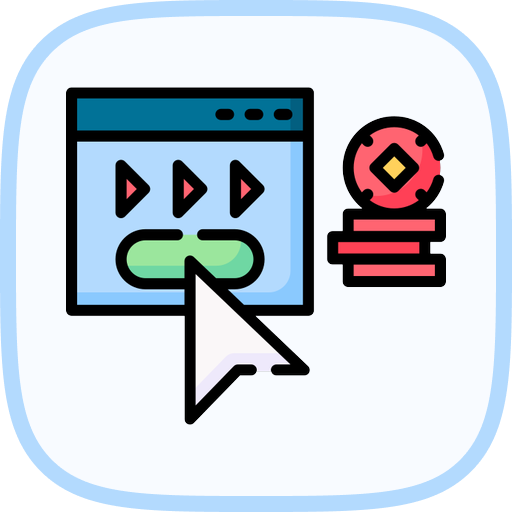
Online JavaScript Playground
LabEx offers an Online JavaScript Playground, a cloud-based environment that allows you to quickly set up a JavaScript development environment for learning and experimentation.
JavaScript
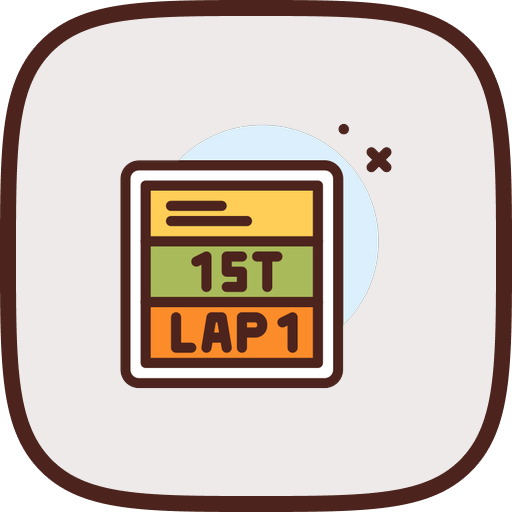
Your First HTML Lab
Hi there, welcome to LabEx! In this first lab, you'll learn the classic 'Hello, World!' program in HTML.
HTML
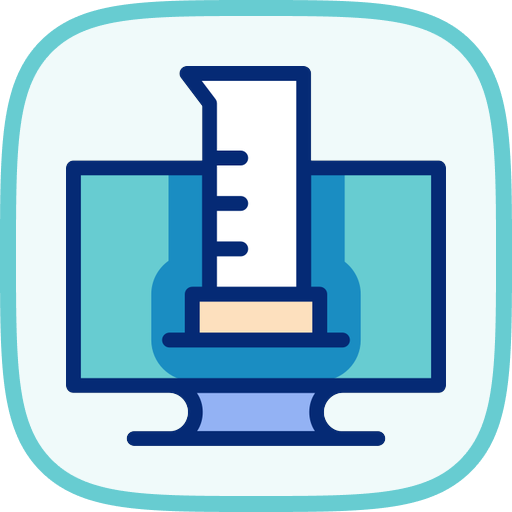
Your First CSS Lab
Hi there, welcome to LabEx! In this first lab, you'll learn the classic 'Hello, World!' program in CSS.
CSS
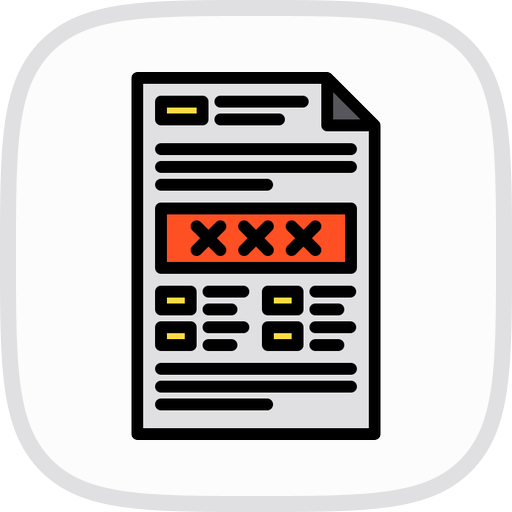
Writing Markup with JSX
Welcome to the React documentation! This lab will give you an introduction to writing markup with JSX.
