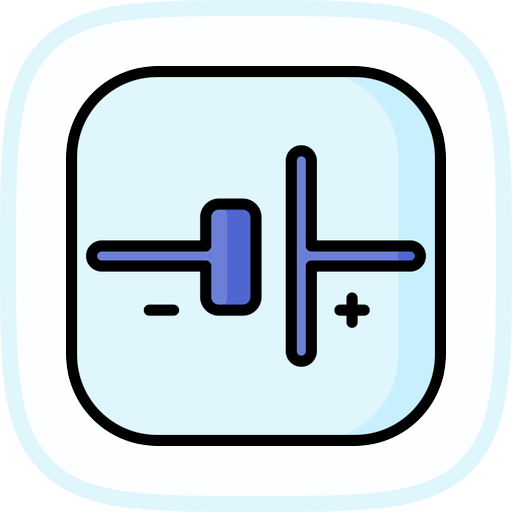
Controlling Symbols and Combining Submodules
Understand package import complexity, control exported symbols with `__all__`, export everything from the package, and split modules for better code organization in Python.
Python
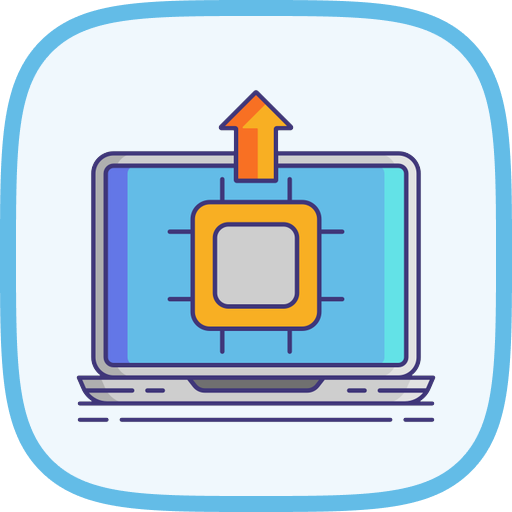
Circular and Dynamic Module Imports
Understand the import problem, explore circular imports, implement subclass registration, and use dynamic imports in Python for circular and dynamic module imports.
Python
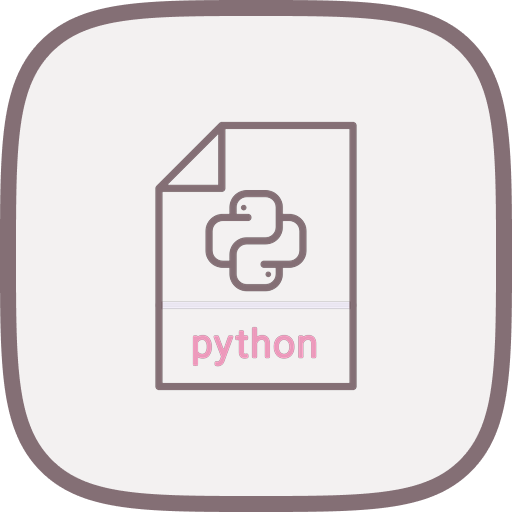
Your First Python Lab
Welcome to LabEx! In this introductory lab, you'll explore Python fundamentals including the Python interpreter, variables, data types, input/output operations, and basic programming concepts.
Python
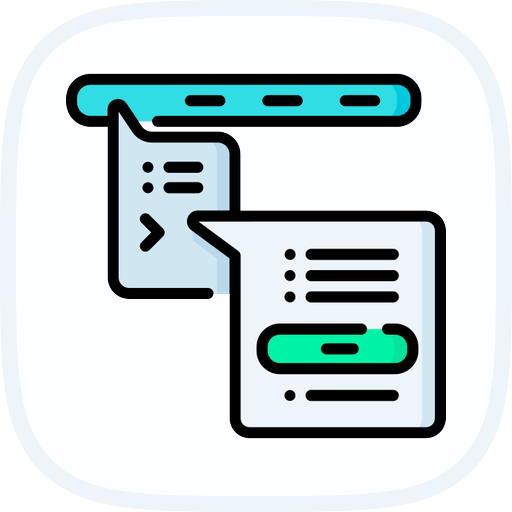
Python Assignment Expressions
In this lab, we will learn about Python Assignment Expressions, also known as the 'walrus operator' (:=). This operator, introduced in Python 3.8, allows you to assign a value to a variable as part of an expression. It is particularly useful for optimizing code, avoiding redundant calculations, and simplifying complex expressions.
