Introduction
In this project, you will learn how to create a personal business card using React. The project involves building a responsive and interactive web application that allows users to input their personal information and generate a customized business card.
👀 Preview
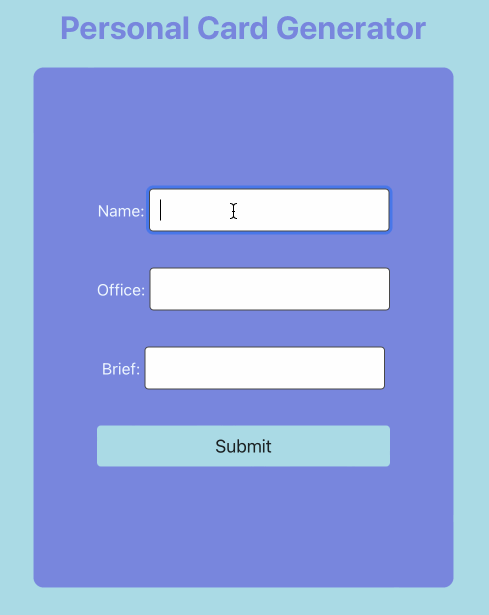
🎯 Tasks
In this project, you will learn:
- How to set up a React project and install necessary dependencies
- How to implement a
ProfileCard
component using theuseForm
hook from thereact-hook-form
library - How to integrate the
ProfileCard
component into the mainApp
component - How to style the application using CSS to create an appealing and visually consistent design
🏆 Achievements
After completing this project, you will be able to:
- Use the
useForm
hook from thereact-hook-form
library to manage form state and validation - Create reusable React components and integrate them into a larger application
- Style a React application using CSS to achieve a desired visual appearance
- Understand the importance of modular and maintainable code structure in React projects