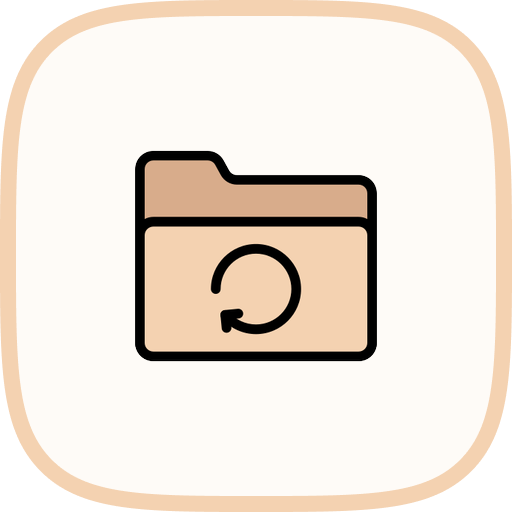
Linux cd Command: Directory Changing
Learn the Linux cd command to efficiently navigate your file system. Learn various techniques for changing directories, understanding paths, and exploring the file structure to enhance your command-line skills.
Linux
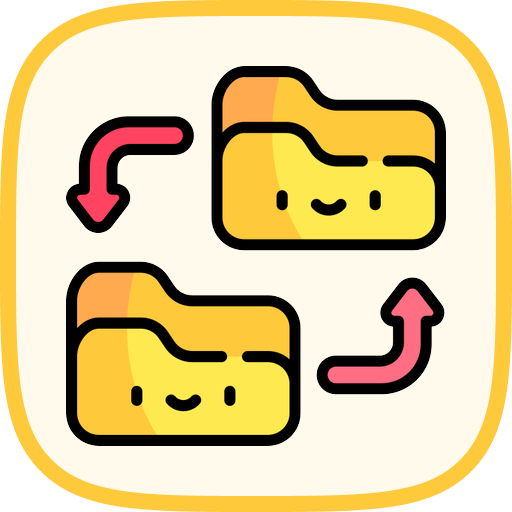
Clone a Repository
Learn how to clone Git repositories using various techniques, including basic cloning, cloning to specific directories, creating shallow clones, and cloning specific branches. This lab will enhance your Git skills and improve your workflow efficiency.
Git
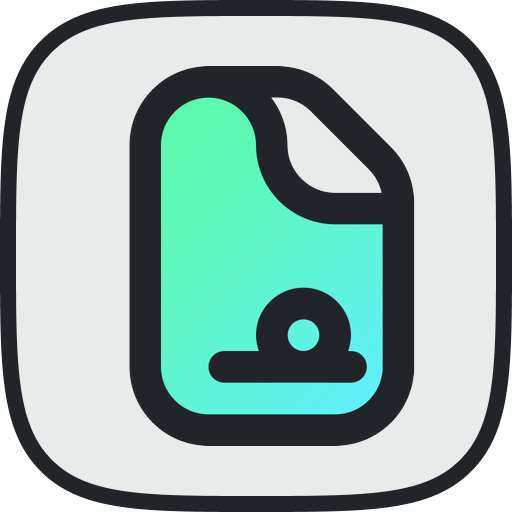
Linux Permission Modifying
In a medieval city nestled amidst rolling hills and thriving markets, word has spread of the arrival of a traveling minstrel — a poet with tales that echo through the halls of time. The minstrel carries with them an ancient tome, filled with songs and stories of old, intended to be shared with all, yet guarded against the night's darkness when only the chosen few gather to hear whispers of wisdom and courage.