Python
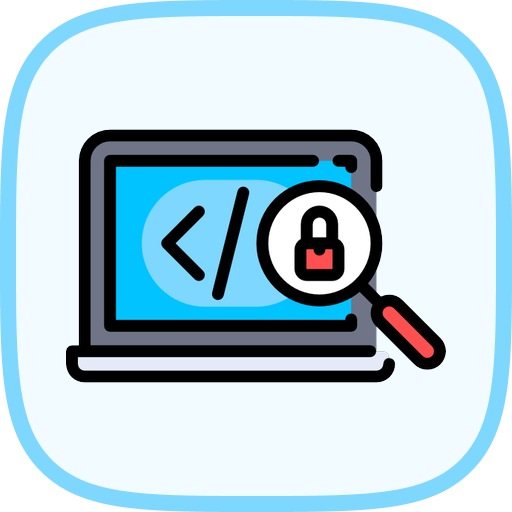
Testing Your Code
In this lab, we will learn how to write unit tests for our Python code using the built-in unittest module. We will start with a simple function and then build up to testing more complex code, including code that interacts with databases.
Python
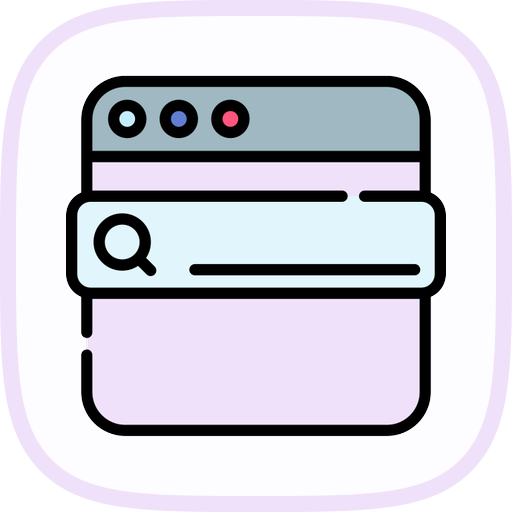
Webbrowser Package Basic
The webbrowser module in Python provides a simple interface to open web browsers, display HTML documents, and navigate the web. This practical lab will walk you through the basics of using the webbrowser package, from opening a URL in a new tab to executing a Google search directly from the Python console.
Python
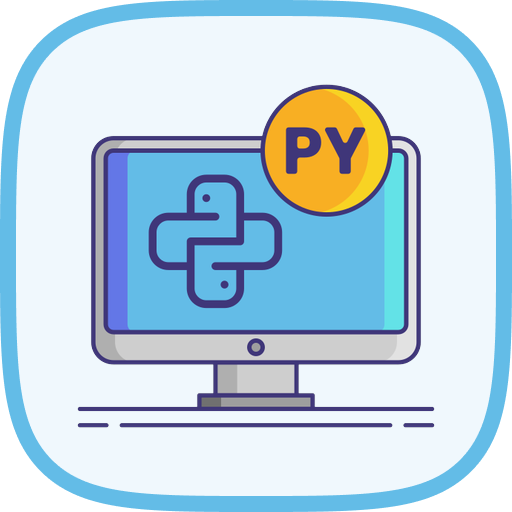
Create a Python Package
Understand Python packages, create the package structure, fix import statements, and update and test the stock.py program to build a Python package.
