Introduction
In this project, you will learn how to build a web conferencing application using Vue.js 2.x. The project focuses on implementing various display effects for the list of attendees in the web conferencing software.
ð Preview
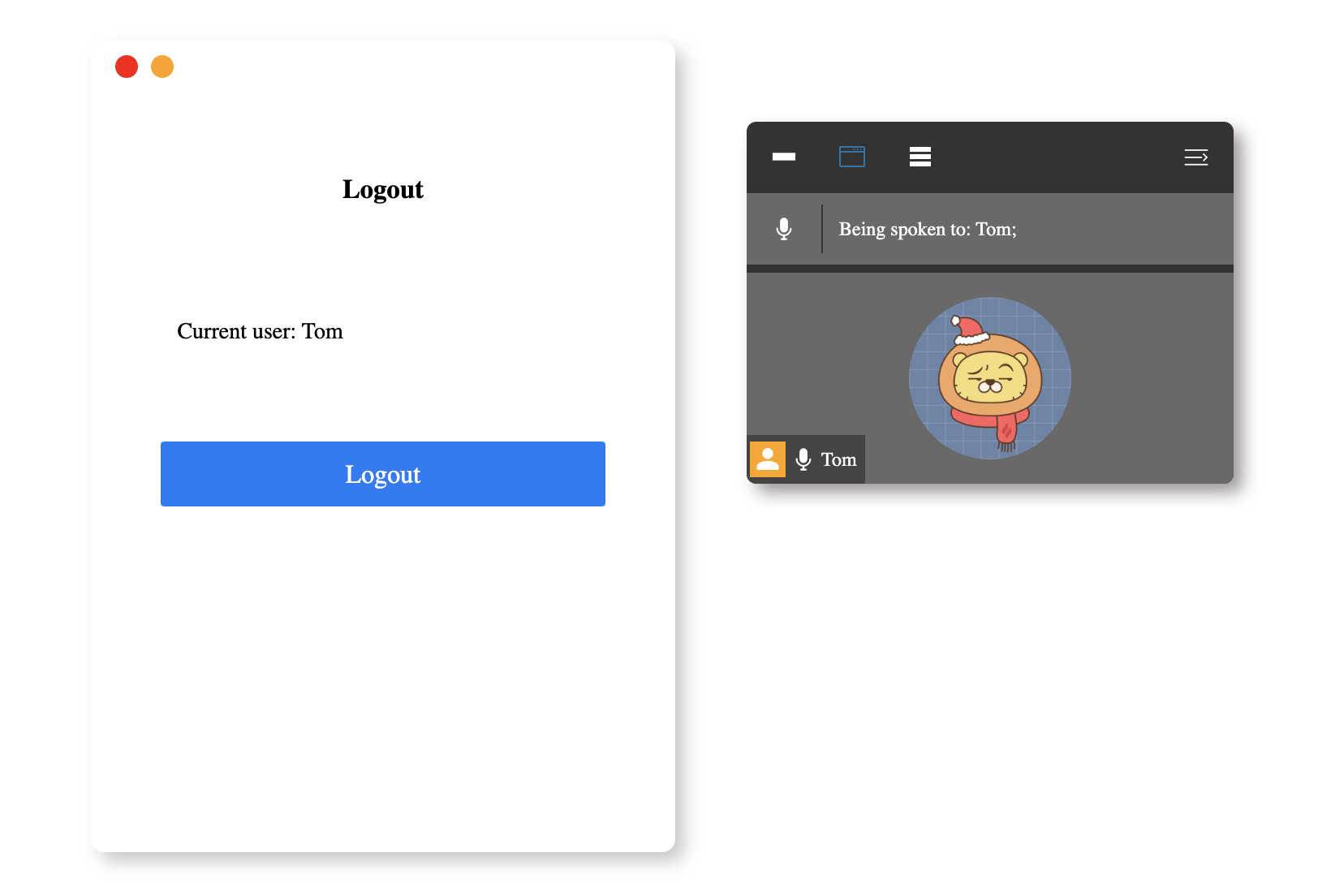
ðŊ Tasks
In this project, you will learn:
- How to implement asynchronous data reading and rendering functionality to fetch and display user data in the login and participant windows.
- How to implement the login and logout switching function, allowing users to log in and out of the application.
- How to ensure the logged-in user is always displayed as the first user in the participant list.
- How to implement the functionality of switching the visibility of the participant window.
- How to implement the functionality of switching the display effect of the participant list, including showing all participants, hiding the participant list, and only showing the currently logged-in user.
ð Achievements
After completing this project, you will be able to:
- Build a web conferencing application using Vue.js 2.x with various user interface features.
- Fetch and display data asynchronously using Axios.
- Implement state management and event handling in a Vue.js application.
- Create dynamic user interfaces and switch between different display modes.