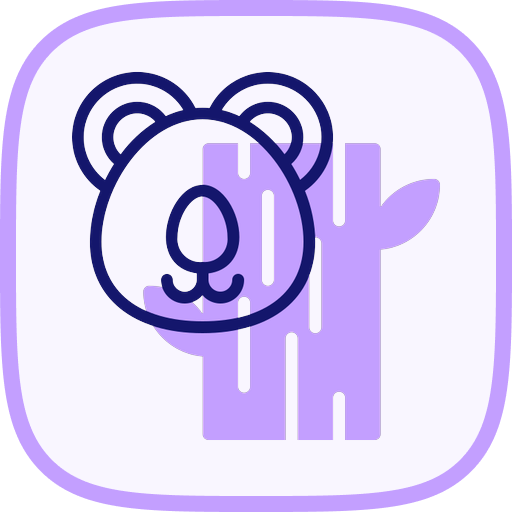
Data Selection in Pandas
In this lab, we are going to learn how to select specific data from a DataFrame using Pandas, a popular data analysis and manipulation library in Python. We will use the Titanic dataset for this tutorial.
PythonPandas
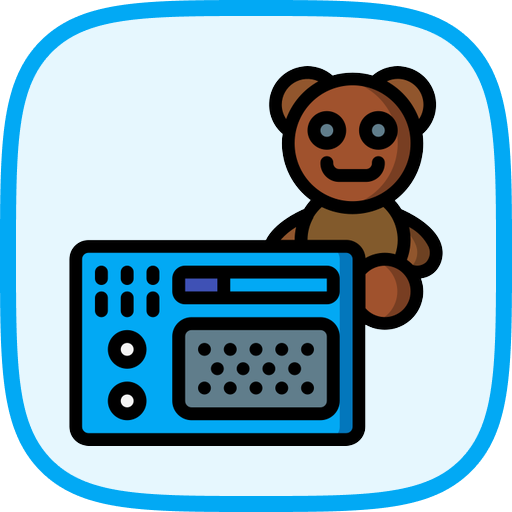
Pandas Data Manipulation
This lab will guide you on how to read, write, and manipulate data using Pandas, a powerful data analysis and manipulation library for Python. We will use a dataset from the Titanic shipwreck for this exercise.
PythonPandas