Python
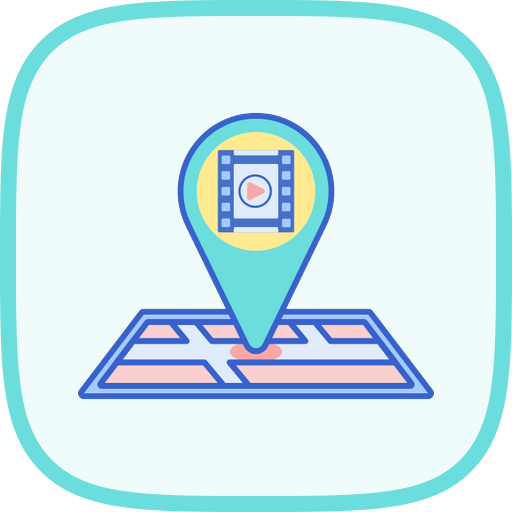
Variables and Memory Addresses
In this tutorial, we will explore Python variables, memory addresses, and the differences between mutable and immutable data types:
Python
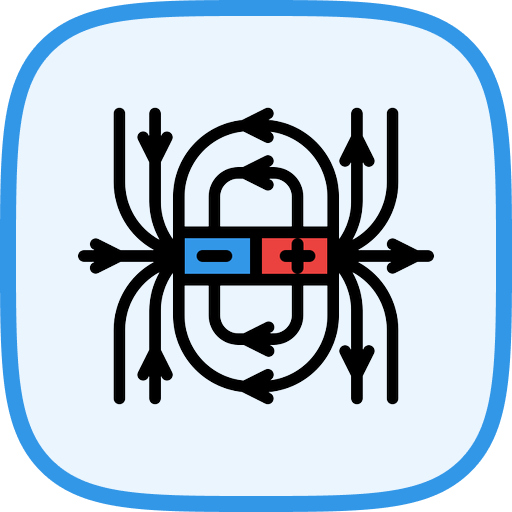
The Power and Flexibility of F-String
In this tutorial, you will learn about Python F-strings, a modern and concise way to format strings in Python. F-strings were introduced in Python 3.6 and have quickly become popular among Python developers for their readability and ease of use.
Python
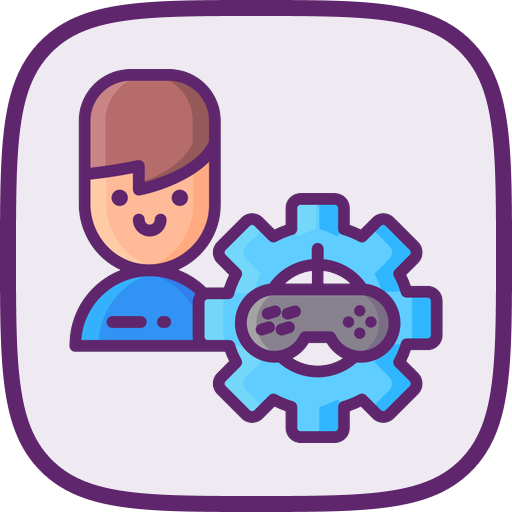
Python Is Operator
The is operator in Python is used to check if two variables refer to the same object in memory. This is different from the == operator, which checks if two variables have the same value. In this lab, we will explore how the is operator works and how it can be useful in Python programming.This is an important concept to understand, especially when working with mutable objects like lists and dictionaries. In this lab, we will explore the usage of the is operator in Python with simple and complex examples, and see how it can be used to write efficient and reliable code.
Python
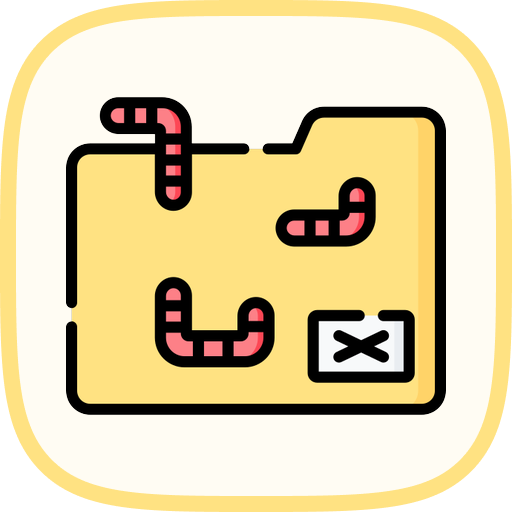
Exploring Python's Collections Module
In this tutorial, we will explore Python's built-in collections module. The collections module is a powerful library that offers a variety of container data types that extend the functionality of Python's built-in containers such as lists, tuples, and dictionaries.