React
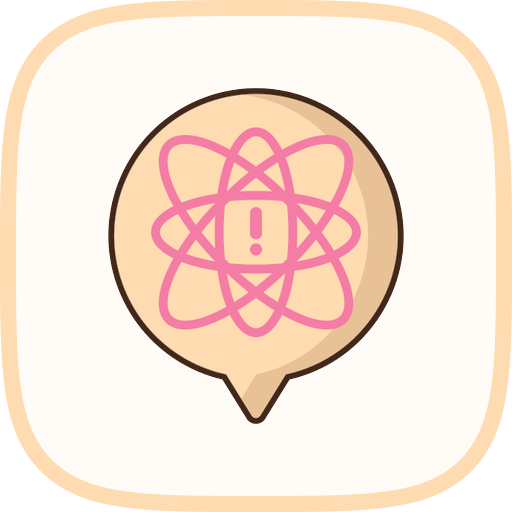
React Hooks Introduction
Welcome to the React documentation! This lab will give you an introduction to using hooks.
React
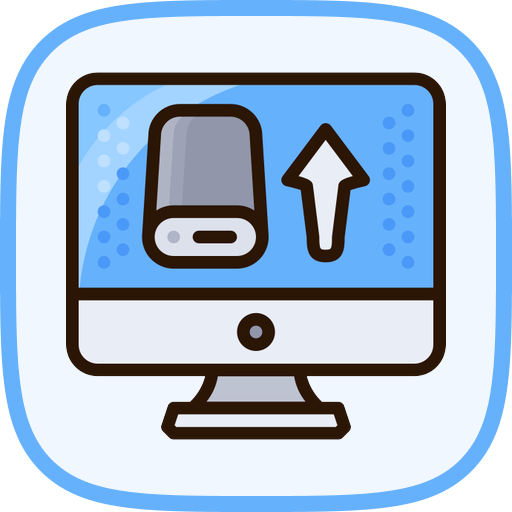
Updating the Screen
Welcome to the React documentation! This lab will give you an introduction to updating the screen.
React
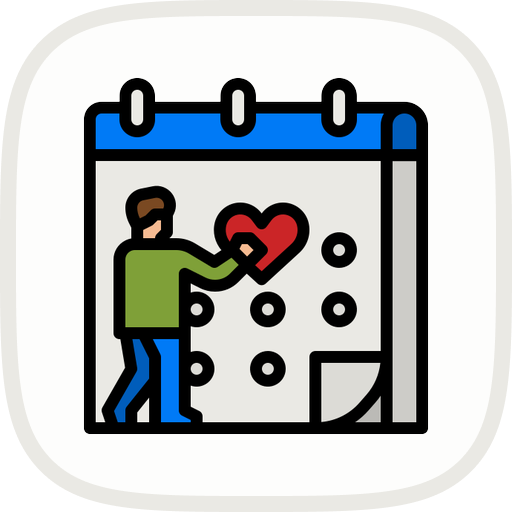
Responding to Events
Welcome to the React documentation! This lab will give you an introduction to responding to events.
React
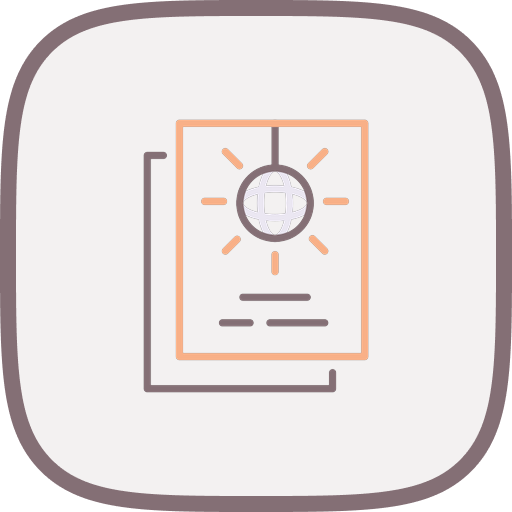
Rendering React Lists Introduction
Welcome to the React documentation! This lab will give you an introduction to rendering lists.
