Introduction
In this project, you will learn how to create a real-time sales dashboard using the Echarts library. The dashboard will display the sales and volume data in a visually appealing and easy-to-understand format.
👀 Preview
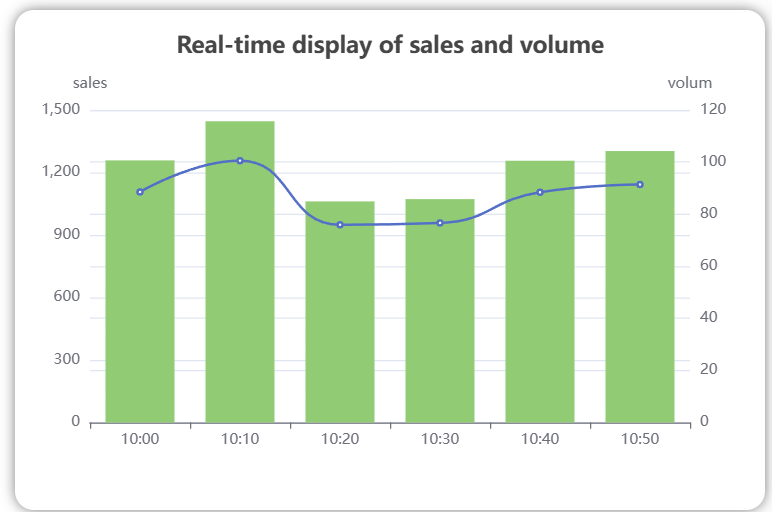
🎯 Tasks
In this project, you will learn:
- How to set up the project and get the necessary files
- How to complete the "yAxis" setting in the
index.js
file - How to correctly assign the values from the backend data to the appropriate chart options in the
renderChart
function
🏆 Achievements
After completing this project, you will be able to:
- Render data into charts using the Echarts library
- Structure and organize your code for a data visualization project
- Work with asynchronous data retrieval and update the chart in real-time