Introduction
In this project, you will learn how to create a simple search function using Vue 2. The search function allows users to search for content and displays the relevant results. This project will help you understand the basics of Vue.js and how to implement a search feature in a web application.
๐ Preview
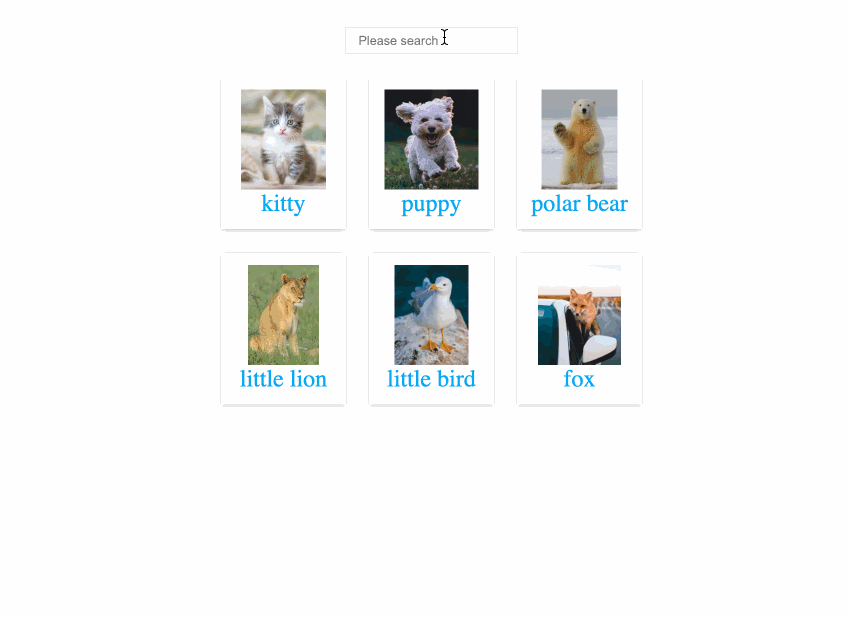
๐ฏ Tasks
In this project, you will learn:
- How to set up the project structure and prepare the necessary files and folders
- How to add the HTML structure to the
index.html
file - How to implement the JavaScript logic to handle the search functionality
- How to style the search input and the search results using CSS
๐ Achievements
After completing this project, you will be able to:
- Use Vue.js to create a responsive and interactive user interface
- Implement a search feature that filters and displays relevant content
- Integrate HTML, CSS, and JavaScript to create a complete web application
- Work with data and computed properties in Vue.js