Introduction
In this project, you will learn how to create a holiday greeting card that displays random greetings when the "Write" button is clicked. This project will help you understand how to work with JavaScript functions, event listeners, and DOM manipulation.
👀 Preview
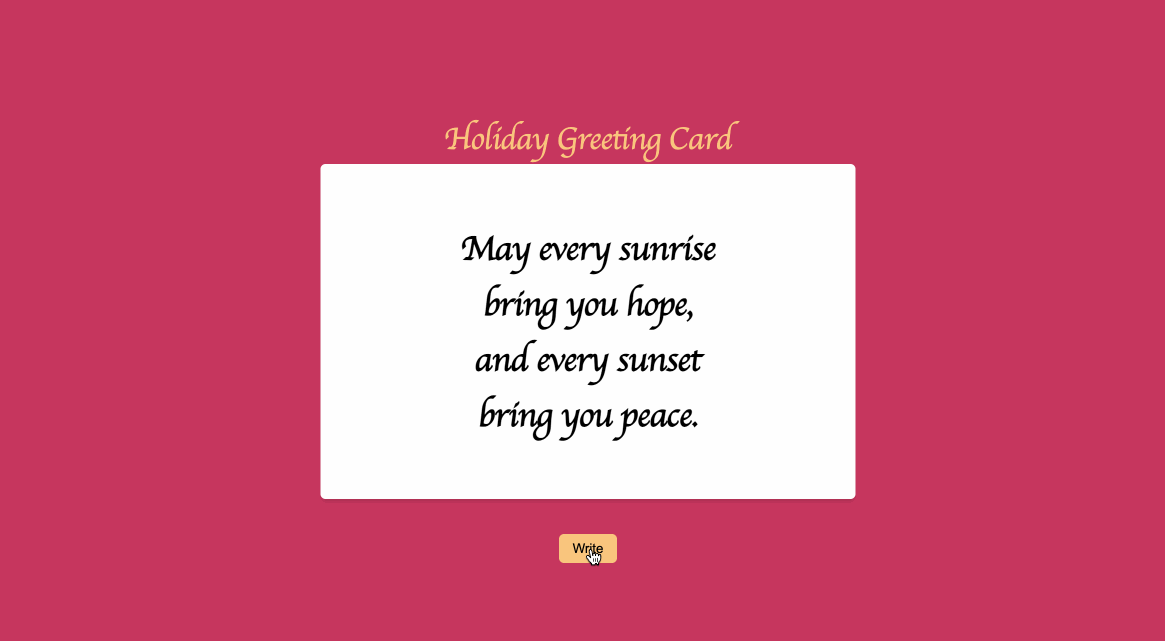
🎯 Tasks
In this project, you will learn:
- How to set up the project environment
- How to implement a function to randomly select a greeting from a pre-defined array
- How to implement a function to display the selected greeting in the greeting card
- How to add an event listener to the "Write" button to trigger the greeting display
🏆 Achievements
After completing this project, you will be able to:
- Use JavaScript functions to generate random content
- Manipulate the DOM to update the content of an HTML element
- Add event listeners to trigger specific actions
- Work with a provided project structure and follow step-by-step instructions