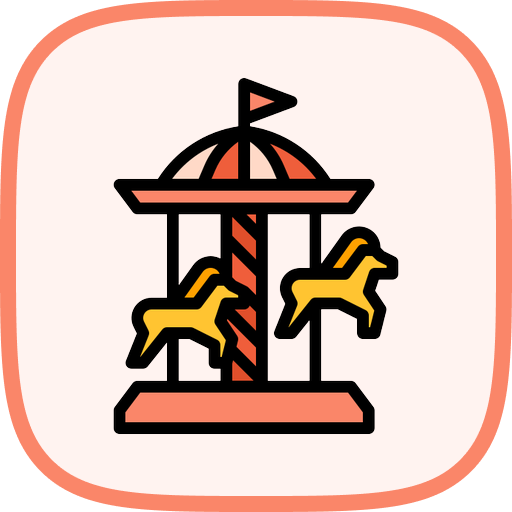
Online Go Playground
Explore the power of Go programming with Online Go Playground - a seamless coding environment for developers to test, debug, and optimize their Go applications.
Go
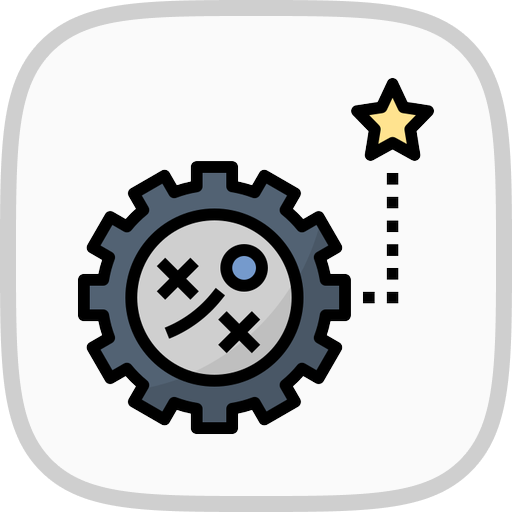
Implementing Rate Limiting in Go
The lab demonstrates how to implement rate limiting in Go using goroutines, channels, and tickers.
Go
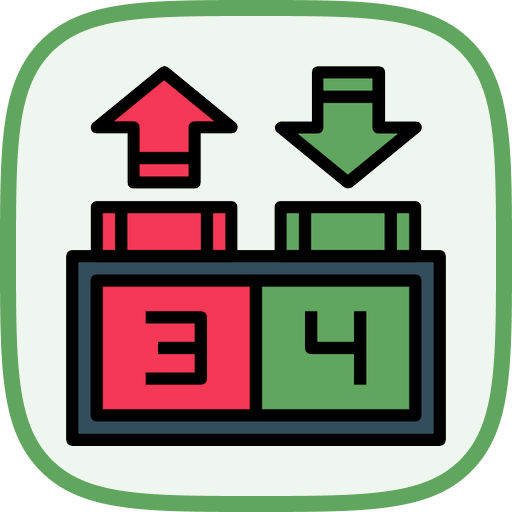
Sorting Built-in Types in Go
The Go programming language provides a built-in package named sort that implements sorting for builtins and user-defined types. In this lab, we will focus on sorting for builtins.
Go
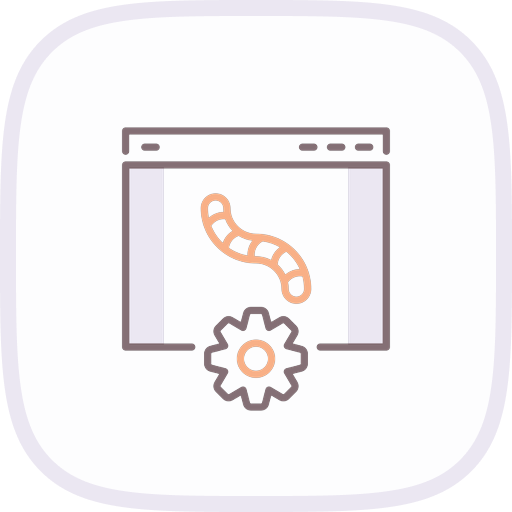
Golang HTTP Request Handling
This lab aims to test your ability to use the net/http package in Golang to issue HTTP requests.
