Introduction
In this project, you will learn how to create an interactive collection of films web application using HTML, CSS, and JavaScript (jQuery). The application allows users to mark their favorite films, and when a collection of favorite films is created, a success message is displayed.
👀 Preview
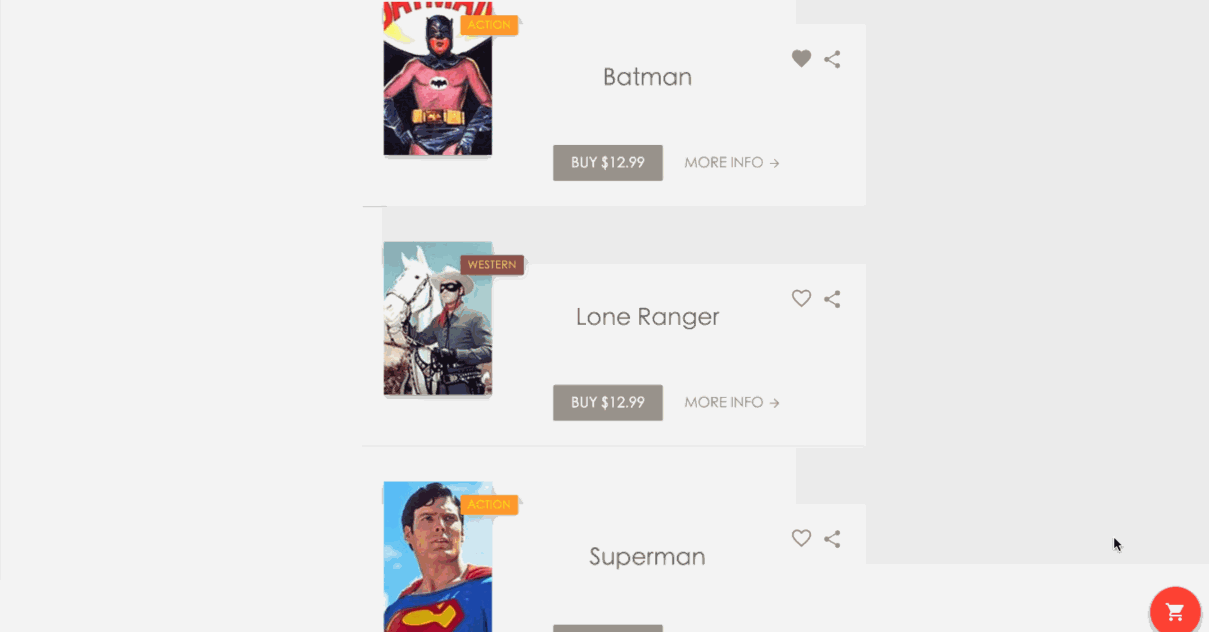
🎯 Tasks
In this project, you will learn:
- How to set up the project files and open the project in the editor
- How to implement the functionality for the favorite icon, allowing users to toggle between hollow and solid states
- How to implement the functionality for the collection icon, displaying a success message when at least one favorite film is added
🏆 Achievements
After completing this project, you will be able to:
- Manipulate the DOM using jQuery
- Handle user interactions and events
- Display and hide elements based on specific conditions
- Use timers to automatically hide elements after a certain duration