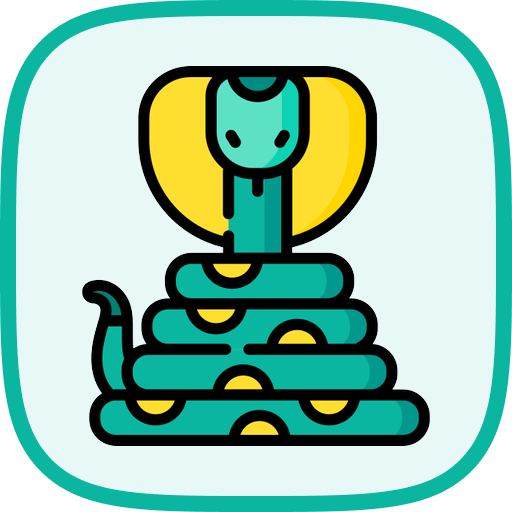
Online Python Playground
Discover the ultimate online Python playground, where you can effortlessly write, test, and execute code in a user-friendly environment.
Python
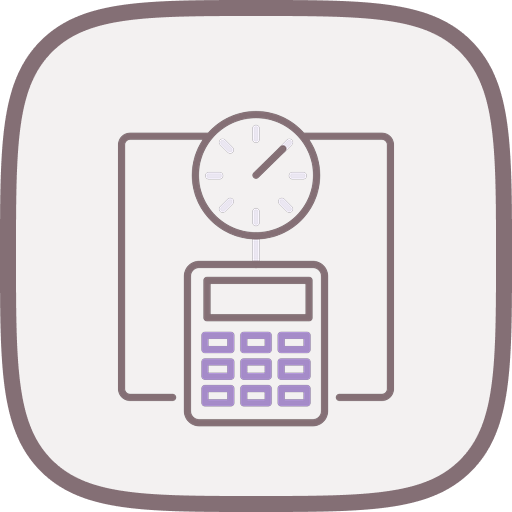
Weighted Average Calculation Function
A weighted average is a type of average that takes into account the importance, or weight, of each value in a set of numbers. In this challenge, you will create a function that calculates the weighted average of a list of numbers.
Python
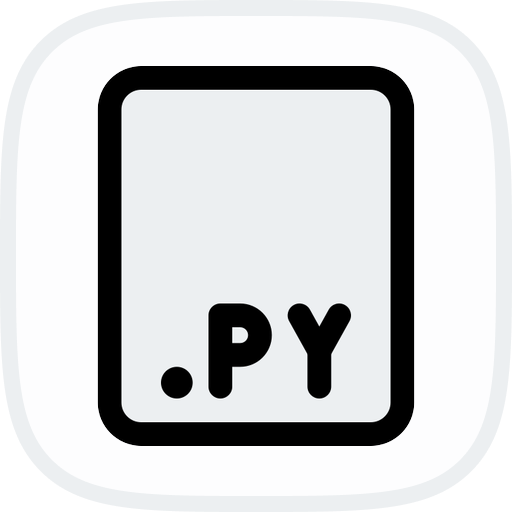
Matrix Transpose in Python
In linear algebra, the transpose of a matrix is an operator which flips a matrix over its diagonal. The transpose of a matrix is obtained by exchanging its rows into columns. In Python, we can transpose a two-dimensional list using a simple one-liner code.
Python
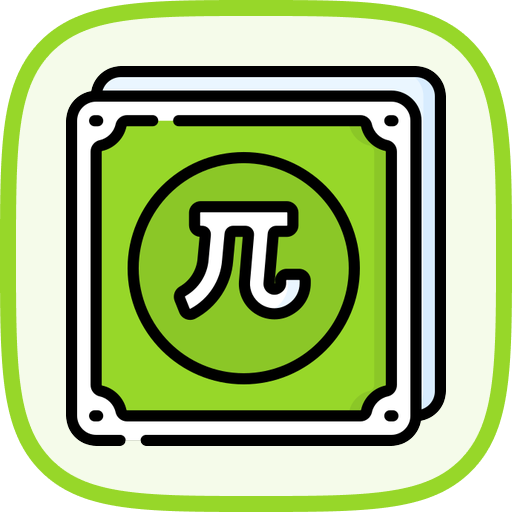
Calculate Month Difference in Python
In Python, you can calculate the difference between two dates using the datetime module. This challenge will test your ability to calculate the month difference between two dates.
