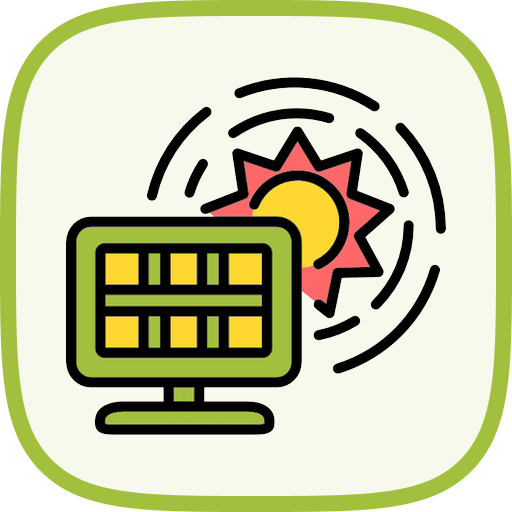
Creating the Solar System in OpenGL
In this project, we will create a solar system simulation using OpenGL. The simulation will include the sun, planets, and their movements and rotations. We will use GLUT (OpenGL Utility Toolkit) to handle window and input functions, and OpenGL for rendering.
C++
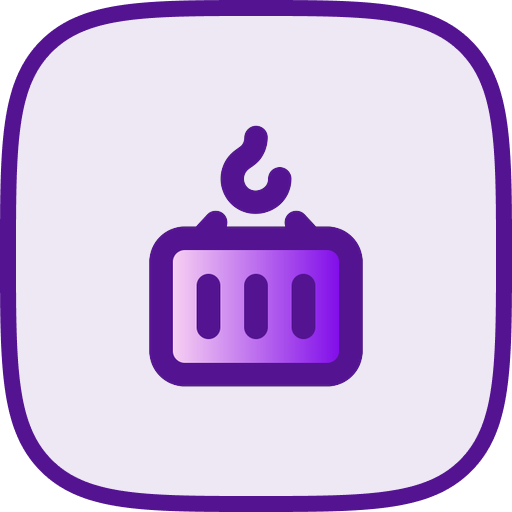
Creating a Simple Docker Container in C++
The essence of Docker is to use LXC to achieve virtual machine-like functionality, thus saving hardware resources and providing users with more computational resources. This project combines C++ with Linux's Namespace and Control Group technologies to implement a simple Docker container.
C++
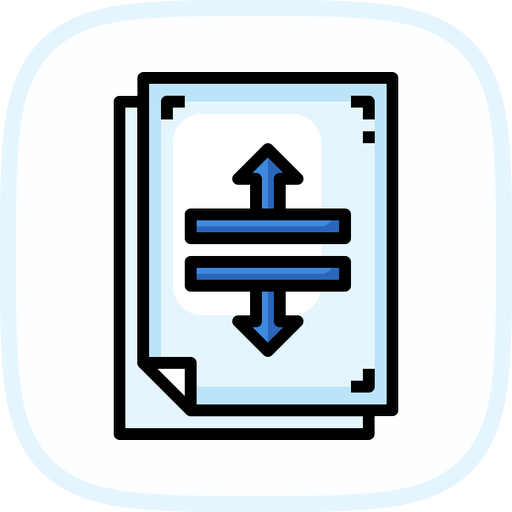
Custom Sort Method for STL Pair Template
In this lab, you will learn how to create a custom sort method for a pair template and implement it using a vector in C++ programming language. This lab assumes that you have prior knowledge of the basics of C++ programming and the concept of the pair template. If you are new to these topics, it is recommended to go through the corresponding tutorials before proceeding.
