Introduction
In this project, you will learn how to implement a portal-like functionality on a web page. The portal feature allows users to quickly locate and navigate to their desired content on a long webpage.
š Preview
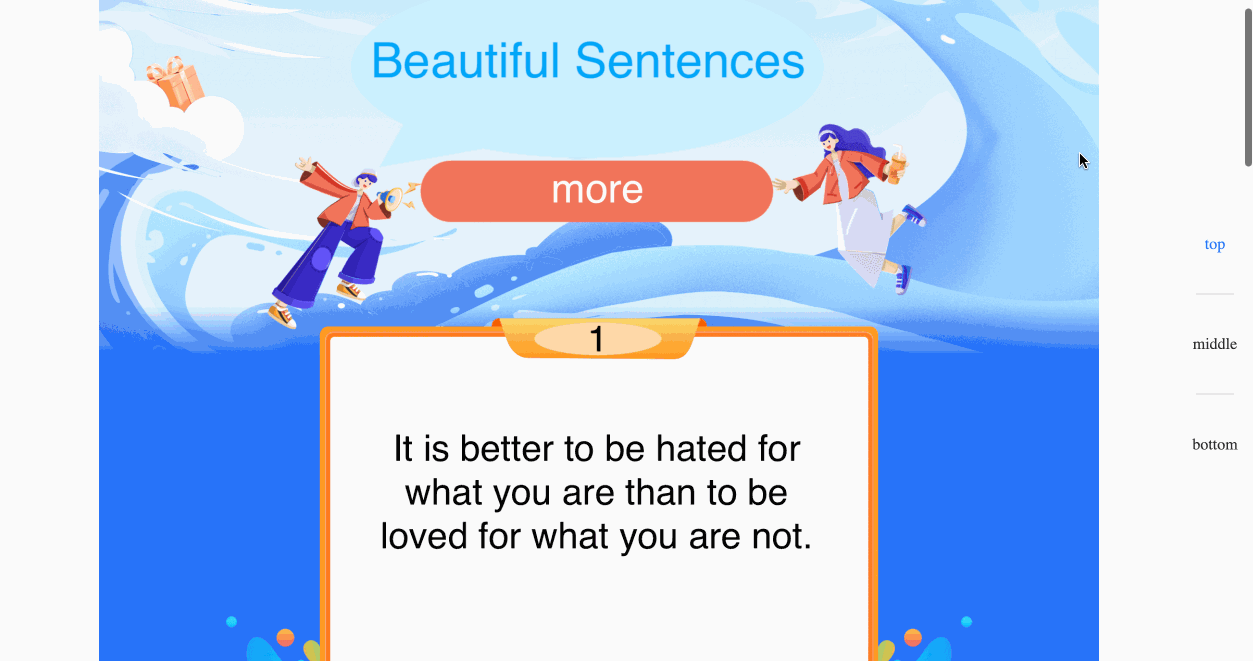
šÆ Tasks
In this project, you will learn:
- How to set up the project files and structure
- How to implement the portal functionality using JavaScript and jQuery
- How to ensure the side buttons change color based on the user's scroll position
š Achievements
After completing this project, you will be able to:
- Structure and set up a web development project
- Use JavaScript and jQuery to manipulate the DOM and handle user interactions
- Implement a portal-like feature to enhance the user experience on a long webpage