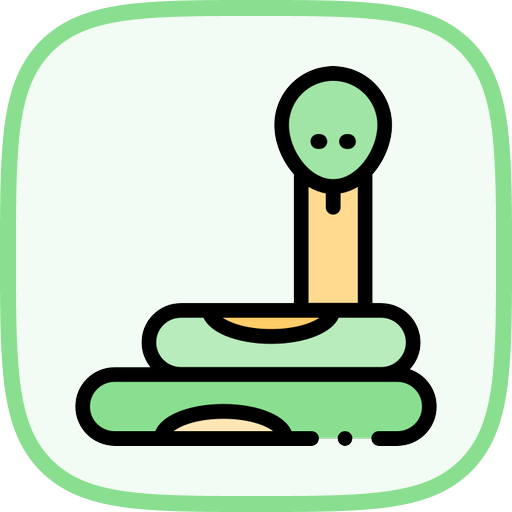
Online Java Playground
Explore, learn, and master Java programming with our cutting-edge Online Java Playground - the ultimate interactive coding environment.
Java
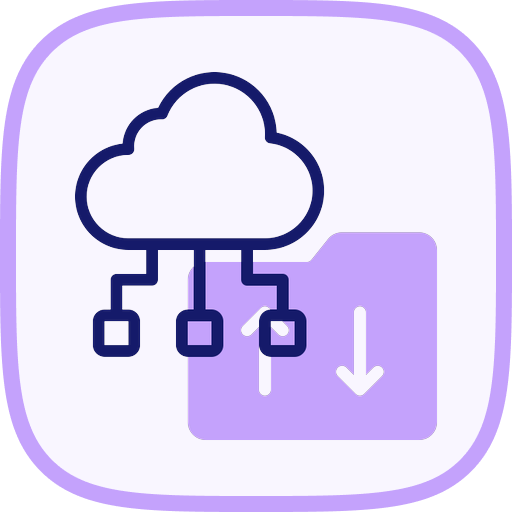
Hadoop Installation and Deployment
This lab is mainly about the foundations of Hadoop and is written for students with a certain Linux foundation to understand the architecture of the Hadoop software system, as well as the basic deployment methods.
HadoopLinux
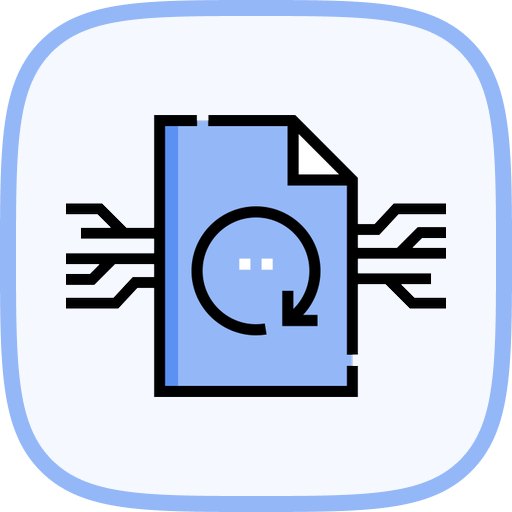
Convert Integer to String in Java
In this lab, you will learn how to use the toString() method of the Java Integer class to convert an integer value to a string in Java programming. This method is extremely useful when you need to display the integer value as a string.
Java
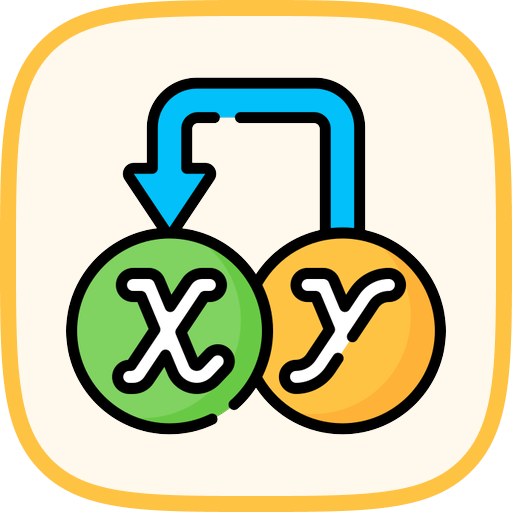
Java Character reverseBytes Method
Java reverseBytes() method is a part of Character class which returns the values obtained by reversing the order of bytes for the specified character. In this lab, we will learn how to use this method step-by-step.