Python
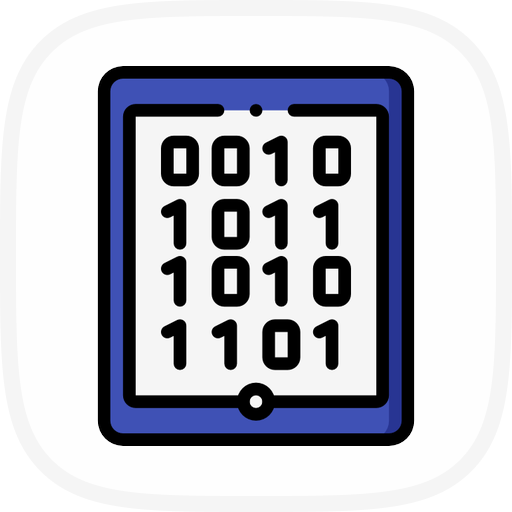
Number to Binary
In computer science, binary is a base-2 numbering system that represents numeric values using two symbols, typically 0 and 1. Binary is widely used in computing and digital electronics. In this challenge, you will write a Python function that takes a decimal number as input and returns its binary representation.
Python
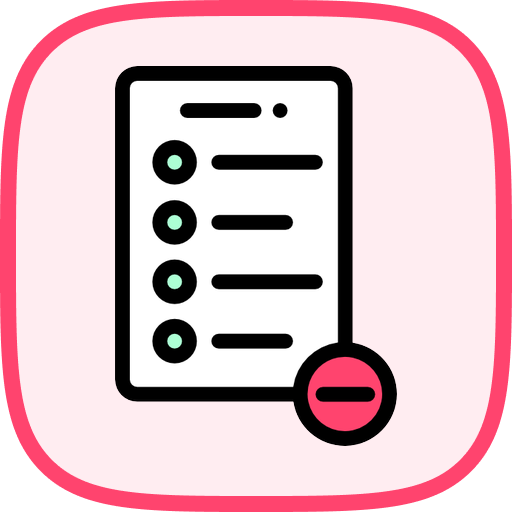
Remove List Elements from the End
In Python, you can remove elements from a list using various methods. One such method is to remove elements from the end of the list. In this challenge, you will write a function that removes n elements from the end of a list.
Python
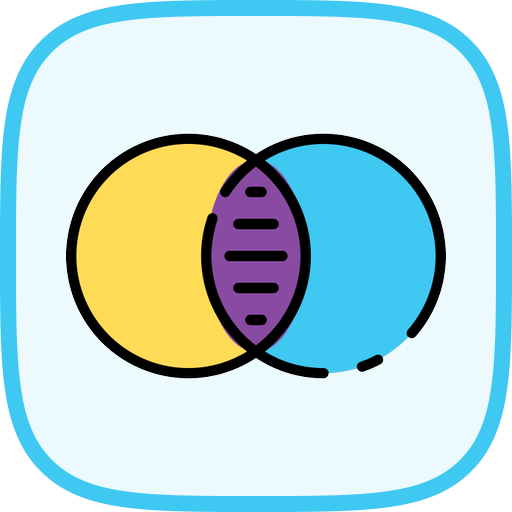
Symmetric Difference Based on Function
In Python, the symmetric difference between two lists is a new list containing all elements that are in either of the original lists, but not in both. In this challenge, we will write a function that returns the symmetric difference between two lists, after applying the provided function to each list element of both.