Go
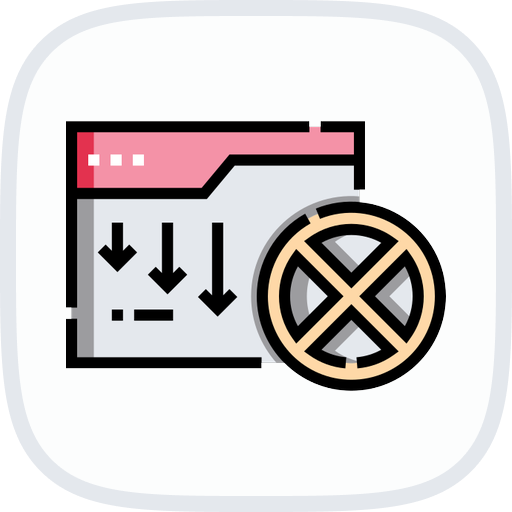
Golang Context Cancellation Demonstration
This lab aims to demonstrate the usage of context.Context for controlling cancellation in Golang. A Context carries deadlines, cancellation signals, and other request-scoped values across API boundaries and goroutines.
Go
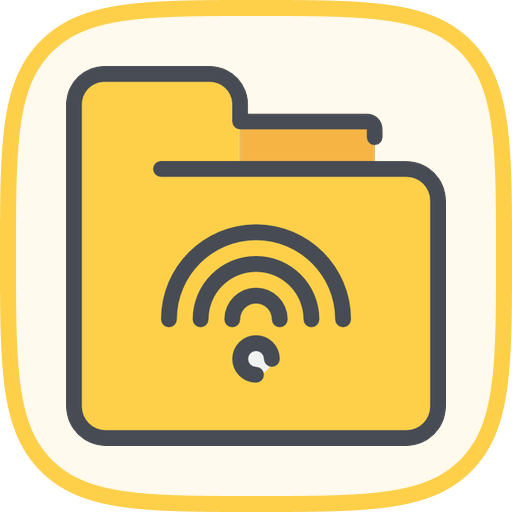
Concurrent Data Access with Mutexes
This lab aims to demonstrate how to use mutexes to safely access data across multiple goroutines.
Go
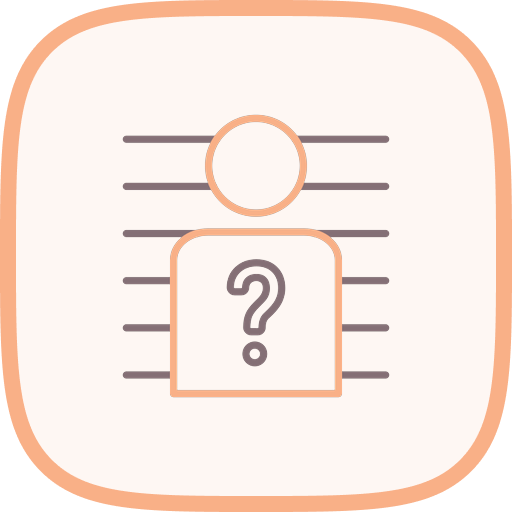
Creating Closures with Anonymous Functions in Go
In this lab, you will learn how to use anonymous functions to create closures in Golang.
