Introduction
In this project, you will learn how to build a voting application that allows users to create and manage voting options, as well as delete options if needed. The application also includes features for multi-selection support and public voting results.
👀 Preview
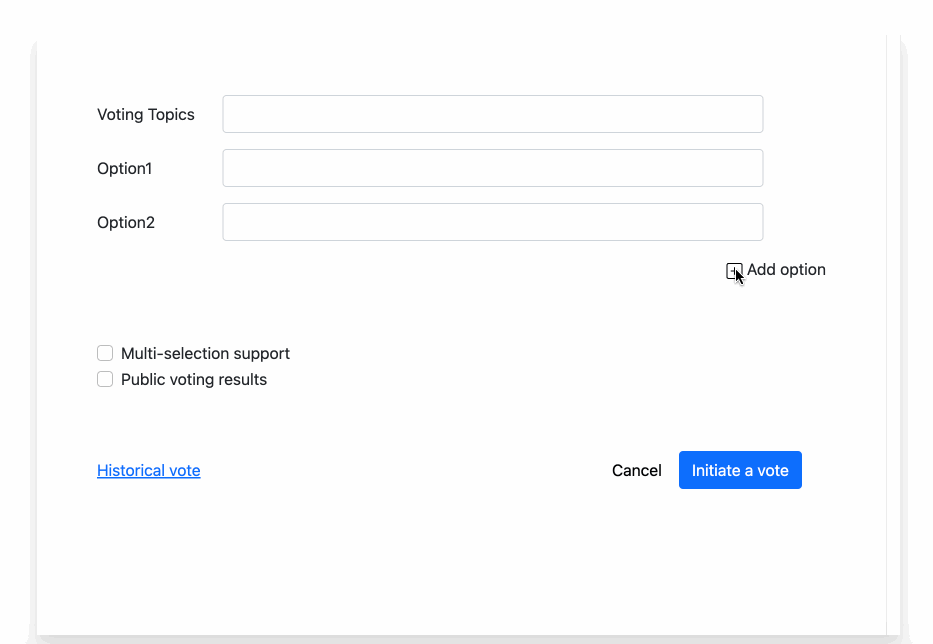
🎯 Tasks
In this project, you will learn:
- How to initialize the HTML structure for the voting application
- How to implement the functionality to add new voting options
- How to implement the functionality to delete voting options
- How to test the application to ensure the functionality works as expected
🏆 Achievements
After completing this project, you will be able to:
- Set up the basic HTML structure for a voting application
- Use JavaScript to dynamically add and remove voting options from the application
- Handle user interactions, such as clicking the "Add option" button and the delete icon
- Test the application to ensure it meets the requirements
- Build interactive web applications using HTML, CSS, and JavaScript