Linux
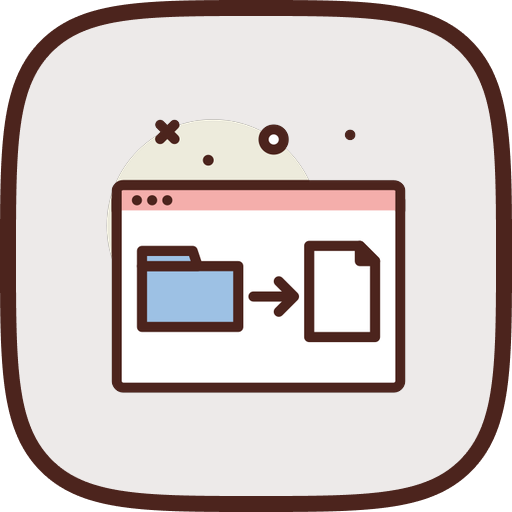
Linux Directory Changing
Welcome to the desolate dunes of the Digital Desert, the virtual landscape where our tale unfolds. Amid this barren expanse lies an oasis of knowledge, a place where the tenacious learner can quench their thirst for Linux command-line mastery.
Linux
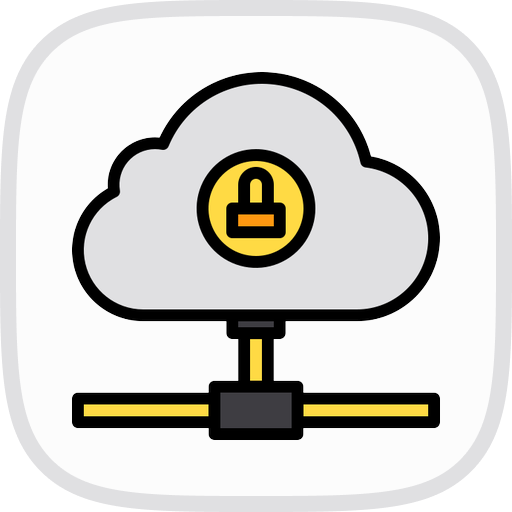
Linux Secure Connecting
Welcome to the Linux Secure Connecting lab. In this lab, you will learn how to use Secure Shell (SSH) to establish secure connections to Linux servers. You will generate SSH keys, understand public key authentication, and practice secure remote connectivity fundamentals.
Linux
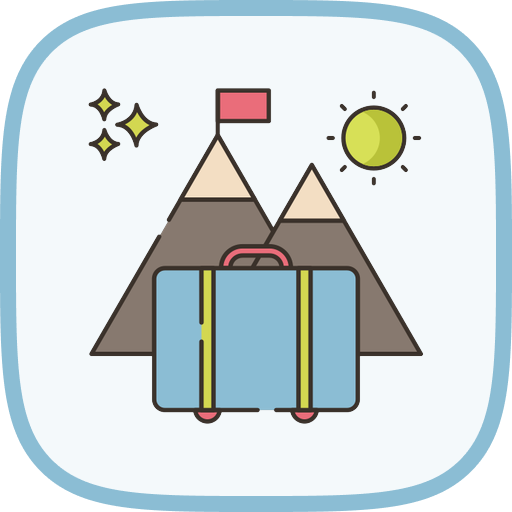
Make a New Primitive Type
Create a basic MutInt class, improve its string representation, add mathematical and comparison operations, and implement type conversions to make a new primitive type in Python.
Python
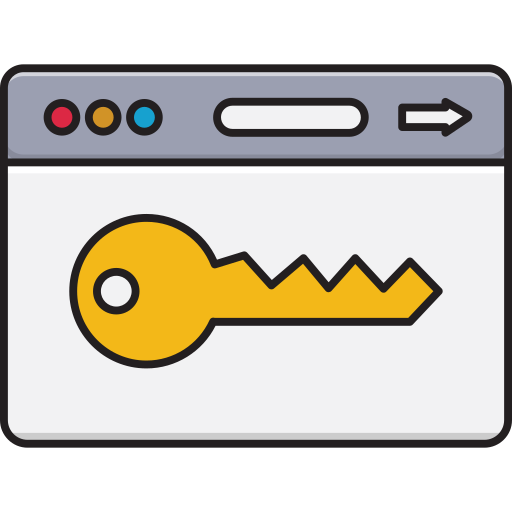
Find Exposed Login Credentials
Learn how to detect and extract exposed login credentials from network traffic using Wireshark, enhancing your network security and packet analysis skills.
