Introduction
In this project, you will learn how to build a web application that allows users to search for and display beautiful sentences from literature in real-time. The application will fetch the data from a JSON file, and use Vue.js to implement the search functionality and display the results.
👀 Preview
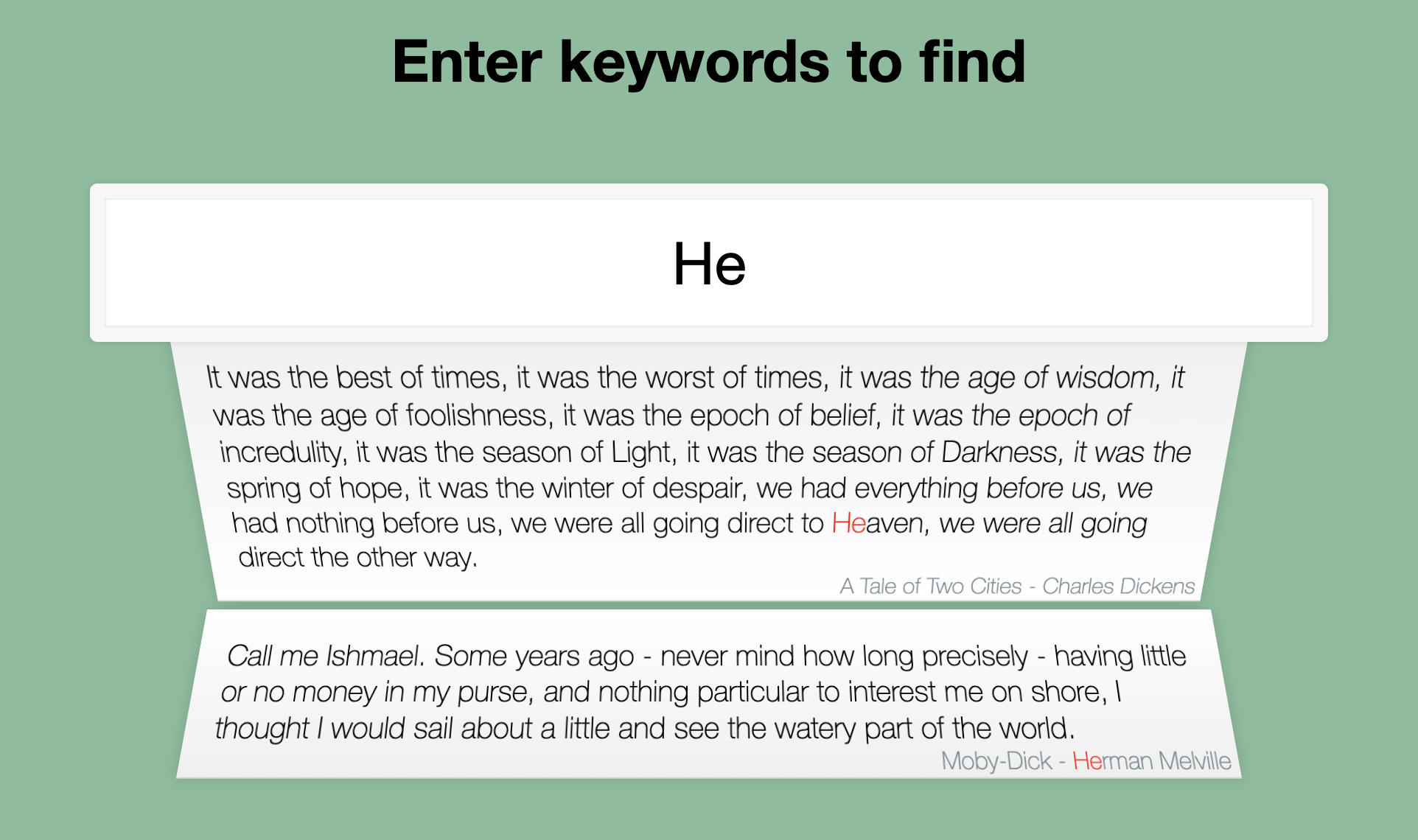
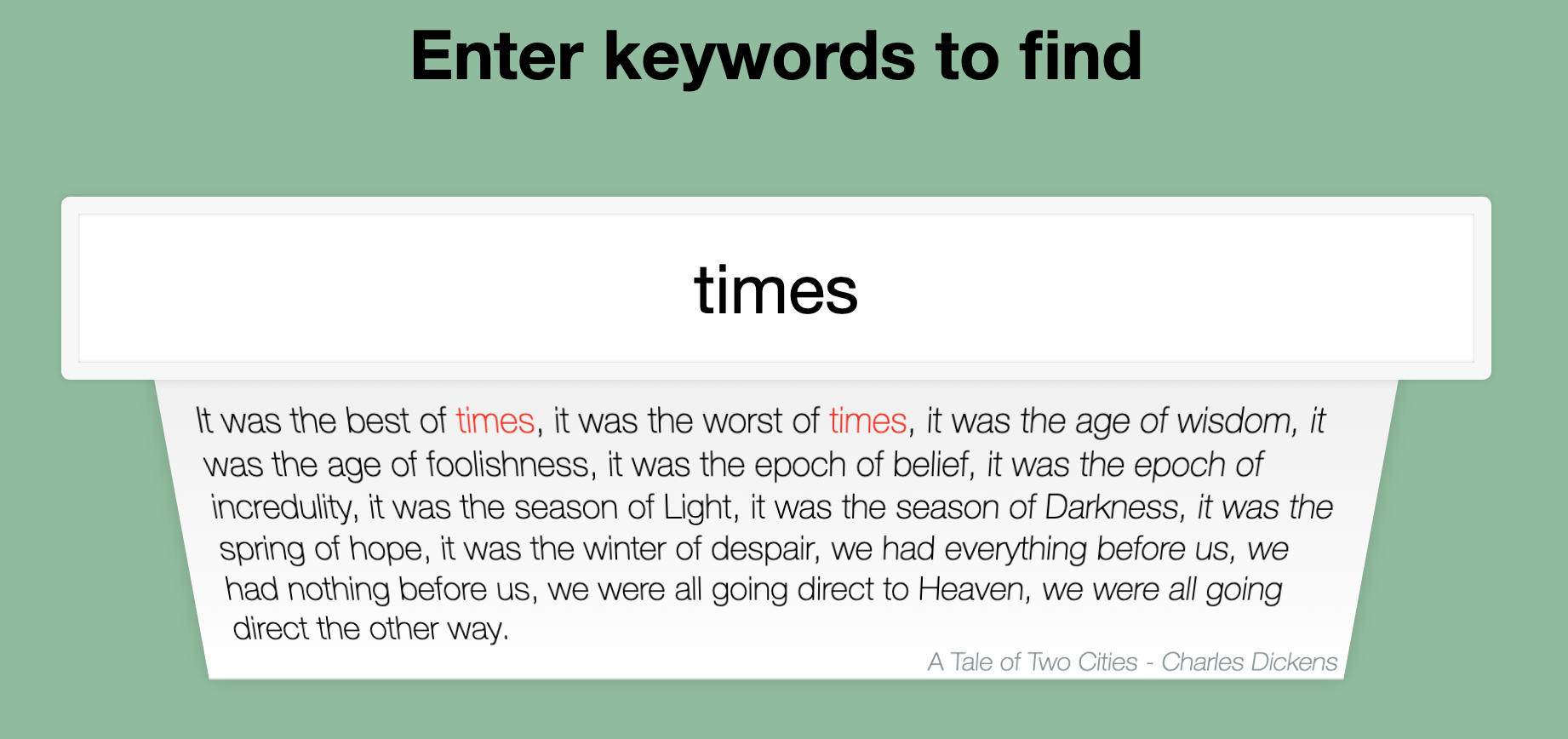
🎯 Tasks
In this project, you will learn:
- How to set up the project and understand the provided files and directories
- How to implement the data request to fetch the data from the
data.json
file - How to implement the real-time search functionality to display the matching sentences
- How to add some finishing touches to the project by styling the web page
🏆 Achievements
After completing this project, you will be able to:
- Set up a web development project with HTML, CSS, and JavaScript
- Use Vue.js to build a responsive and interactive web application
- Fetch data from a JSON file and display it on the web page
- Implement real-time search functionality and highlight the matching keywords
- Style a web page using CSS to improve the overall appearance and user experience