C++
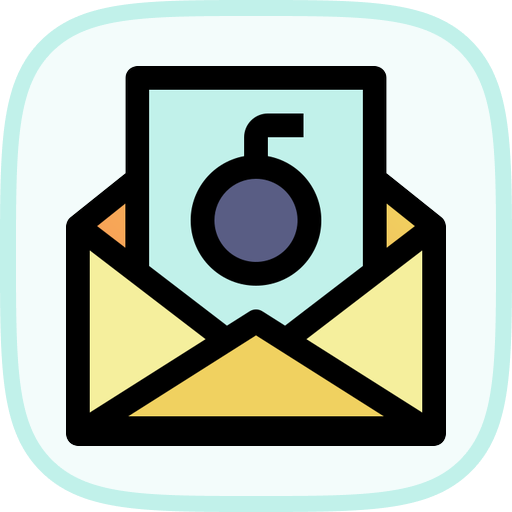
Personalized C++ Greeting
Dive into C++ programming with this beginner-friendly challenge. Learn to modify a simple C++ program to create a personalized greeting, gaining hands-on experience with basic syntax, string manipulation, and output in C++.
C++
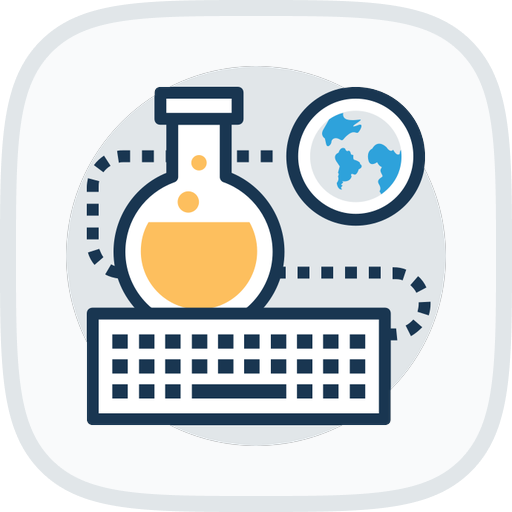
Your First C++ Lab
Embark on your C++ programming journey with this beginner-friendly lab. Learn to write, compile, and run your first C++ program, explore basic output operations, and understand the fundamentals of variables in C++.
C++
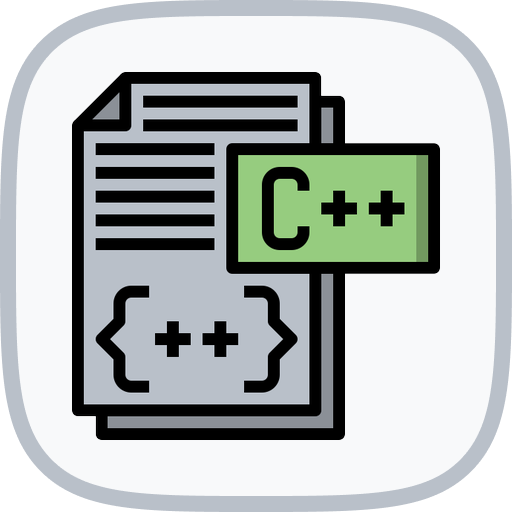
Basic Syntax of C++
Master the fundamentals of C++ programming in this comprehensive lab. Learn about program structure, variables, data types, control structures, functions, and input/output operations through hands-on coding exercises.
C++
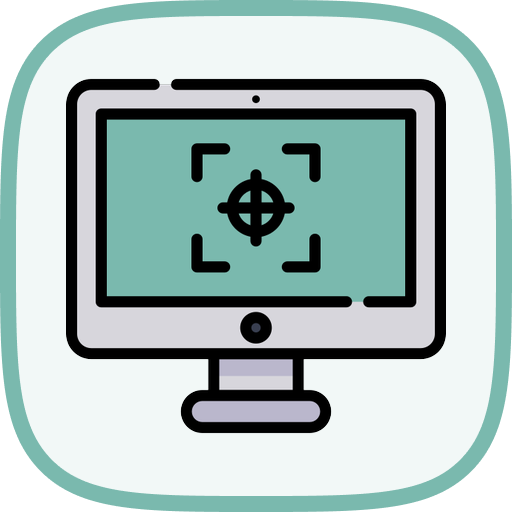
Video Object Tracking by Using OpenCV
In this lab, we will implement video object tracking using OpenCV.