Go
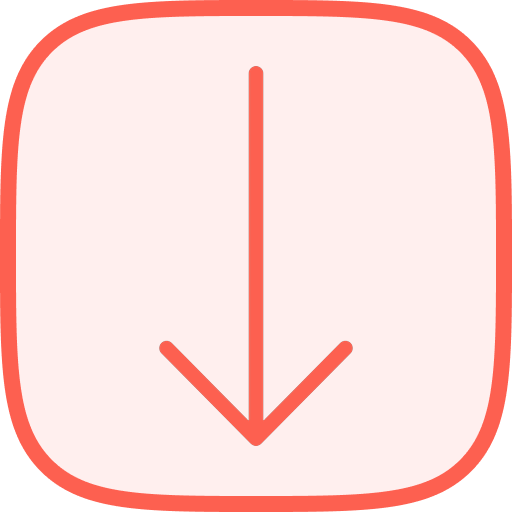
Command-Line Flag Parsing in Go
The purpose of this lab is to implement a command-line program that supports basic command-line flag parsing using the flag package in Golang.
Go
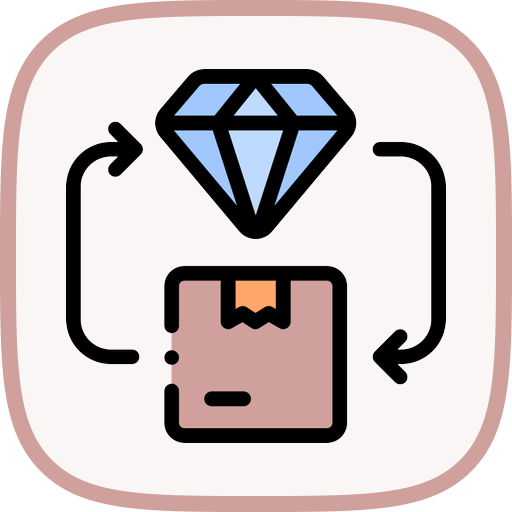
Multiple Return Values
In Go, functions can return multiple values. This feature is commonly used to return both a result and an error value from a function.
Go
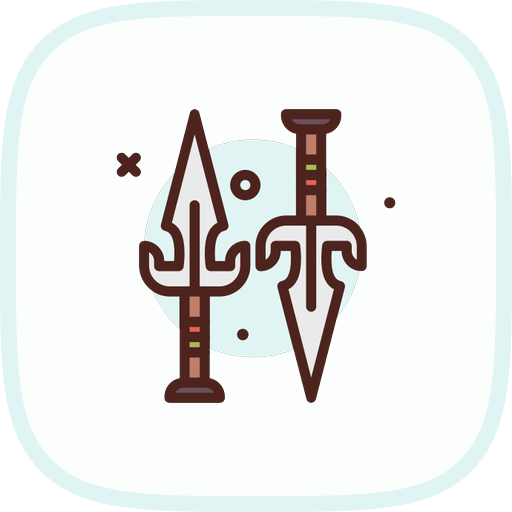
Strings and Runes
This lab focuses on Go strings and runes. In Go, strings are read-only slices of bytes, and runes are integers that represent Unicode code points. This lab will explore how to work with strings and runes in Go.
Go
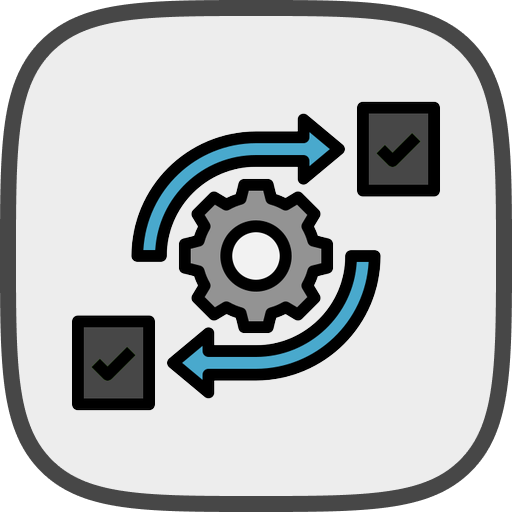
Variadic Functions in Go
In Go, a function that can take a variable number of arguments is called a variadic function. This lab will test your understanding of how to use variadic functions in Go.
