Introduction
In this project, you will learn how to build a simple "Guess the Coin" game. The game involves randomly selecting three cups out of nine cups to place coins in, and the player has to guess the correct cups by inputting the corresponding numbers.
👀 Preview
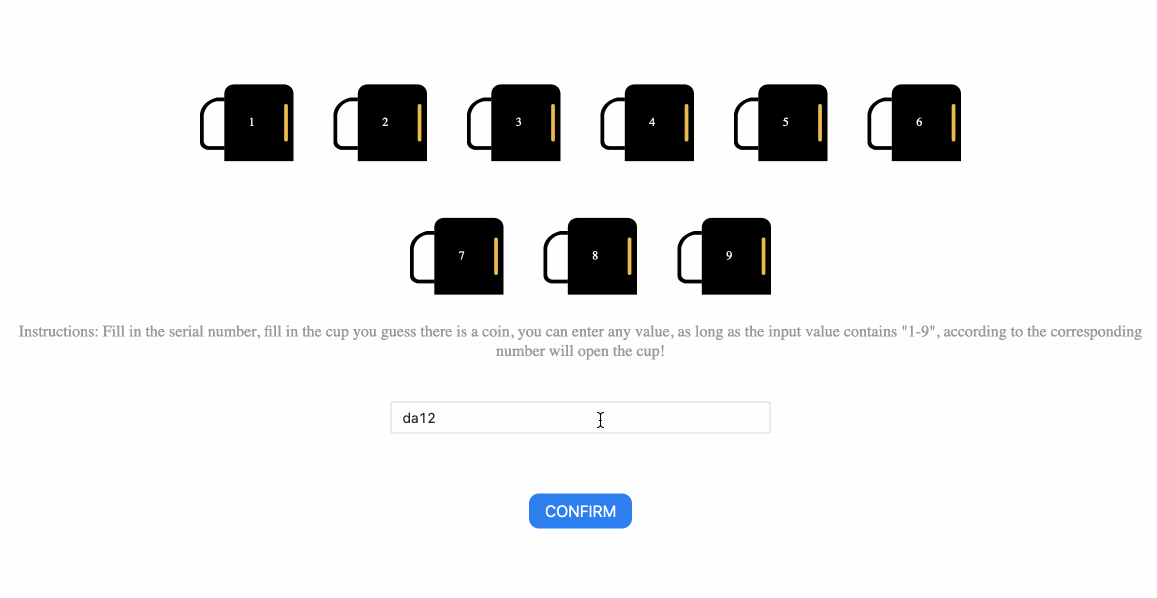
🎯 Tasks
In this project, you will learn:
- Implement the
findNum
function to extract unique numbers from the user's input. - Implement the
randomCoin
function to generate three non-repeating random numbers between 1 and 9. - Understand the project's directory structure and the provided files.
🏆 Achievements
After completing this project, you will be able to:
- Work with JavaScript functions and arrays.
- Use regular expressions to extract specific data from a string.
- Generate random numbers and ensure they are unique.
- Follow step-by-step instructions to complete a project.