C++
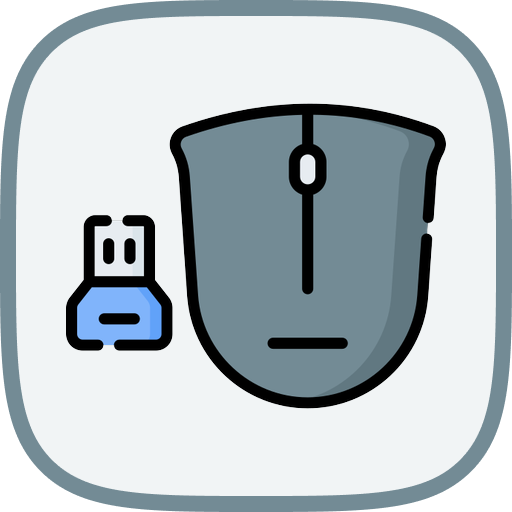
Memory Leak Detector with C++
Memory leak has always been one of the hardest problems, even for senior programmers. Memory leak still proceeds when they get lapsed for some time. Besides the basic leak phenomenon of allocated memory, there are many different types of memory leak, such as exception branch leaking etc. This project guides you to implement a memory leak detector.
C++
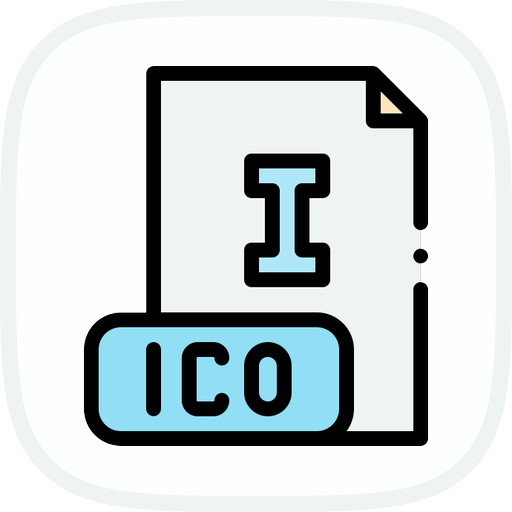
C++ Formatting, File IO and Namespace
In this lab, you will learn the formatting, file I/O, and namespace in C++. You will learn how to format output, how to format input, how to read and write files, and how to use namespaces.
C++
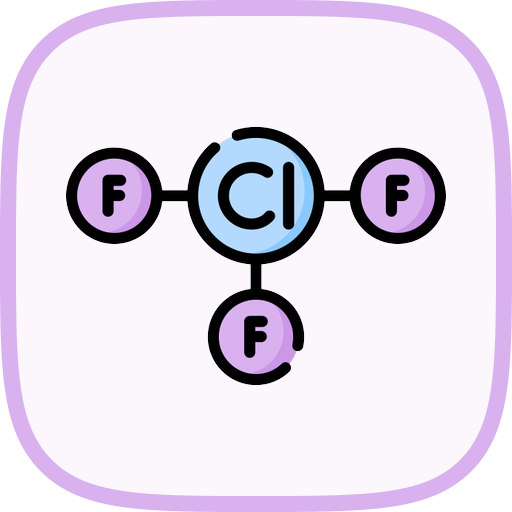
C++ Variables and Types
In this lab, you will learn the variables and types in C++. You will learn how to define variables, and how to use different types of variables.
C++
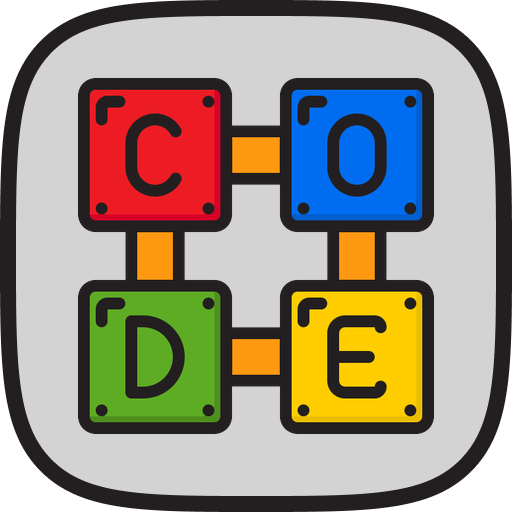
C++ String Fundamentals
In this lab, you will learn strings in C++. You will learn how to define and initialize strings, and how to use string functions.
