Introduction
In this project, you will learn how to convert and format table data to be displayed in a user-friendly way. This is a common task that frontend developers often encounter when working with data from the backend.
👀 Preview
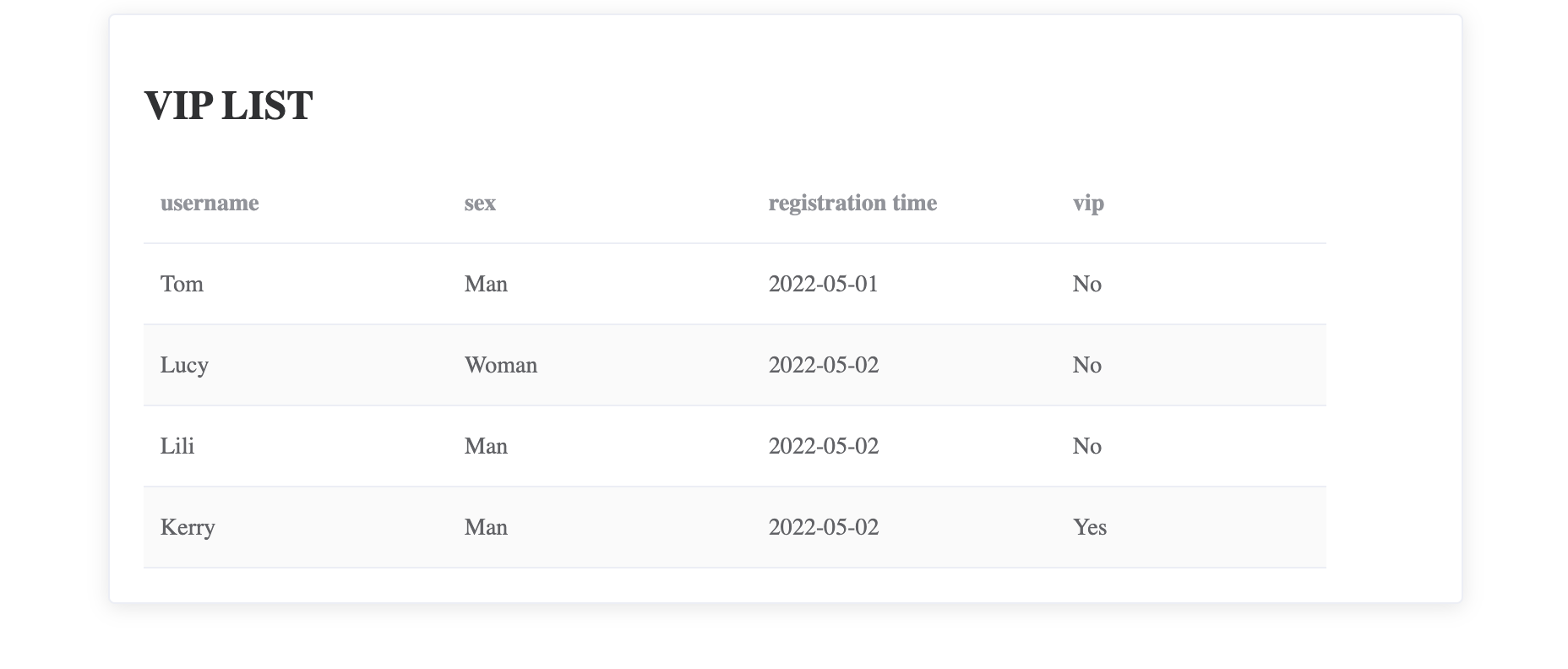
🎯 Tasks
In this project, you will learn:
- How to send an HTTP request to fetch data from a JSON file
- How to format the data to match the required format for display
- How to render the formatted data in an HTML table using Vue.js and Element UI
🏆 Achievements
After completing this project, you will be able to:
- Use
axios
to send HTTP requests and fetch data - Manipulate and transform data to the desired format
- Integrate Vue.js and Element UI to create a responsive and visually appealing table component