React
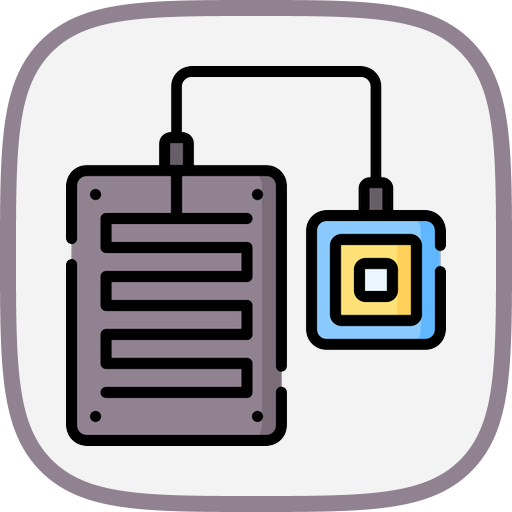
Creating and Nesting Components
Welcome to the React documentation! This lab will give you an introduction to creating and nesting components.
React
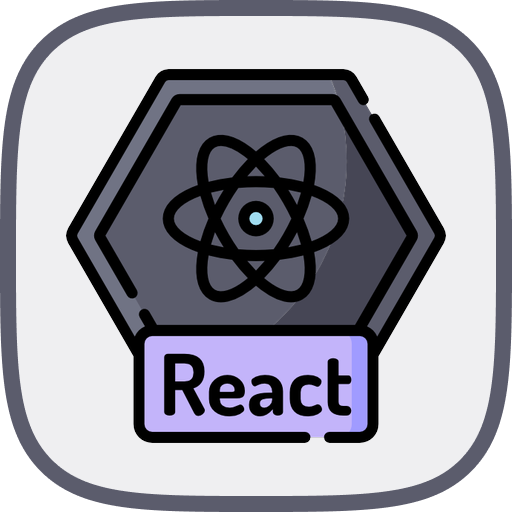
Conditional Rendering in React
Welcome to the React documentation! This lab will give you an introduction to conditional rendering.
React
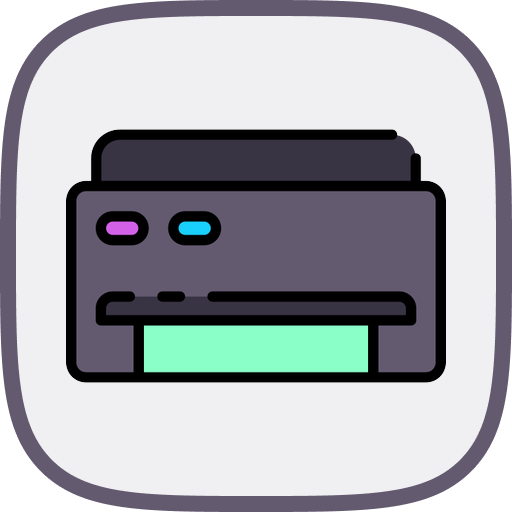
Build a Web Based TCP Port Scanner
In the previous project, we developed a Python port scanner that leveraged threading and sockets to scan TCP ports. While effective, there's room for improvement using third-party packages.
HTMLFlaskPython
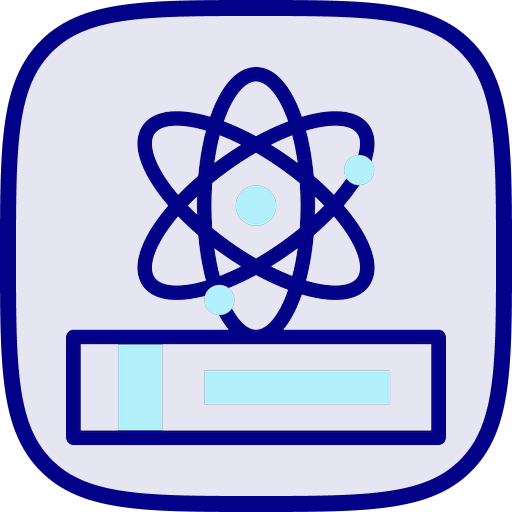
Your First React Lab
Hi there, welcome to LabEx! In this first lab, you'll learn the classic 'Hello, World!' program in React.
