Introduction
In this project, you will learn how to develop a RequestControl
class that collects launch requests for spacecraft and automatically arranges the launch of the next batch of spacecraft based on the results of the spacecraft. This will help humans escape the impact of the "Dual Vector Foil" of the three-body problem, which collapses three-dimensional space into a two-dimensional plane.
👀 Preview
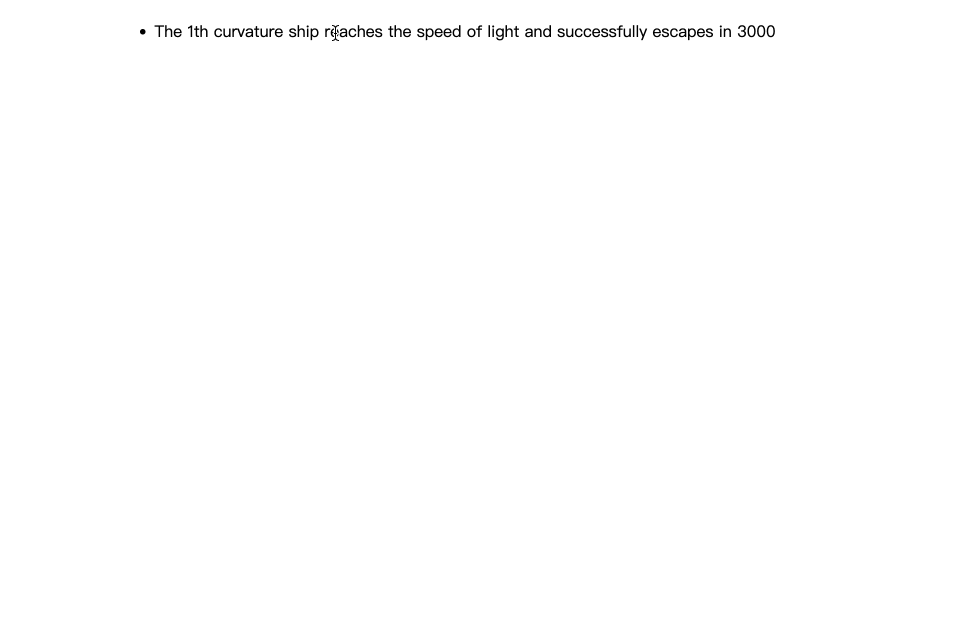
🎯 Tasks
In this project, you will learn:
- How to implement the
run
function in theRequestControl
class to handle the launch of spacecraft. - How to render the launch results on the page using the
render
function.
🏆 Achievements
After completing this project, you will be able to:
- Use promises to simulate the spacecraft launch process.
- Manage the launch queue and control the number of spacecraft that can be launched at a time.
- Update the user interface to display the launch results.