Python
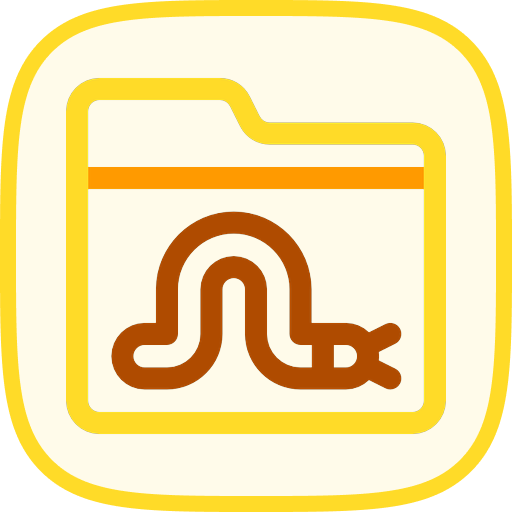
Find Minimum by Attribute in Python
In Python, you can use the min() function to find the minimum value of a list. However, what if you want to find the minimum value of a list based on a specific property or attribute of each element in the list? This is where the min_by() function comes in handy.
Python
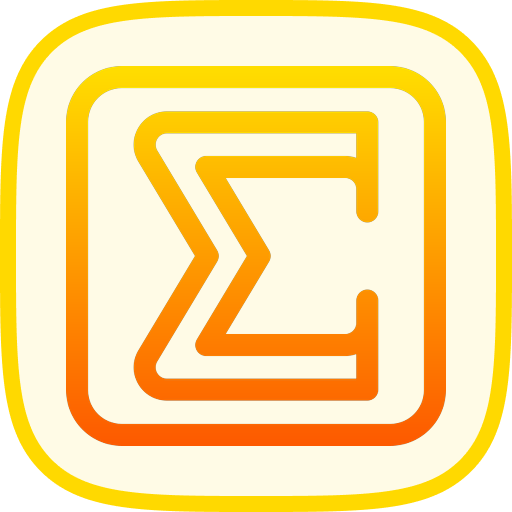
Median Calculation with Python
In statistics, the median is a measure of central tendency that represents the middle value of a dataset. It is the value separating the higher half from the lower half of a data sample. In this challenge, you will be required to write a Python function that finds the median of a list of numbers.
Python
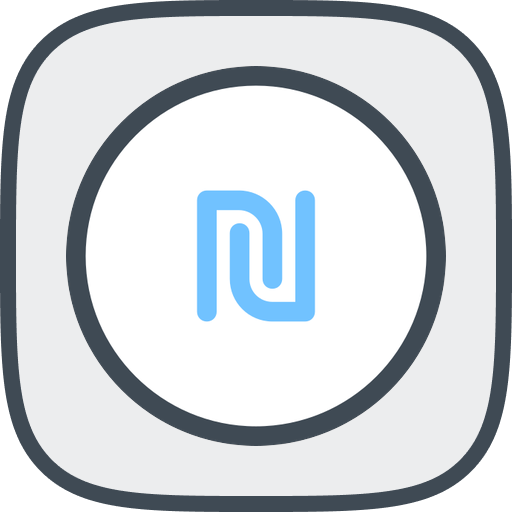
N Minimum Elements
In this challenge, you are tasked with writing a Python function that returns the n minimum elements from a given list.
Python
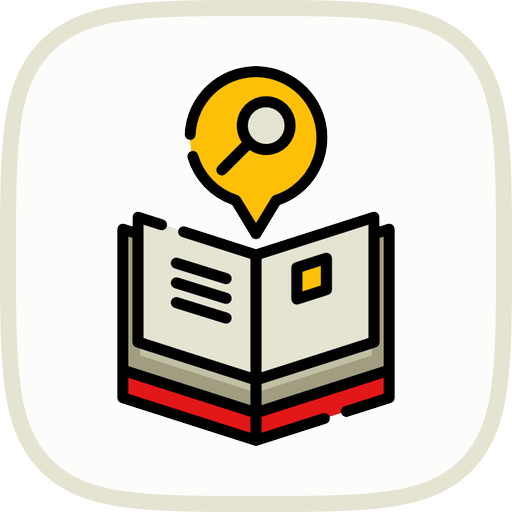
Map List to Dictionary
In Python, a dictionary is a collection of key-value pairs. Sometimes, we need to create a dictionary from a list where the keys are the elements of the list and the values are the result of applying a function to those elements. In this challenge, you will create a function that maps the values of a list to a dictionary using a function.