C++
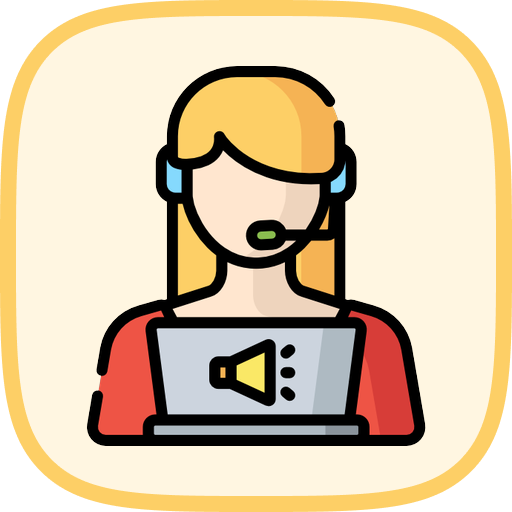
C++ Operators
In this lab, you will learn how to use operators in C++. You will learn how to use arithmetic operators, relational operators, logical operators, and bitwise operators.
C++
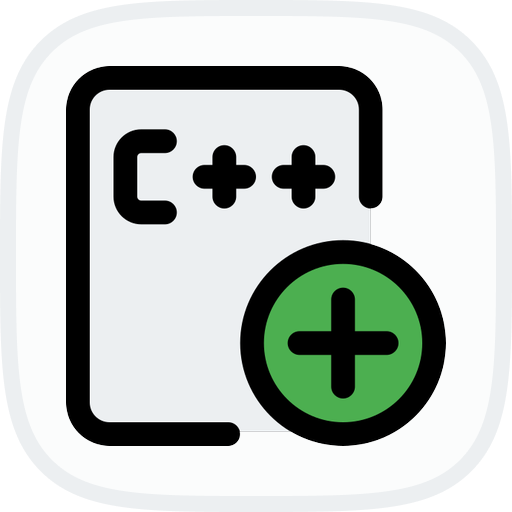
C++ Function Essentials
In this lab, you will learn the functions in C++. You will learn how to define and call functions, and how to pass arguments to functions.
C++
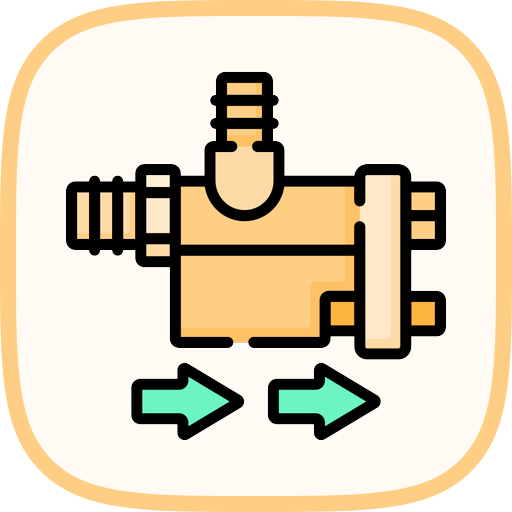
C++ Flow Control
In this lab, you will learn the control flow statements in C++. You will learn how to use if-else statements, switch statements, while loops, do-while loops, and for loops.