Go
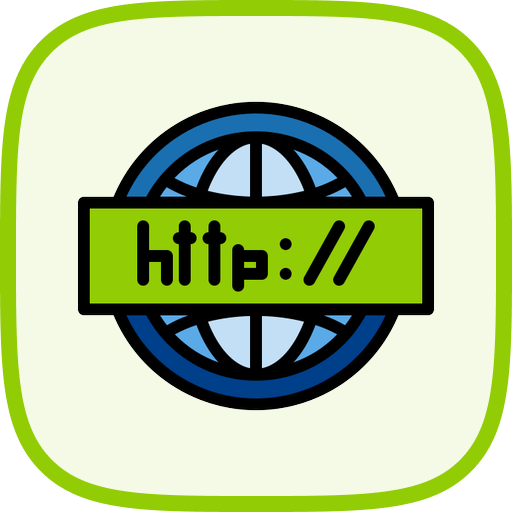
Basic HTTP Server in Go
This lab aims to test your ability to write a basic HTTP server using the net/http package in Golang.
Go
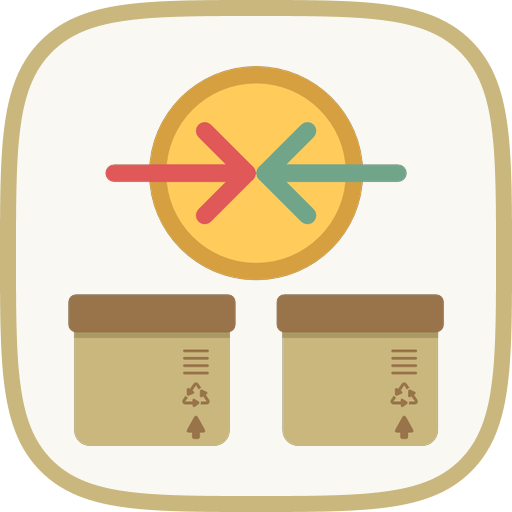
Concurrency Primitives in Go
One of the most attractive features of the Go language is its native support for concurrent programming. It has high support for parallelism, concurrent programming, and network communication, allowing for more efficient use of multi-core processors.
Go
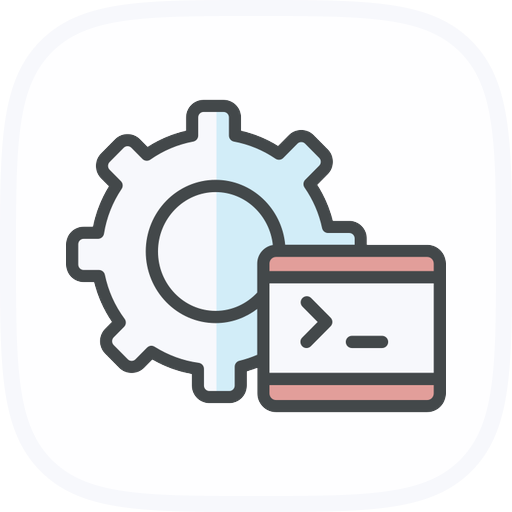
Modular Functions in Programming
In the previous programs, we only used one main function. However, as the program becomes more complex, we can split it into several modular functions. By abstracting the operations with the same functionality into a function, we can simply call the function when needed. It also improves collaboration among multiple people and enhances code readability.