Java
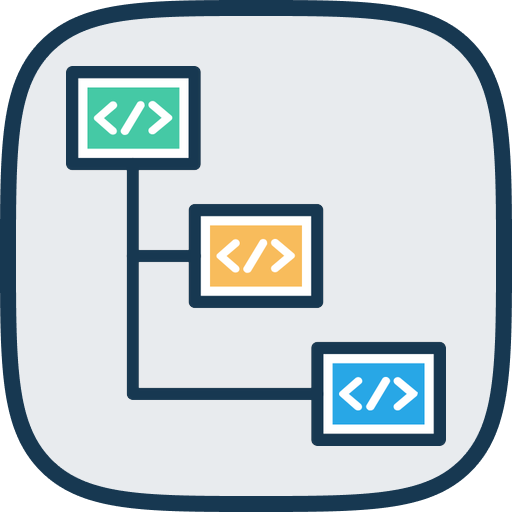
Java Integer Divideunsigned Method
The divideUnsigned() method is one of the methods of the Integer class in Java. The method is used to return the quotient (unsigned) obtained by dividing the first argument (dividend) with the second argument (divisor). This lab provides a step-by-step guide on how to use the divideUnsigned() method in Java.
Java
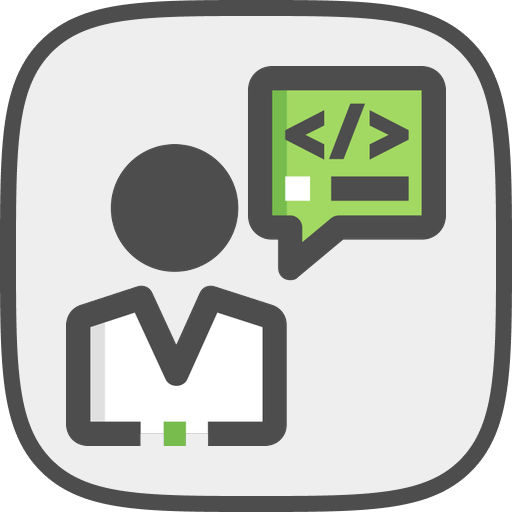
Mastering Java Ternary Operator
In this lab, you will learn how to use the ternary operator in Java to replace if-else statements. The ternary operator is used to write conditional statements in a single line, making the code cleaner and more readable. You will learn how to use the ternary operator and how to nest it.
Java
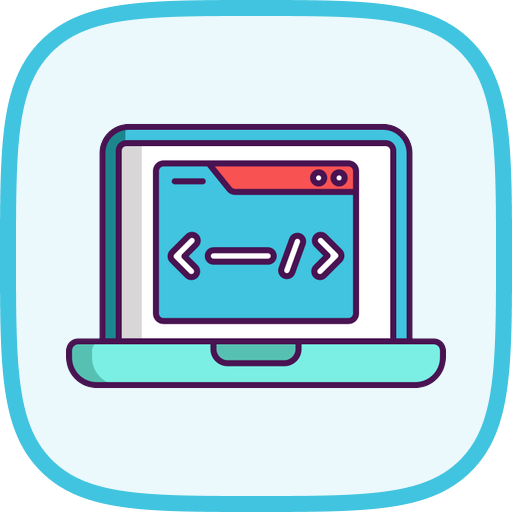
Immutable Strings in Java Programming
This lab will guide you through the benefits of using immutable Strings in Java programming. You will learn why Strings in Java are immutable and how they can increase the overall performance of your application. By the end of this lab, you will grasp the importance of using immutable Strings and know how to create and use them in your program.
