Introduction
In this project, you will learn how to use JavaScript to implement a simple search functionality. You will learn how to bind the input event to an input box, process the search data, and display the search results.
👀 Preview
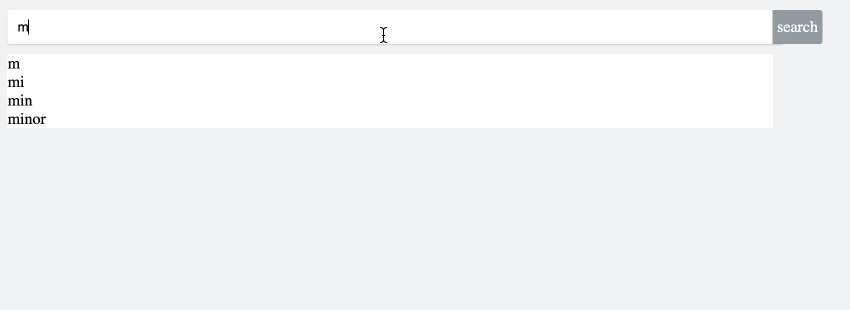
🎯 Tasks
In this project, you will learn:
- How to bind the
input
event to an input box - How to implement the
handleInput
method to filter the search data - How to render the search results in a list
🏆 Achievements
After completing this project, you will be able to:
- Use the
addEventListener
method to bind events - Use the
filter
method to filter an array based on a condition - Manipulate the DOM to update the search results