Introduction
In this project, you will learn how to implement pagination functionality for a course list. Pagination is an essential feature in front-end web development, and this project will guide you through the process of fetching data from a JSON file, displaying the data in a paginated format, and handling the previous and next page functionality.
👀 Preview
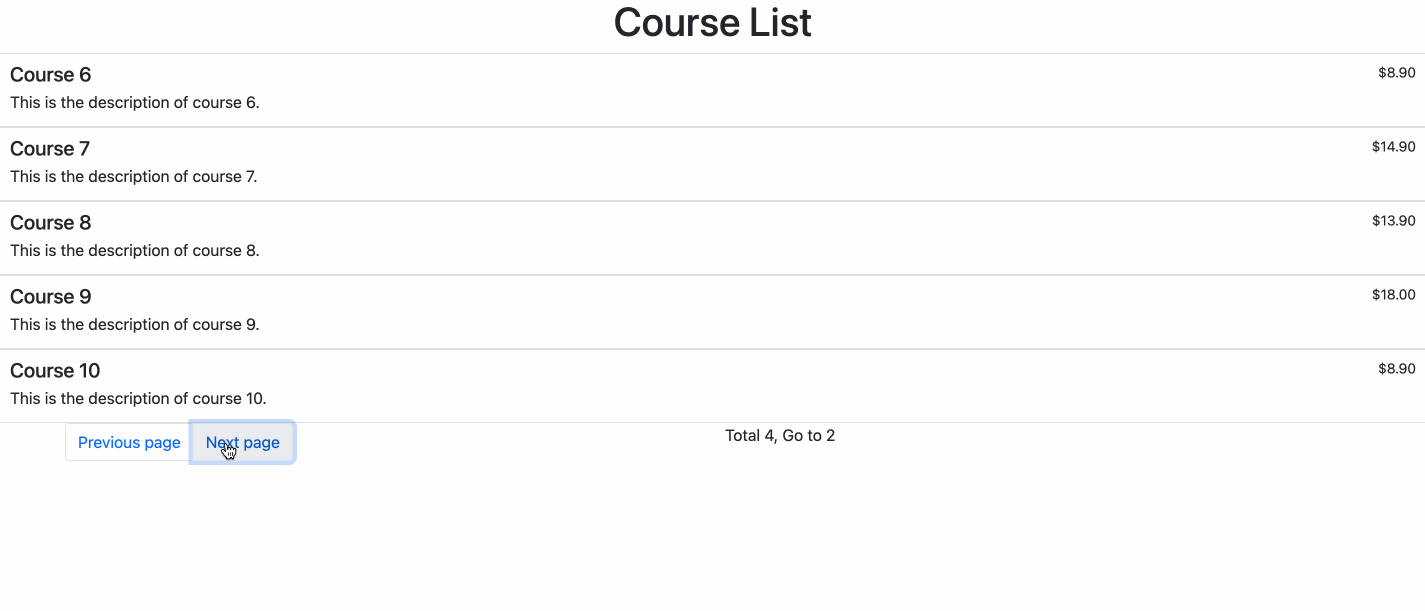
🎯 Tasks
In this project, you will learn:
- How to fetch data from a JSON file using the Axios library
- How to display the course data in a paginated format, with 5 items per page
- How to implement the functionality for the previous and next page buttons
- How to disable the previous and next page buttons when appropriate (first and last page)
- How to update the pagination display to show the current page number and the total number of pages
🏆 Achievements
After completing this project, you will be able to:
- Fetch data from a JSON file using Axios
- Implement pagination functionality for a course list
- Handle user interactions with the previous and next page buttons
- Conditionally disable buttons based on the current page
- Update the UI to display the current page and total pages