React
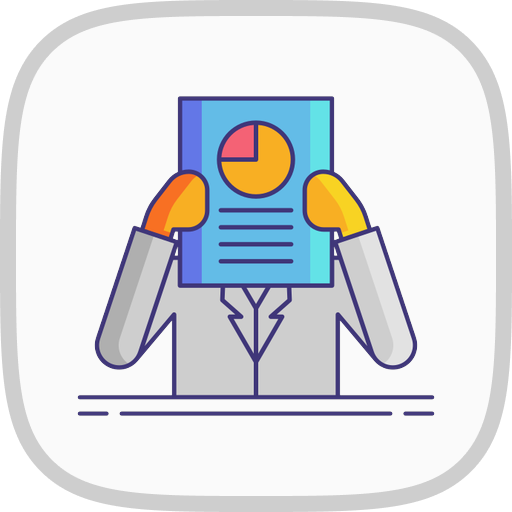
Implementing the Summary
In this lab, set in the bustling startup scene of 'FinTech Valley', you'll step into the shoes of Alex, a budding software engineer tasked with enhancing the financial tracking system for a rapidly growing tech company. The company's financial team needs a dynamic way to view the overall financial health of the organization. Your goal is to implement a feature in their existing web-based accounting application that calculates and displays the total income, total expenses, and net balance, providing real-time financial insights.
JavaScript
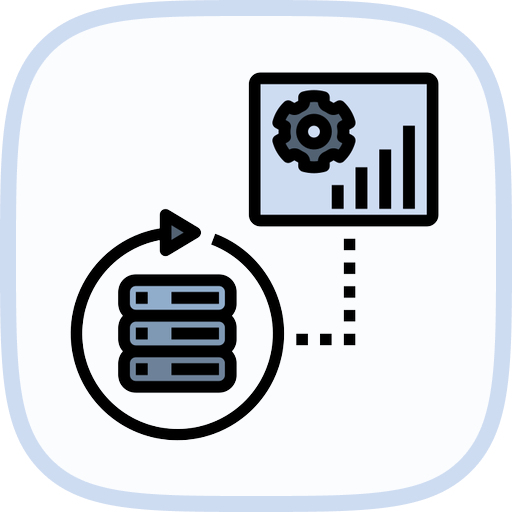
Data Storage and Retrieval
In this Lab, we accompany Alex, a determined web developer at a bustling tech startup, who is now venturing into the realm of data persistence. After successfully creating a mechanism for tracking and displaying financial records in the personal finance tracker, the next challenge is to ensure that these records persist across browser sessions. The goal is to enable users to return to the app and find all their previously entered data intact, enhancing the app's usability and user satisfaction. This task introduces Alex to the concept of web storage, specifically leveraging the localStorage API to save and retrieve the application's state.
JavaScript
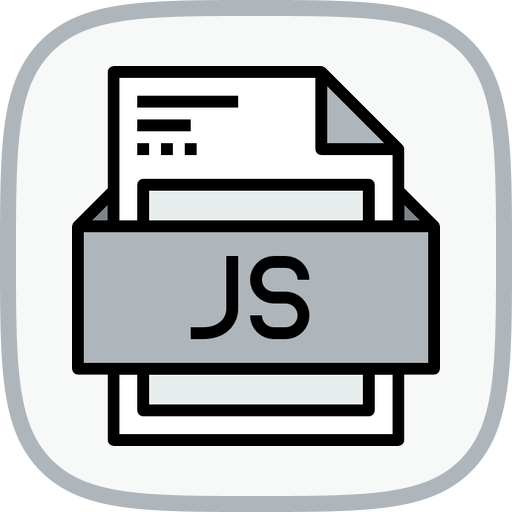
Basic JavaScript and DOM
In this Lab, you'll step into the world of web development through the eyes of Alex, a budding web developer tasked with creating a dynamic personal finance tracker. To build a user-friendly application that allows users to input and track their daily expenses and income. The goal is clear - to develop an interface that's both intuitive and engaging, ensuring users can easily manage their finances without any hassle. This project not only aims to simplify personal finance management but also to introduce you to the fundamental concepts of JavaScript and DOM manipulation.