Go
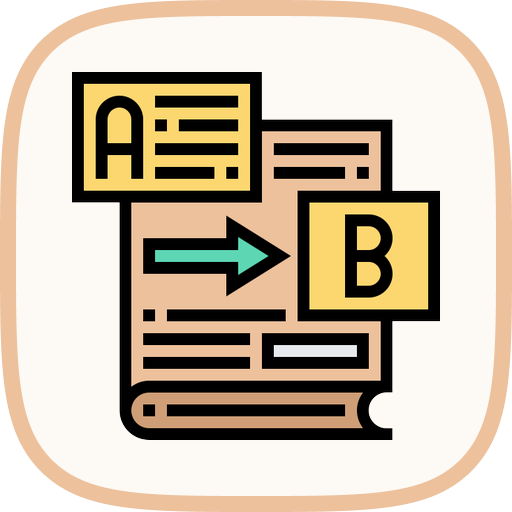
Sorting and Manipulating Go Dictionaries
Unlike other languages, in Go, dictionaries (maps) are unordered collections. In this lab, we will learn about sorting dictionaries and how to use them more flexibly.
Go
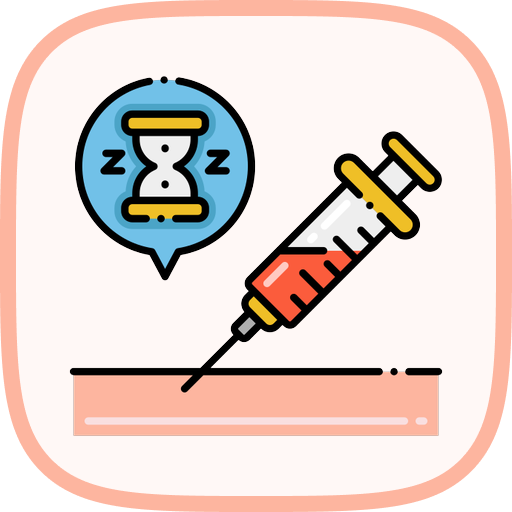
Your First Go Lab
Hi there, welcome to LabEx! In this first lab, you'll learn the classic 'Hello, World!' program in Golang.
Go
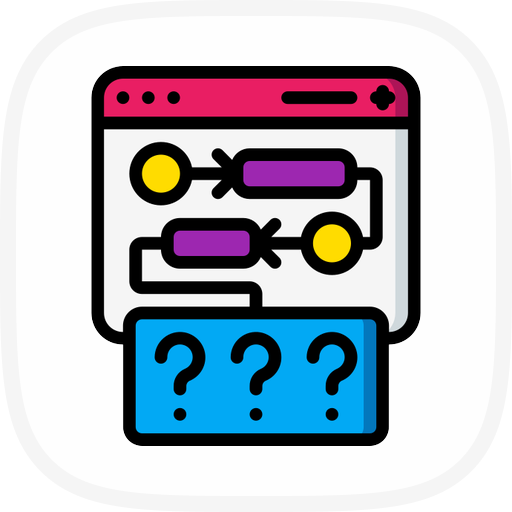
Mastering Anonymous Functions in Go
In the previous experiment, we learned about the use of functions. In Go, there is a special type of function called an anonymous function. It doesn't have a name and is simply referred to as an anonymous function. Let's learn about it together.
Go
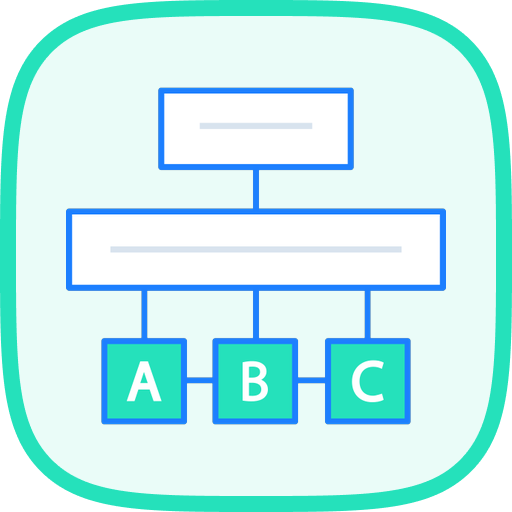
Defining and Using Structures in C
Structure (struct) is a compound type that can be used to store different types of data. It can be used to implement more complex data structures. For example, the items in a convenience store have attributes such as product name, category, and price. We can use a structure to represent them.
Go