Python
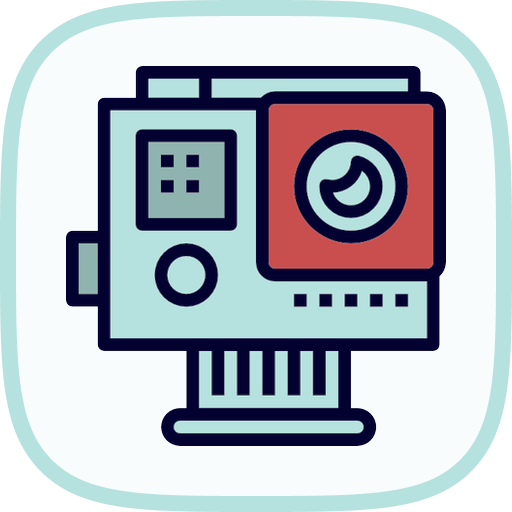
Learn About Managed Generators
Understand Python generators, create a task scheduler with them, test the scheduler, build a network server, and implement an echo server to learn about managed generators.
Python
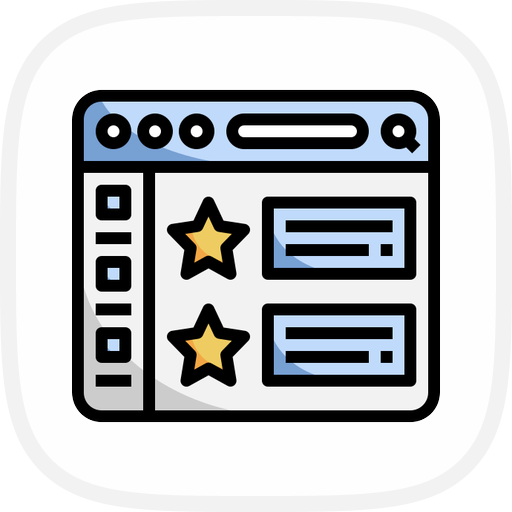
A Review of Module Basics
Create a simple module, import and use modules, understand module loading behavior, use 'from module import' syntax, and explore module reloading limitations to review Python module basics.
Python
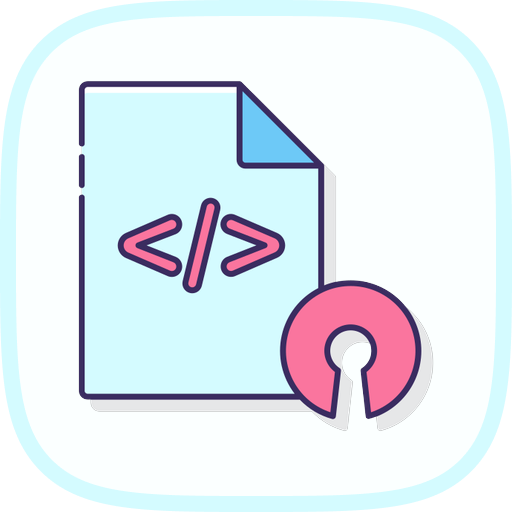
Python Assignment and Reference
In this lab, we'll cover the basics of Python assignment and reference. We'll explore how Python handles assignments, how to create references, and how to work with mutable and immutable objects.