Wireshark
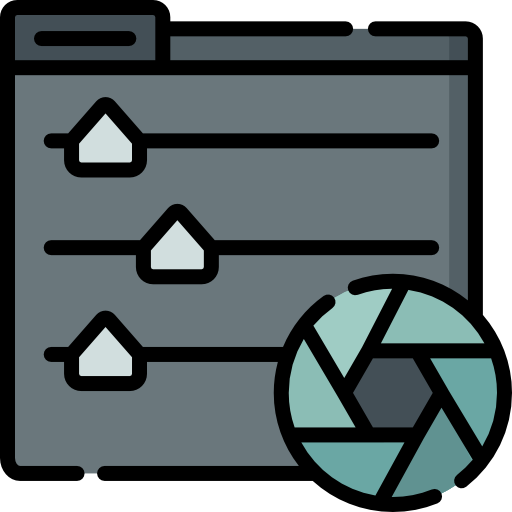
Apply Wireshark Capture Filters for Network Traffic Analysis
Learn to use Wireshark capture filters to selectively capture network traffic. Explore different filter expressions and apply them in real - world scenarios for efficient network troubleshooting and security analysis.
CybersecurityWireshark
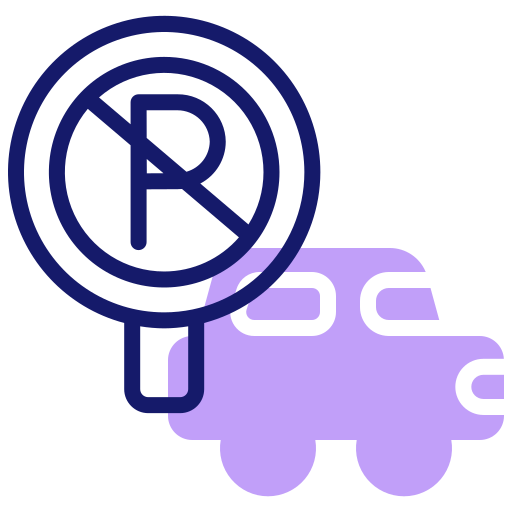
Explore Nmap Verbosity Levels for Network Scanning
Learn to use Nmap at various verbosity levels in this hands - on lab. Set up a local server, understand basics, and see how verbosity impacts scanning results for efficient network analysis.
CybersecurityNmap
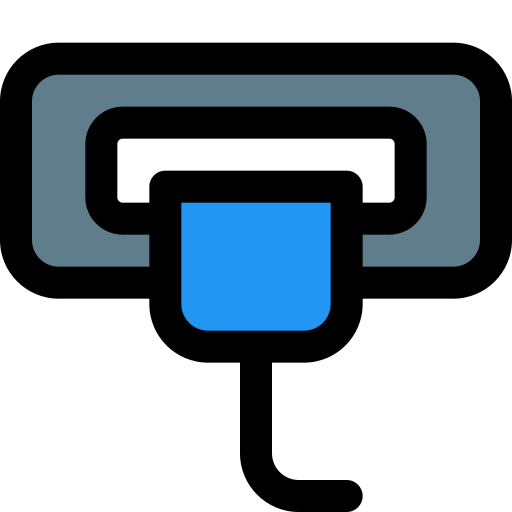
Find Open UDP Port
Discover open UDP ports! This cybersecurity challenge helps you identify vulnerable UDP ports. Learn network scanning techniques and improve your cybersecurity skills. Find open UDP port now!
Nmap
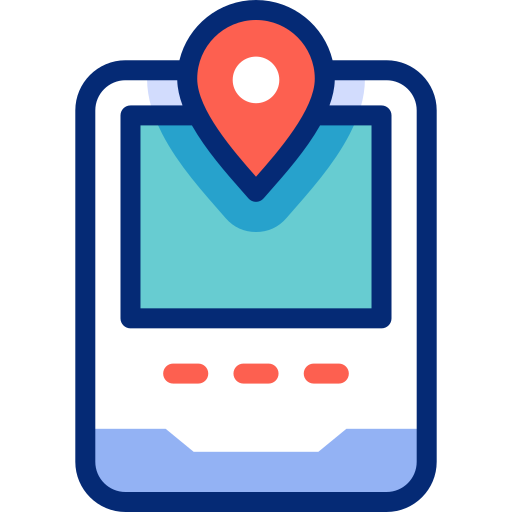
Perform UDP Port Scanning with Nmap
Learn to use Nmap for UDP port scanning in this hands - on lab. Set up a UDP server, perform basic scans, and interpret results, essential for network security assessment.