Python
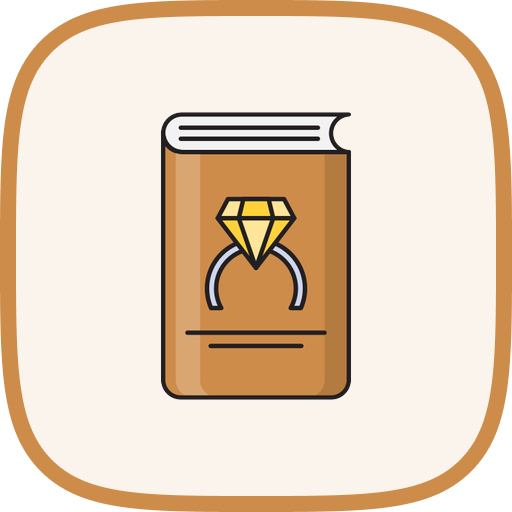
Map Dictionary Values
In Python, a dictionary is a collection of key-value pairs. Sometimes, we need to transform the values of a dictionary using a function. This challenge will test your ability to create a function that takes a dictionary and a function as arguments and returns a new dictionary with the same keys as the original dictionary and values generated by running the provided function for each value.
Python
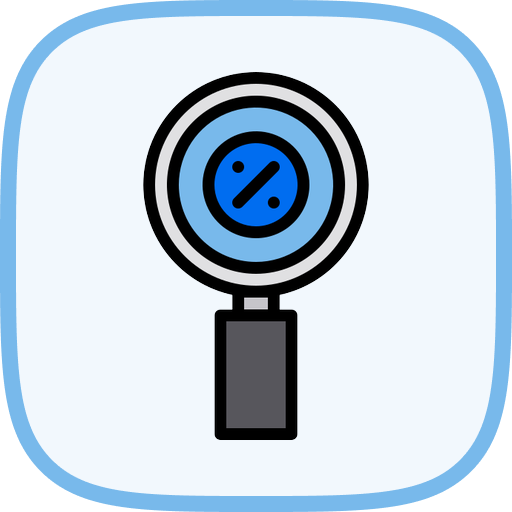
Efficient Prime Number Detection
In mathematics, a prime number is a natural number greater than 1 that is not a product of two smaller natural numbers. For example, 5 is prime because the only ways of writing it as a product, 1 × 5 or 5 × 1, involve 5 itself. However, 4 is not prime because it is a product (2 × 2) in which both numbers are smaller than 4. In this challenge, you need to write a Python function to check if a given number is prime or not.
Python
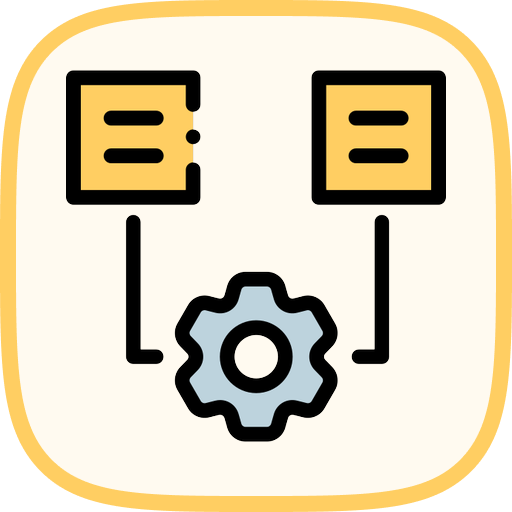
Execute Function for Each List Element
In Python, it is common to need to execute a function for each element in a list. This can be done using a for loop, but it can be tedious to write out the loop every time. In this challenge, you will create a function that takes a list and a function as arguments and executes the function for each element in the list.
