Introduction
In this project, you will learn how to integrate the Log4j2 logging framework into a Spring Boot application. You will configure Log4j2 to log project information in both the console and log files.
👀 Preview
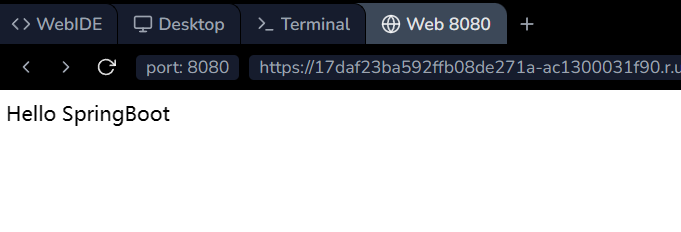
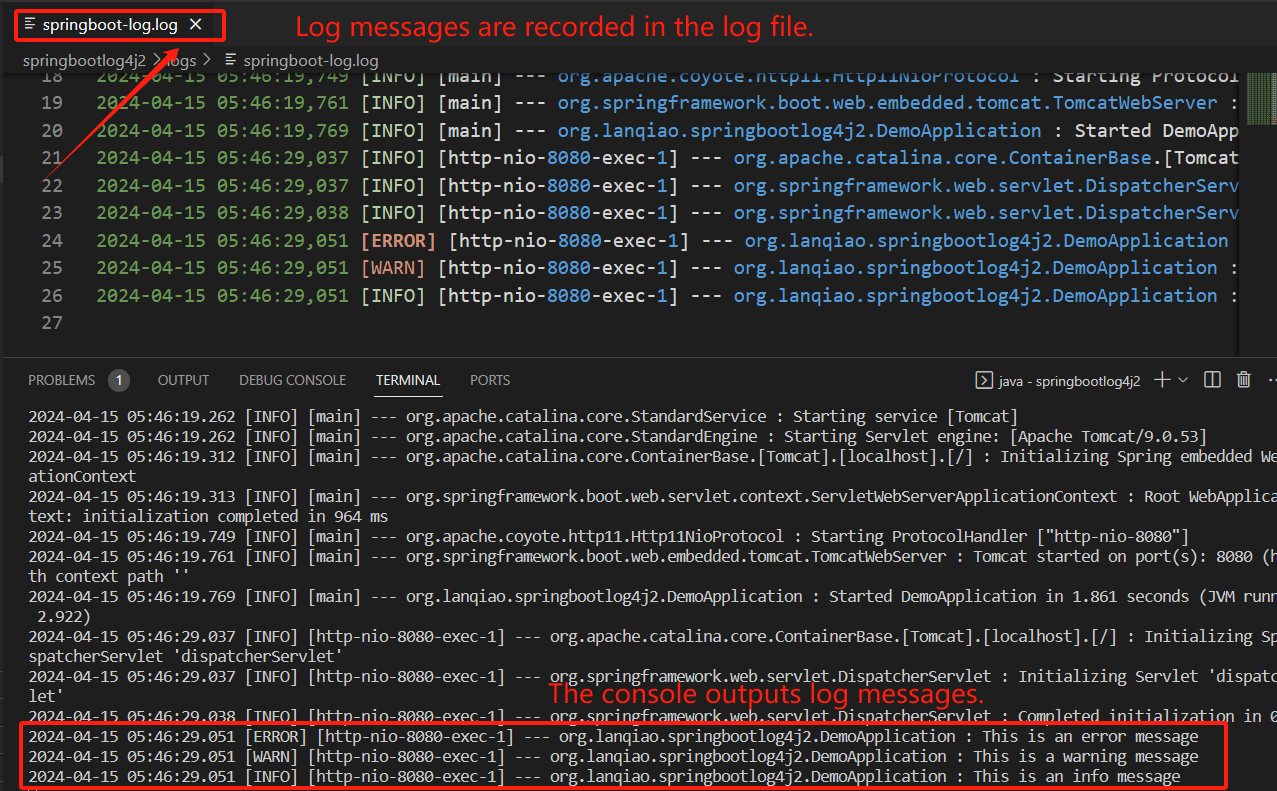
🎯 Tasks
In this project, you will learn:
- How to modify the
pom.xml
file to add the necessary Log4j2 dependencies - How to configure Log4j2 in the
application.properties
file - How to create a logger and log information using different log levels in the
DemoApplication.java
file - How to package and run the Spring Boot application to view the logged information
🏆 Achievements
After completing this project, you will be able to:
- Use the Log4j2 logging framework in a Spring Boot application
- Configure Log4j2 to log information in the console and log files
- Create a logger and use different log levels to log information
- Package and run a Spring Boot application