Introduction
In this project, you will learn how to create a new address and manage addresses in a web application. The project involves implementing state-city cascading dropdown, form validation, label styling, and rendering of new addresses.
👀 Preview
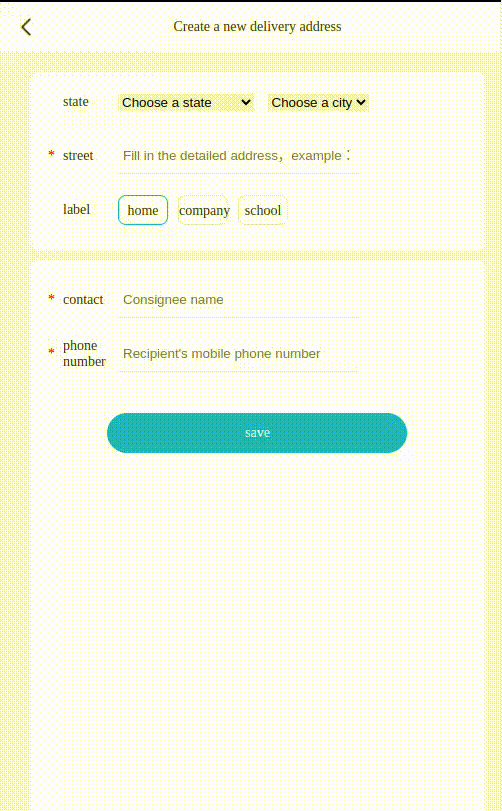
🎯 Tasks
In this project, you will learn:
- How to initialize the province dropdown with data from the
data.js
file - How to implement the state-city cascading dropdown functionality
- How to add a click event to the labels in the tag list, allowing the selected label to be activated and others to be deactivated
- How to validate the address, contact, and phone number fields before saving a new address
- How to create and append a new address list item to the address list
🏆 Achievements
After completing this project, you will be able to:
- Manipulate the DOM using JavaScript
- Handle user interactions and events
- Implement form validation and data handling
- Dynamically create and append HTML elements