Introduction
In this project, you will learn how to create a traffic lights system that changes the color of the light from red to green after a certain time interval. This project will help you understand the basics of JavaScript and how to manipulate the DOM to display different elements based on time-based events.
👀 Preview
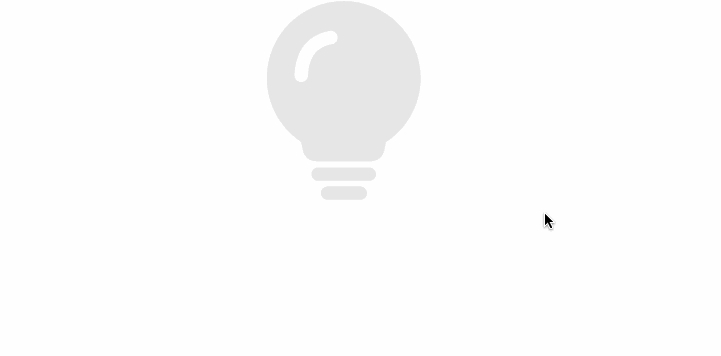
🎯 Tasks
In this project, you will learn:
- How to set up a basic HTML and JavaScript project structure
- How to implement a function to change the display of the traffic light to red after 3 seconds
- How to implement a function to change the display of the traffic light to green after 6 seconds
- How to coordinate the execution of the red and green light functions using async/await
- How to test the traffic lights system and ensure it works as expected
🏆 Achievements
After completing this project, you will be able to:
- Manipulate the DOM using JavaScript to change the display of HTML elements
- Use setTimeout() to schedule time-based events
- Manage asynchronous operations with async/await
- Test and debug your JavaScript code