Java
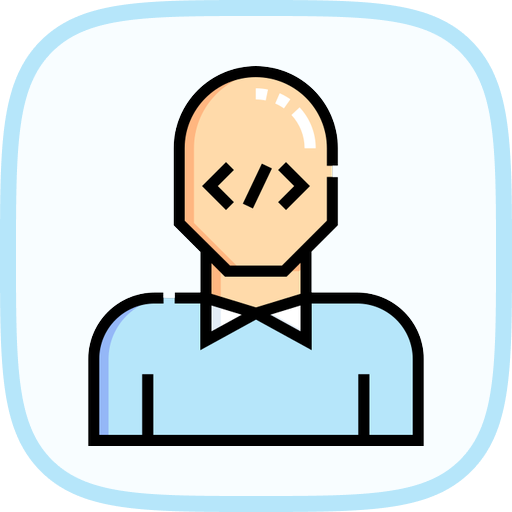
Bitwise XOR Operator in Java
The XOR operator is a bitwise operator used in Java to compare individual bits of the operands. In Java, the XOR operator is represented by the caret symbol (^). It is used to compare two bits and returns true if and only if the two bits being compared are not the same.
Java
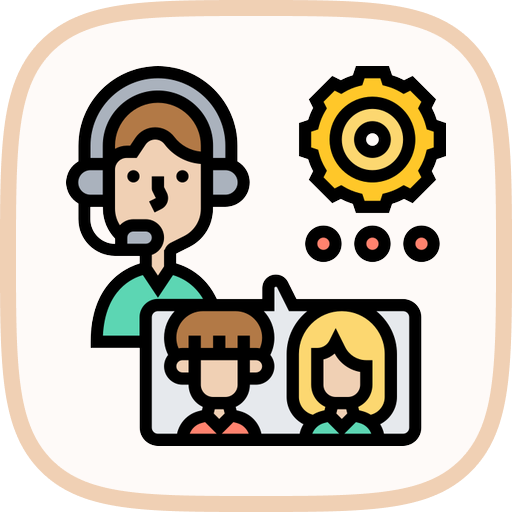
Variables And Operators
In this lab, you'll learn how to declare different types of variables and assignment, several primitive data types, their differences, and different operators and expressions.
Java
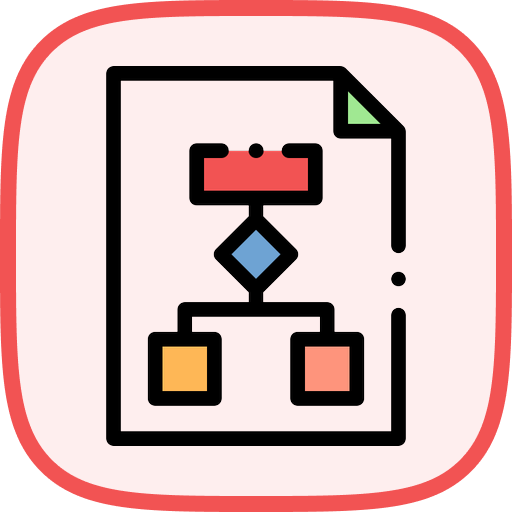
Mastering Java TreeMap Data Structure
The TreeMap class in Java is a part of the Java Collection Interface and implements the Map interface. It stores the key-value pairs in a sorted order unlike other Map implementations. In this lab, you will learn how to use the TreeMap class effectively in your Java programs.
Java
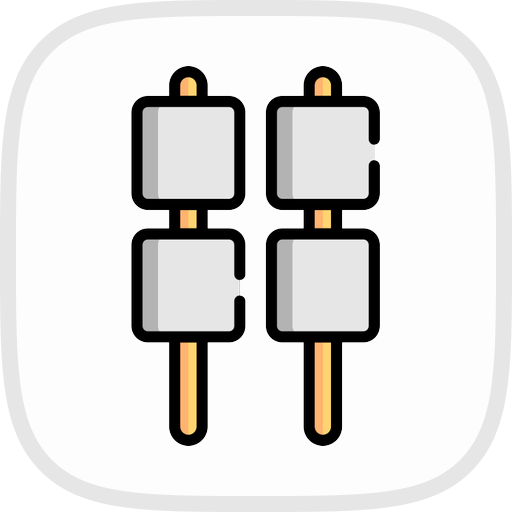
Sorting a HashMap
A HashMap is a collection that stores key-value pairs. However, it does not store the key-value pairs in any particular order, and it does not maintain the insertion order of elements. There may be cases when we want to view the data stored in a sorted manner. In such cases, we need to sort the HashMap.