CybersecurityNmap
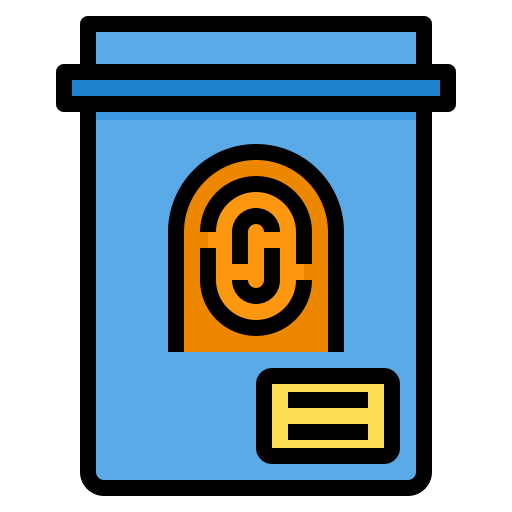
Uncover The Secret Port
Uncover the secret port in this cybersecurity challenge! Test your skills and learn about network security, port scanning, and vulnerability assessment. Perfect for cybersecurity enthusiasts and professionals.
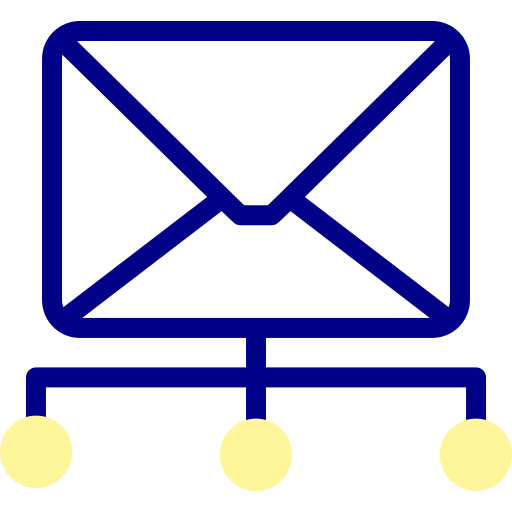
Filter DNS Communications
Learn how to filter DNS communications using Wireshark for effective network traffic analysis and troubleshooting in this hands-on lab exercise.
Wireshark
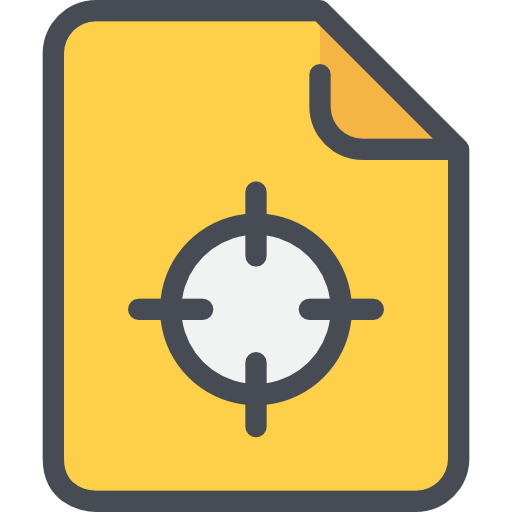
Scan Target From File
Perform cybersecurity scans on targets from a file. Learn to automate vulnerability scanning and target identification for improved security posture. Enhance your cybersecurity skills now!
