Introduction
In this project, you will learn how to implement a card binding function using jQuery and AJAX. This project is designed to help you understand the basics of data dynamization, which is a crucial skill in front-end development.
๐ Preview
The successful results are as follows:
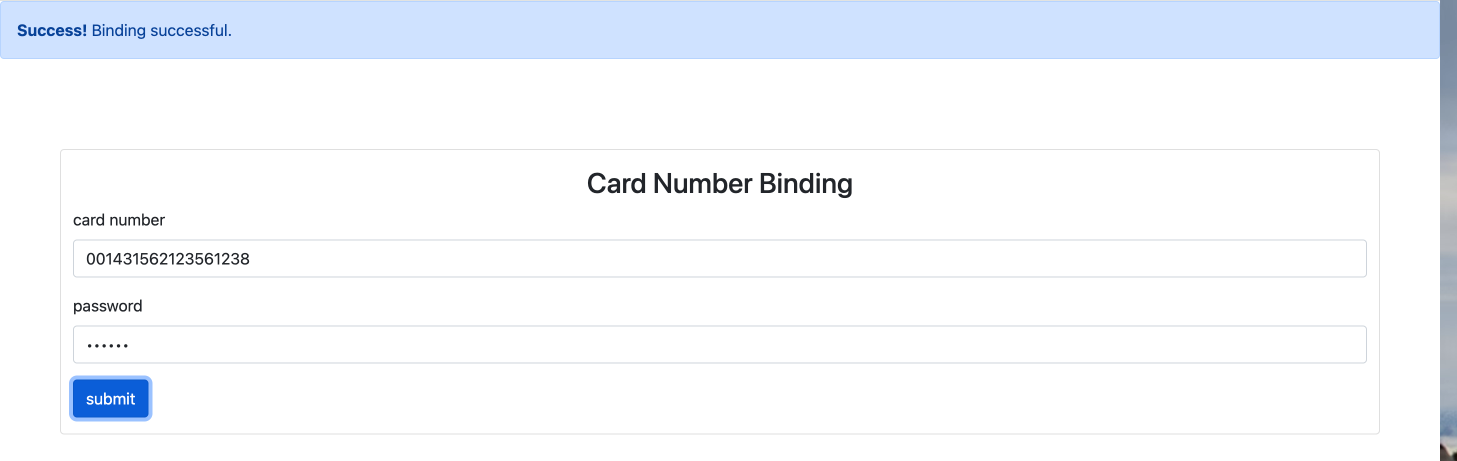
The failure effects are as follows:
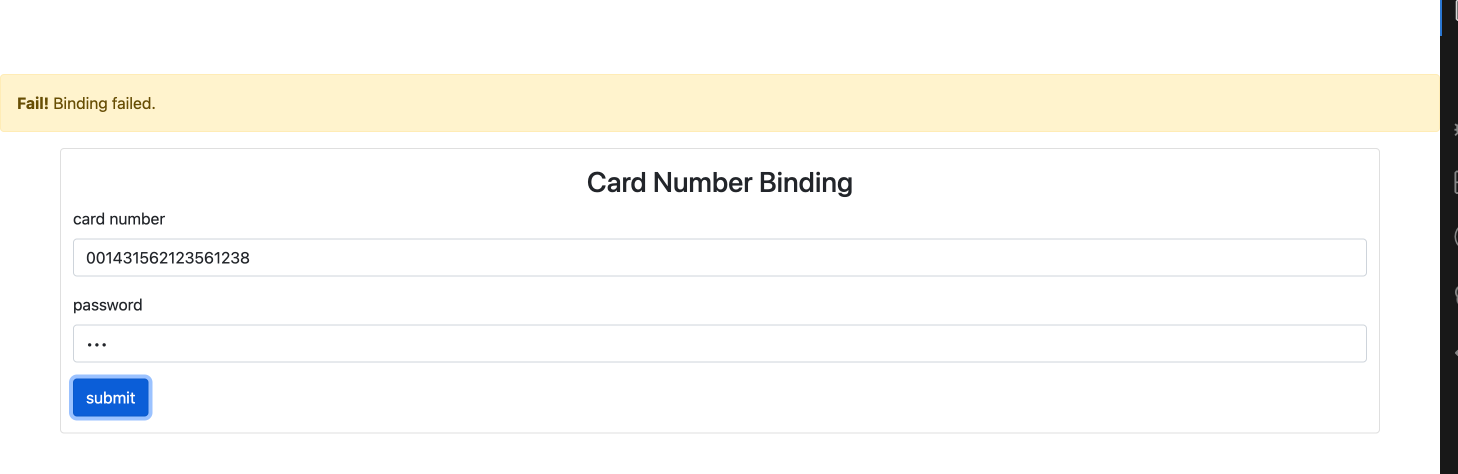
๐ฏ Tasks
In this project, you will learn:
- How to use jQuery's
ajax
function to retrieve data from a JSON file - How to compare user input with the retrieved card data
- How to display success or failure messages based on the comparison
๐ Achievements
After completing this project, you will be able to:
- Implement a card binding function using jQuery and AJAX
- Understand the basics of data dynamization, a crucial skill in front-end development
- Make AJAX requests to retrieve data from a server
- Manipulate the DOM using jQuery to display dynamic content
- Use Bootstrap classes to control the display and hiding of alerts