Introduction
In this project, you will learn how to create a reverse proxy using Node.js. A reverse proxy is a server that sits between a client and a backend server, forwarding requests from the client to the backend server and returning the response back to the client.
👀 Preview
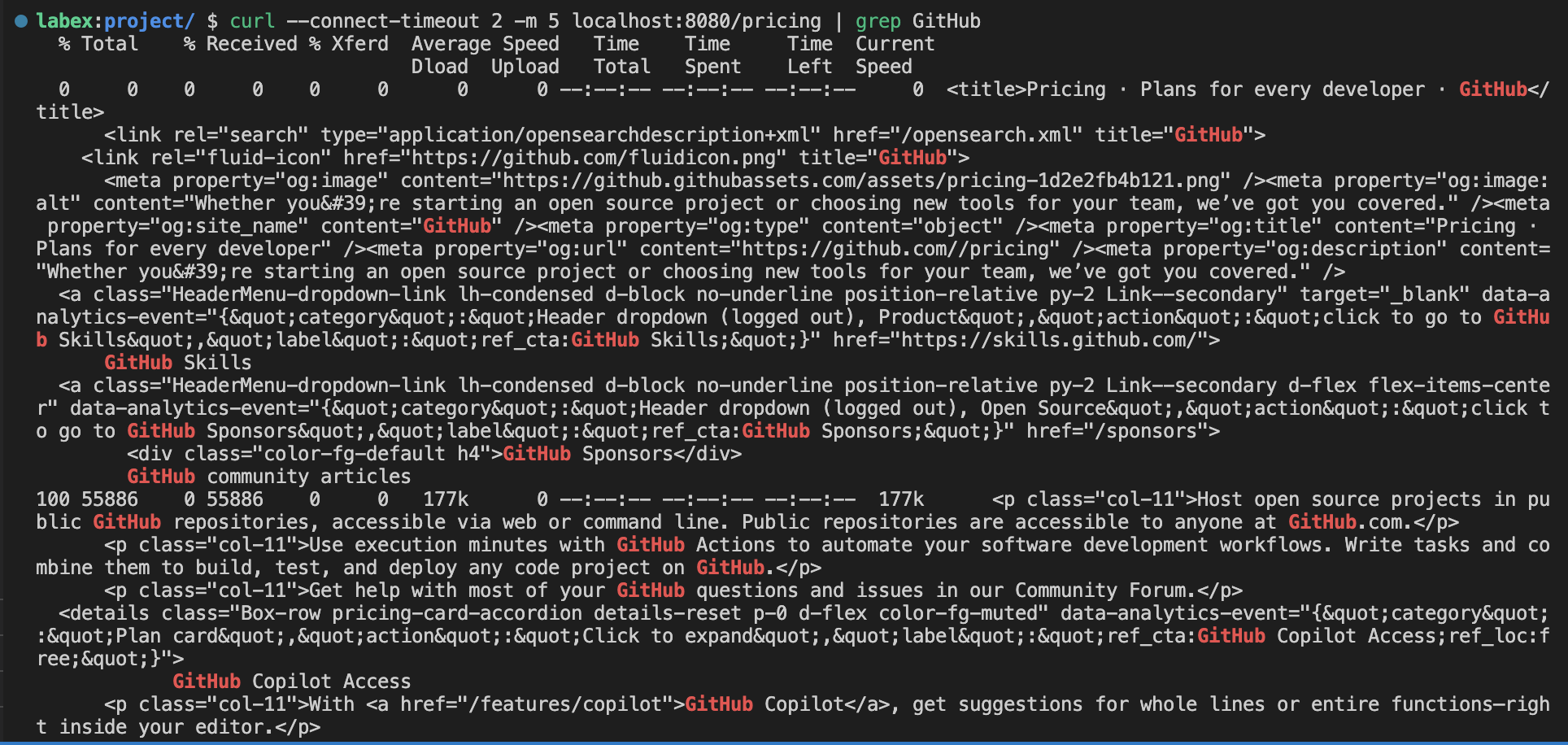
🎯 Tasks
In this project, you will learn:
- How to create an HTTP server using Node.js
- How to use the
Stream pipe
to pass the GitHub response data directly to the client - How to start the reverse proxy server and test it
🏆 Achievements
After completing this project, you will be able to:
- Understand the concept of a reverse proxy and how it works
- Create a reverse proxy using Node.js to proxy requests to the GitHub website
- Implement the reverse proxy functionality using the
Stream pipe
- Start and test the reverse proxy server