Introduction
In this project, you will learn how to build a pagination component, which is a commonly used component in web applications. The pagination component helps reduce the query time on the backend and does not affect the performance of page rendering due to too much data loading.
👀 Preview
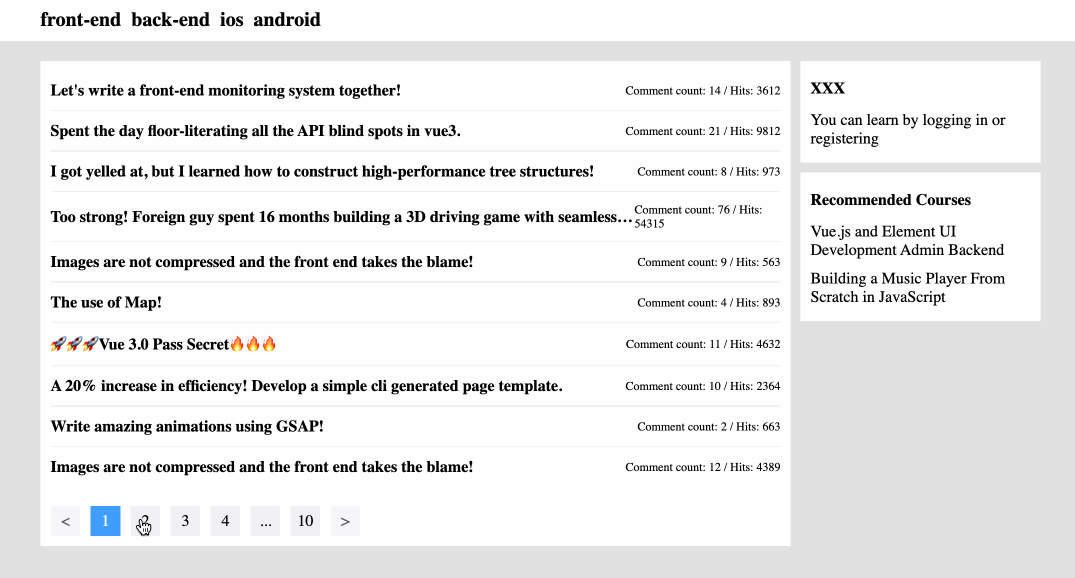
🎯 Tasks
In this project, you will learn:
- How to implement the
ajax
function to handle data requests and retrieve the data for the current page and the total number of pages. - How to implement the
initEvents
function to bind events to the buttons of the pagination component. - How to implement the
createPaginationIndexArr
function to generate a pagination array based on the function arguments. - How to implement the
renderPagination
function to generate the string template for the pagination component. - How to render the content based on the current page.
🏆 Achievements
After completing this project, you will be able to:
- Build a reusable pagination component in JavaScript.
- Use Axios to make HTTP requests and handle the response data.
- Generate a pagination array based on the current page, total pages, and the maximum number of page buttons to display.
- Update the pagination component and the content based on the current page.