Java
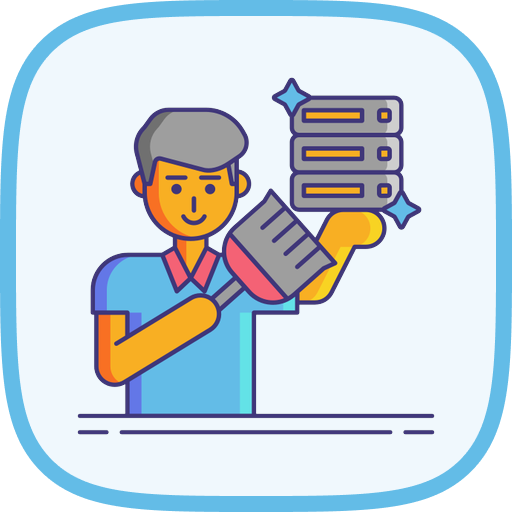
Overloading And Overriding
In this lab, you will learn method overloading and method overriding. Overriding and overloading are two concepts used in Java programming language. Both the concepts allow programmer to provide different implementations for methods under the same name. Overloading happens at compile-time while overriding happens at runtime. Static methods can be overloaded but cannot be overridden. Overloading is a static bond while overriding is dynamic bond.
Java
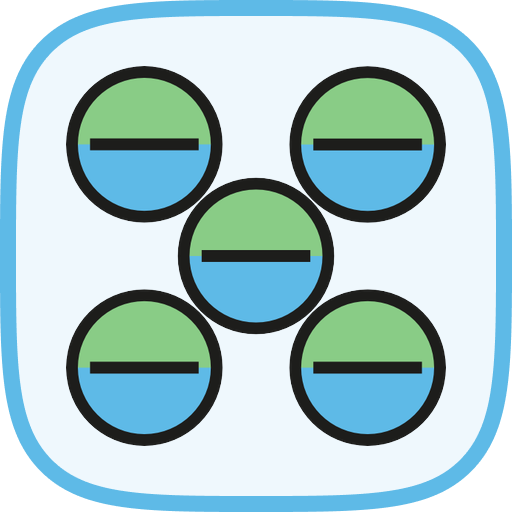
Polymorphism And Encapsulation
Encapsulation is like a bag that encloses the operations and the data related to an object together. Polymorphism is the ability of an object to take on many forms. In this lab, you will see what they are like.
Java
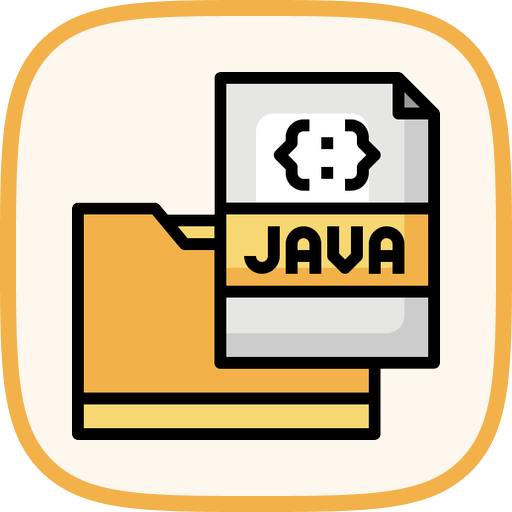
Checking Palindromes in Java
In this lab, we will learn how to check whether a string is a palindrome or not in Java. A palindrome is a string which reads the same forwards and backward.
Java
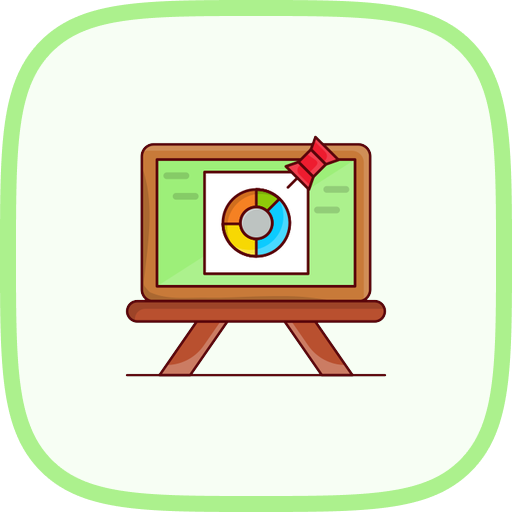
Arranging Classes by Functionality
In this lab, you will learn to use packages to arrange for classes according to their functionalities.
