Introduction
In this project, you will learn how to implement a step-by-step form with a progress bar using jQuery. This project is designed to help you understand the process of creating a dynamic and interactive form that allows users to navigate through different steps easily.
š Preview
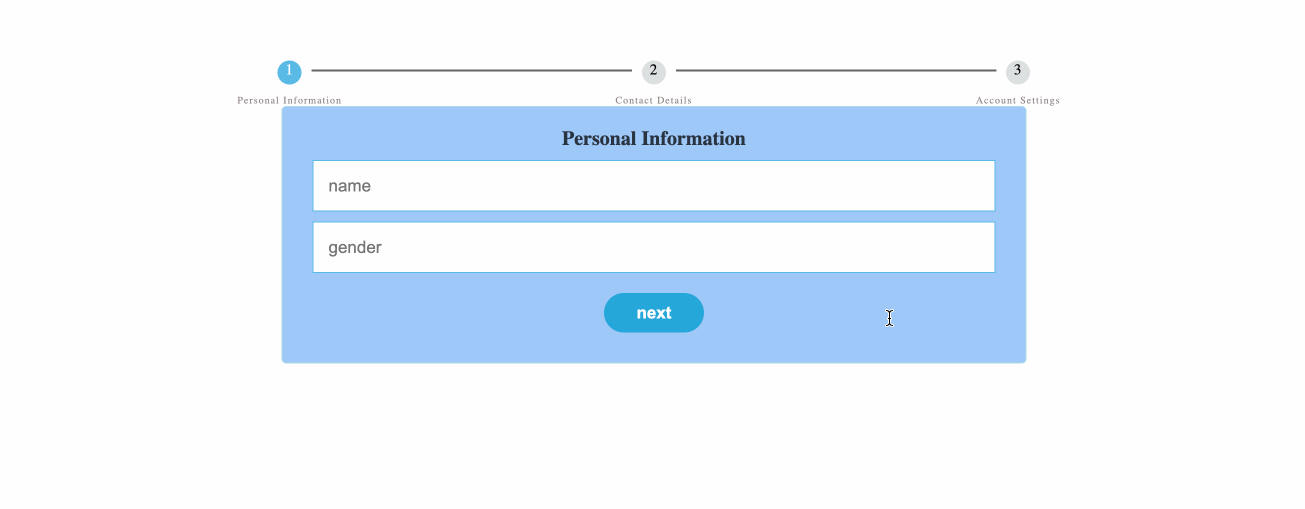
šÆ Tasks
In this project, you will learn:
- How to implement the functionality of the "Next Page" button to switch to the next step of the form.
- How to implement the functionality of the "Previous" button to switch to the previous step of the form.
- How to implement the functionality of the "Submit" button to display a success message.
- How to update the progress bar to reflect the current step of the form.
š Achievements
After completing this project, you will be able to:
- Use jQuery to manipulate the DOM and handle user interactions.
- Control the display of form fields using the
display
property. - Update the progress bar to reflect the current step of the form.
- Create a step-by-step form with a clean and intuitive user interface.