Python
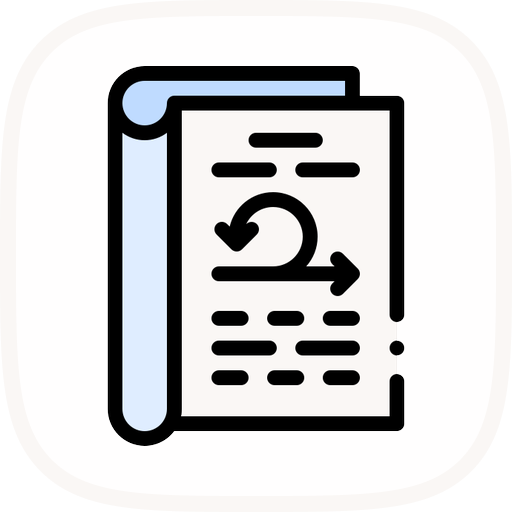
Yield Statement Management in Python
Understand generator lifetime and closure, handle exceptions in generators, and explore practical applications of generator management to master yield statement management in Python.
Python
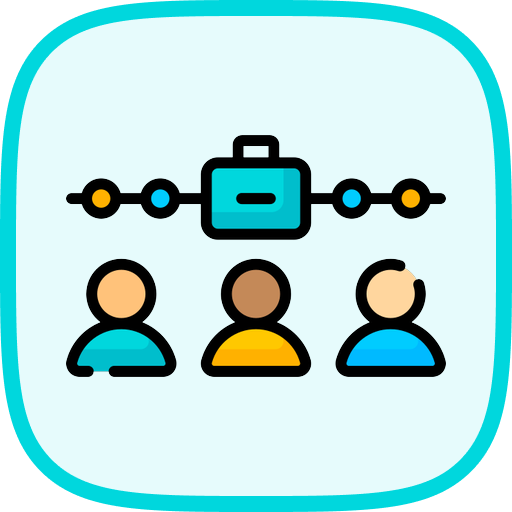
Learn About Delegating Generators
Understand the `yield from` statement, use it in coroutines, wrap sockets with generators, and transition from generators to async/await in Python.
Python
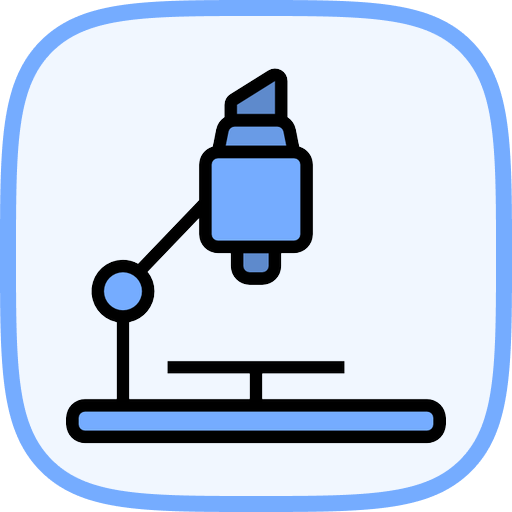
Lab Working with Color Spaces
Welcome to this tutorial on OpenCV-Python Colorspaces!
OpenCV
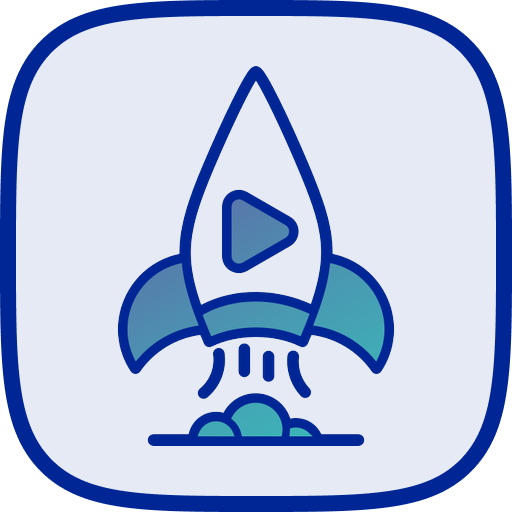
Getting Started with Videos
Welcome to this lab on how to get started with videos using OpenCV-Python! OpenCV (Open Source Computer Vision) is a powerful library designed for image processing, machine learning, and computer vision applications. In this lab, we'll be focusing on how to work with videos in OpenCV-Python.
OpenCV