JavaScript
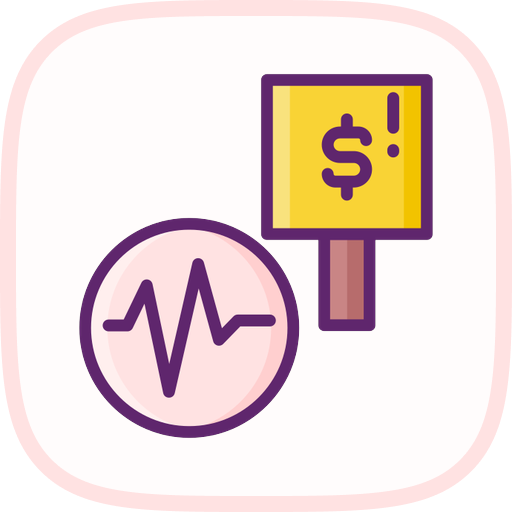
Enhancing Personal Finance Tracker
In this Lab, Alex's journey through the development of the personal finance tracker enters an advanced stage, focusing on enhancing the application's interactivity through sophisticated event handling techniques. The scene is set in a brainstorming session at the tech startup, where Alex proposes adding a drag-and-drop feature to reorder financial records. This ambitious goal aims to provide users with an intuitive way to organize their records, reflecting the fluid nature of personal finance management. Achieving this requires Alex to explore advanced DOM events and manipulate elements in a way that's both visually appealing and user-friendly.
JavaScript
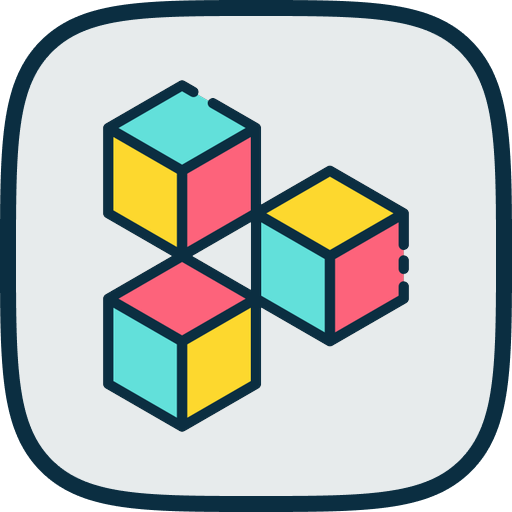
Arrays and Objects
In this Lab, we continue to follow Alex on their journey as a web developer at a tech startup. The scene unfolds in the development team's workspace, where Alex is tasked with enhancing the personal finance tracker by incorporating the ability to handle multiple financial records. This crucial feature requires Alex to delve into JavaScript's arrays and objects, storing each financial record as an object within an array. The goal is to create a dynamic and interactive application that not only tracks but also organizes users' financial data efficiently. Through this endeavor, Alex aims to provide users with a clear overview of their financial activities, thus making the app more useful and engaging.
JavaScript
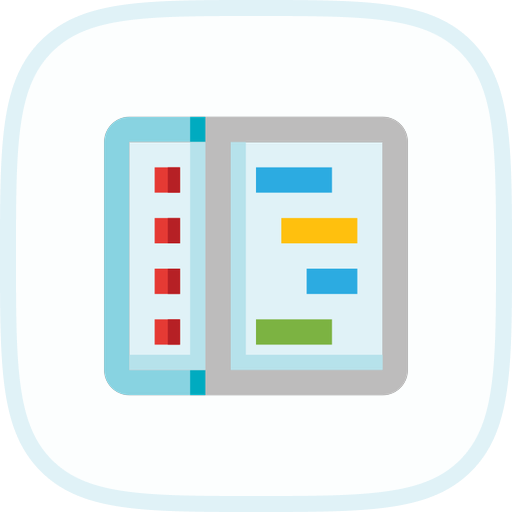
HTML Unordered List
The ul tag in HTML is used to create an unordered list where the items are typically rendered as a bulleted list. This lab will guide you on how to create an unordered list using HTML and provides examples of ul tag syntax and usage.
HTML