C++
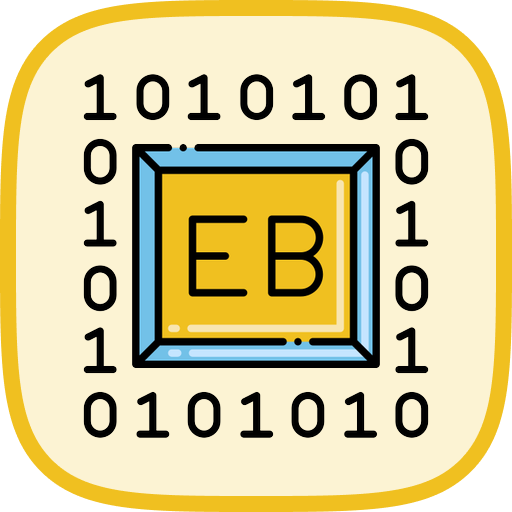
C++ Arrays Fundamentals
In this lab, you will learn the arrays in C++. You will learn how to define and initialize arrays, and how to use array functions.
C++
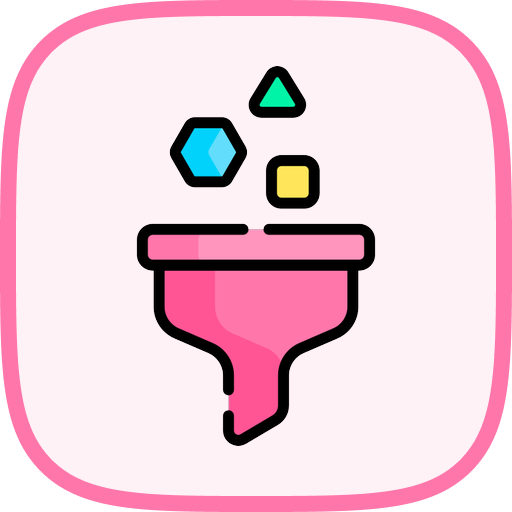
Bubble Sort Using Dynamic Array
In this lab, we will learn to write an algorithm to implement bubble sort technique in C++. Bubble sort is one of the most popular and simple sorting techniques, where we compare two adjacent elements in an array and swap their positions if they aren't arranged correctly.
C++
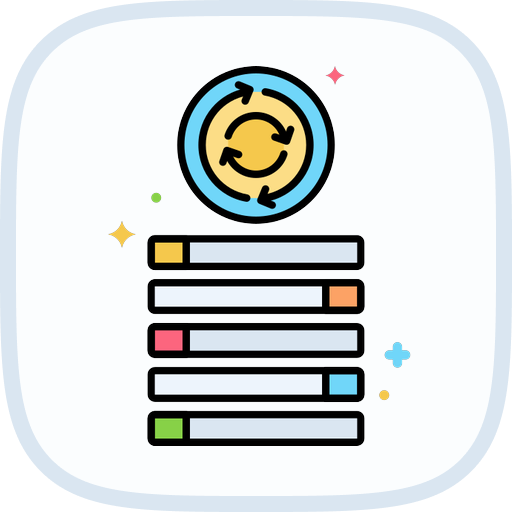
C++ STL Stack
In this lab, we will learn how to create and manipulate a Stack data structure in C++. We will be using the STL (Standard Template Library) provided by C++ to create the stack object.
C++
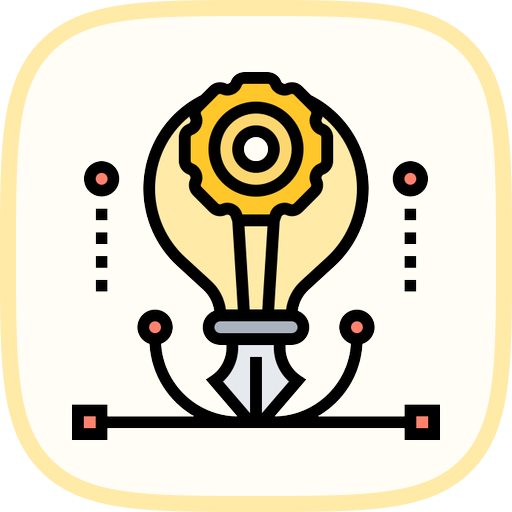
Initializing a Vector
In this lab, we will learn how to initialize vectors in C++. A vector is a dynamically resizable array with the ability to resize itself depending on the number of elements to be inserted or deleted. Vectors are more advantageous than ordinary arrays, which are of fixed size and are static in nature.
C++