Introduction
In this project, you will learn how to create a responsive product list layout with a switching feature. This feature allows users to easily toggle between a grid view and a list view of the product items.
👀 Preview
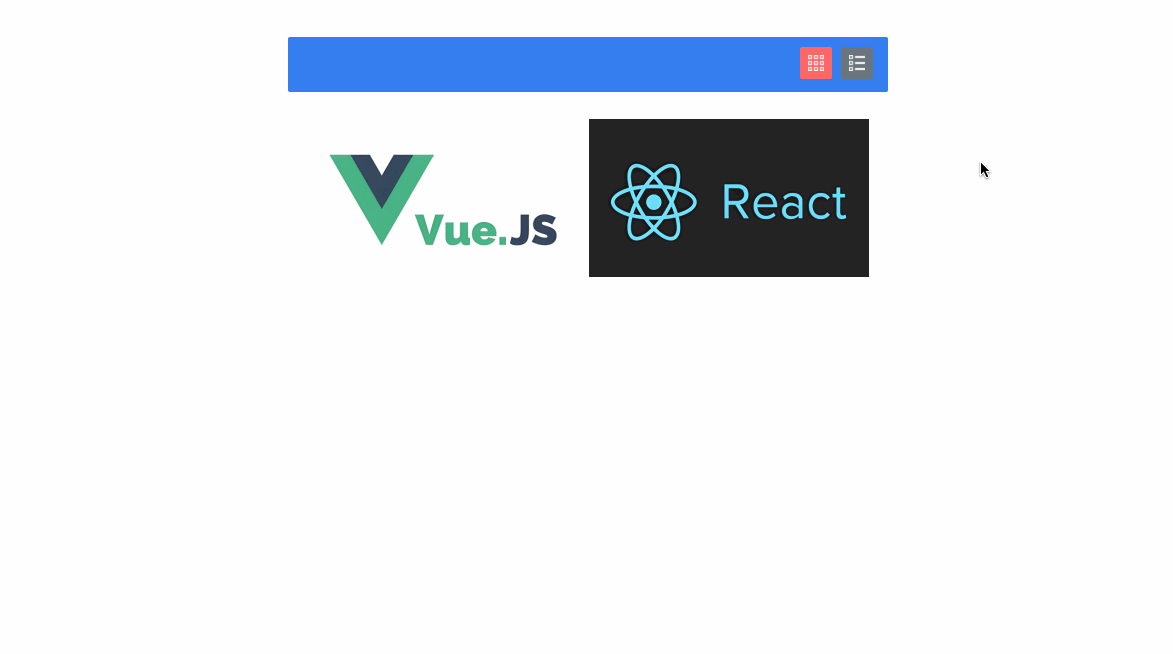
🎯 Tasks
In this project, you will learn:
- How to set up the project structure and understand the purpose of each file and folder
- How to fetch data from a JSON file and populate the product list
- How to implement the layout switching functionality using Vue.js
- How to conditionally render the grid and list layouts based on the selected view
🏆 Achievements
After completing this project, you will be able to:
- Structure a Vue.js project effectively
- Use Axios to fetch data from a JSON file
- Utilize Vue.js directives like
v-if
andv-else
to conditionally render content - Handle user interactions and update the UI accordingly