Introduction
In this project, you will learn how to build a simple Vue.js application that allows users to switch the business status of a store between "open" and "close". The application will display the current status of the store and the corresponding image based on the status. Users can click on a switch button to toggle the store's business status.
👀 Preview
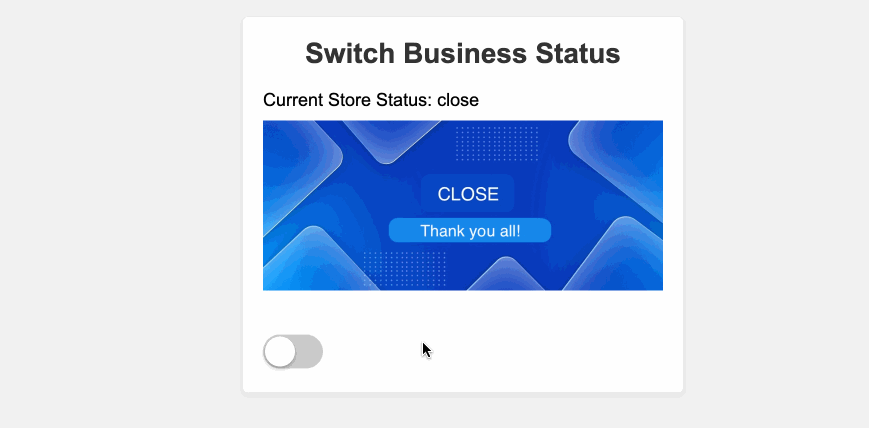
🎯 Tasks
In this project, you will learn:
- How to set up the project structure with the necessary folders and files
- How to create the Vue.js setup and define the reactive state and toggle functionality
- How to implement the HTML structure and CSS styles to display the store's business status and the switch button
- How to integrate the toggle functionality to allow users to switch the store's business status
🏆 Achievements
After completing this project, you will be able to:
- Set up a basic Vue.js project structure
- Use the
ref
function to create reactive state variables - Define and use custom functions in the Vue.js setup
- Bind data and event handlers in the HTML template
- Style the application using CSS to create a visually appealing interface