Introduction
In this project, you will learn how to create a simple prize draw application using JavaScript and jQuery. The application will simulate a prize draw by rotating a list of prizes and displaying the winning prize when the rotation stops.
👀 Preview
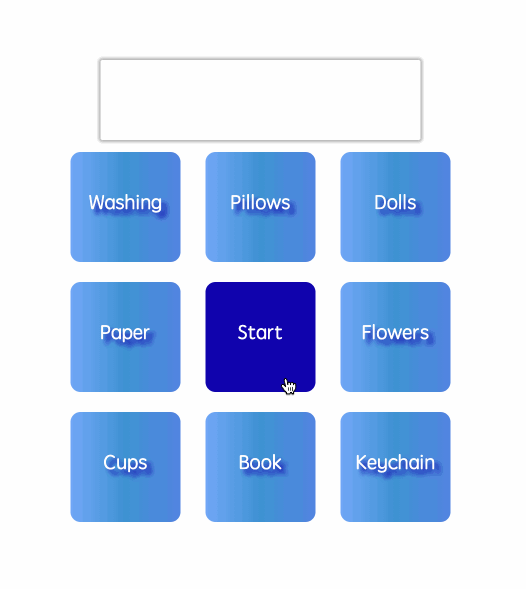
🎯 Tasks
In this project, you will learn:
- How to set up the project files and directories
- How to implement the
rolling
function to handle the prize rotation and display the winning prize - How to test the project to ensure it works as expected
🏆 Achievements
After completing this project, you will be able to:
- Use JavaScript and jQuery to create interactive web applications
- Implement a simple animation loop using
requestAnimationFrame
- Handle user interactions and update the UI accordingly