Introduction
In this project, you will learn how to build a movie ticket reservation system. The project involves fetching movie data asynchronously, rendering the movie information on the web page, and implementing the functionality of seat selection and total price calculation.
👀 Preview
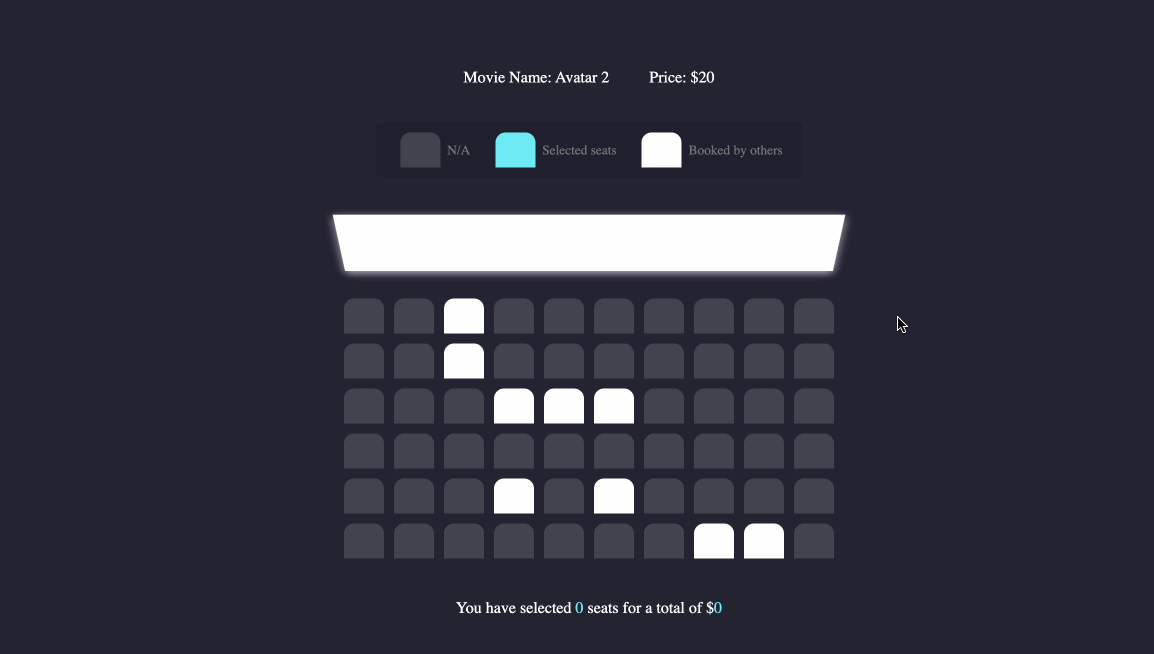
🎯 Tasks
In this project, you will learn:
- How to fetch data asynchronously using the
axios
library - How to render movie information, including the movie name, price, and seating availability, on the web page
- How to implement the functionality of seat selection, updating the number of selected seats and the total price accordingly
- How to update the display to show the current number of selected seats and the total price
🏆 Achievements
After completing this project, you will be able to:
- Fetch data asynchronously using the
axios
library - Manipulate the DOM to display dynamic content
- Implement interactive user interfaces with event handling
- Update the UI based on user actions