Introduction
In this project, we will create a simple web-based Markdown editor that offers live HTML preview as you type. Using libraries like Ace Editor, marked
, and highlight.js
, you'll develop an intuitive editor that not only allows writing in Markdown but also saves data across browser sessions and highlights code snippets in the preview.
๐ Preview
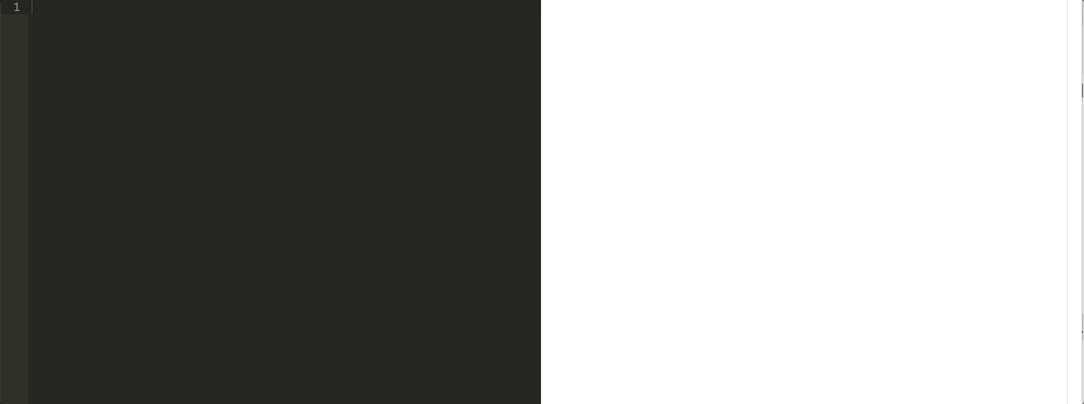
๐ฏ Tasks
In this project, you will learn:
- How to set up the HTML structure for the editor and viewer
- How to style the editor and viewer for a pleasing user experience
- How to implement the editor initialization logic
- How to parse Markdown to HTML and display it in the viewer
- How to synchronize scrolling between the editor and viewer
๐ Achievements
After completing this project, you will be able to:
- Develop a web-based Markdown editor with live HTML preview
- Utilize libraries like Ace Editor,
marked
, andhighlight.js
to enhance the editor's functionality - Implement data persistence across browser sessions
- Provide code syntax highlighting in the Markdown preview