Nmap
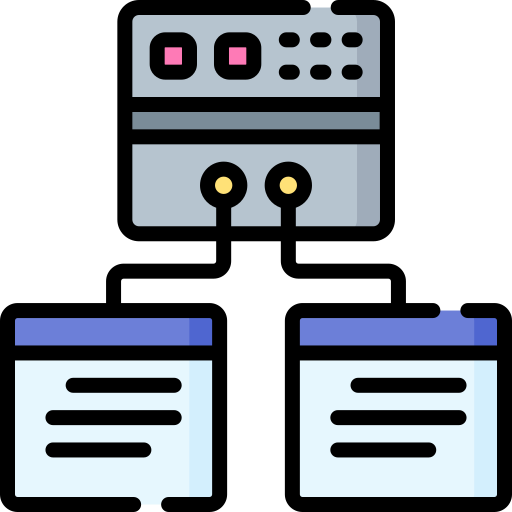
Identify Linux Server Version
Identify Linux server version using command-line tools. Learn Linux server version detection, cybersecurity skills, and system information gathering techniques.
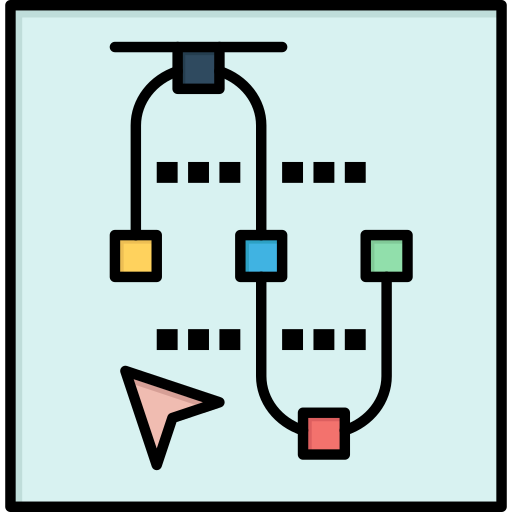
Learn Nmap OS and Version Detection Techniques
Learn the fundamentals of network reconnaissance using Nmap, focusing on OS and version detection. Set up a test environment, conduct scans, and perform comprehensive network analysis.
CybersecurityNmap
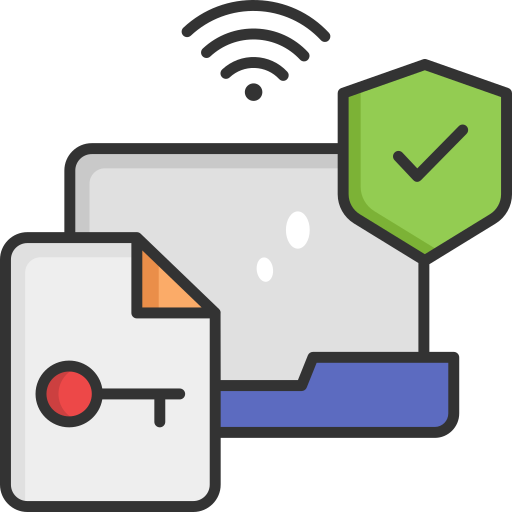
Conduct Nmap SYN Scans for Network Security
Learn Nmap SYN scanning, a stealthy port reconnaissance technique. Create target services, perform SYN scans, and analyze results to assess network vulnerabilities and enhance defenses.
CybersecurityNmap
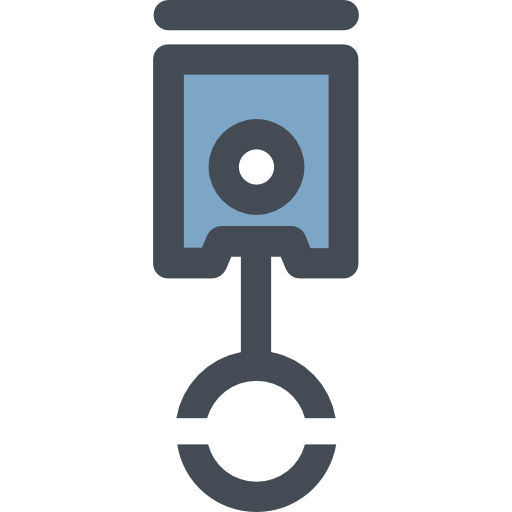
Analyze IPv6 Traffic with Wireshark
Learn to use Wireshark to capture and analyze IPv6 network traffic. Gain practical experience in monitoring and troubleshooting IPv6 networks, applicable in real - world scenarios.
Wireshark