Java
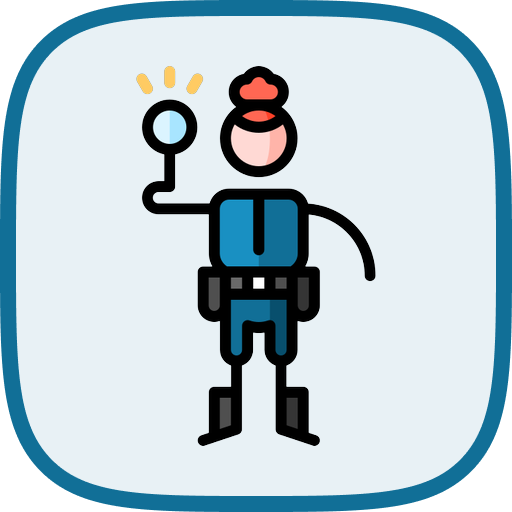
Spy in Mockito
Mockito is a Java framework used for mocking and unit testing Java applications. In this lab, we will learn about spies in Mockito. A Spy is like a partial mock, which will track the interactions with the object like a mock. Additionally, it allows us to call all the normal methods of the object. We will use the Mockito.spy() method and annotations to create spies and demonstrate how to stub a Spy.
Java
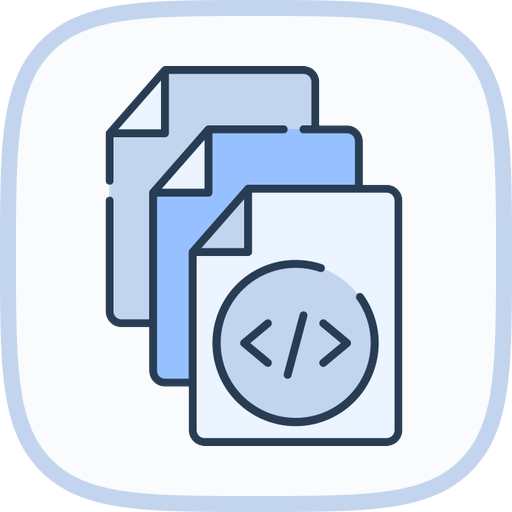
Utilizing Pairs in Java Programming
Pairs are a way of storing paired data, consisting of two fields - one for the key and another for the value. In Java, Pairs are a great way of returning multiple values from a method. There are plenty of ways of using Pairs in Java. This lab will provide step-by-step guidance on how to use pairs in Java with code examples.
Java
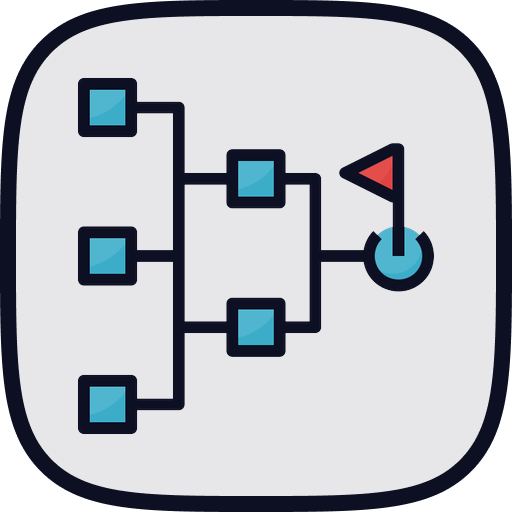
Efficient QuickSort Divide-and-Conquer Algorithm
QuickSort is a Divide-and-Conquer sorting algorithm. It works by selecting a 'pivot' element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then sorted recursively. QuickSort's average case complexity is O(n log n). It should be noted that QuickSort is not a stable sort, that is, the relative positions of equal sort items may not be preserved.
Java