Python
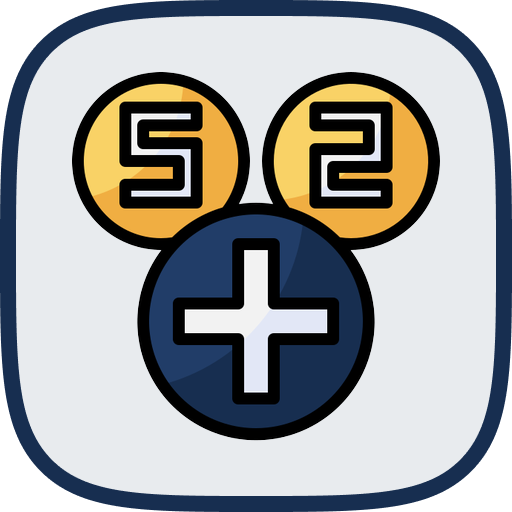
Calculating Greatest Common Divisor
In mathematics, the greatest common divisor (GCD) of two or more integers, is the largest positive integer that divides each of the integers without a remainder. For example, the GCD of 8 and 12 is 4.
Python
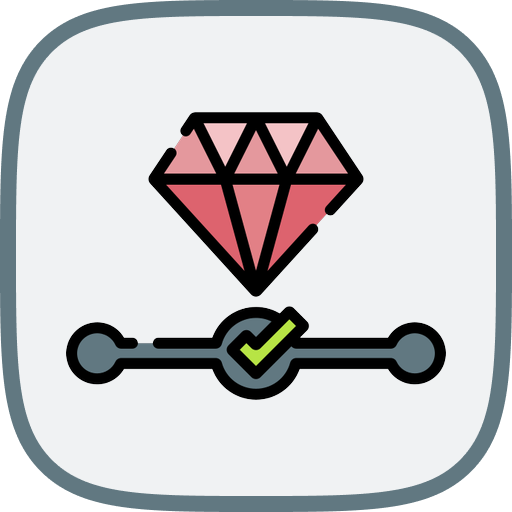
Key of Max Value
In Python, dictionaries are a useful data structure that allows you to store key-value pairs. Sometimes, you may need to find the key of the maximum value in a dictionary. In this challenge, you will write a function that takes a dictionary as an argument and returns the key of the maximum value in the dictionary.
Python
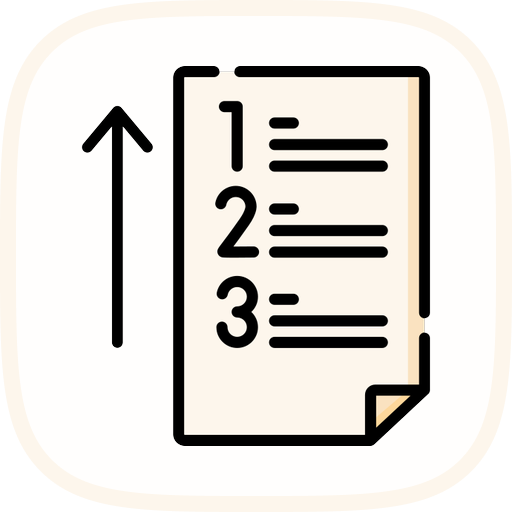
Initialize 2D List
In Python, a 2D list is a list of lists. It is a useful data structure for representing grids, tables, and matrices. Initializing a 2D list involves creating a list of lists with a given width and height and initializing each element with a default value.
Python
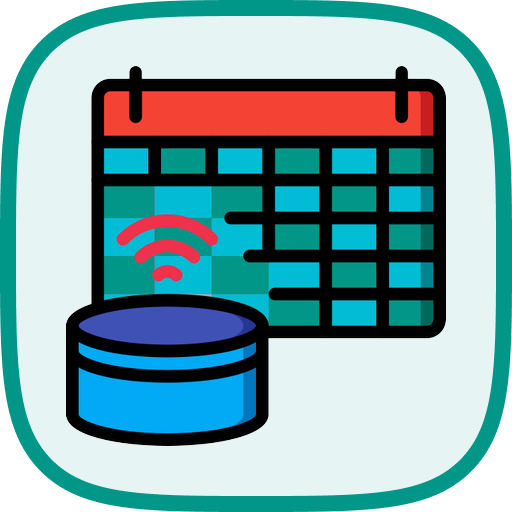
Generate Date Range in Python
In Python, the datetime module provides classes for working with dates and times. One common task is to create a list of dates between two given dates. In this challenge, you will create a function that takes two dates as input and returns a list of all the dates between them.
Python