Introduction
In this project, you will learn how to build a dynamic menu tree search functionality using Vue.js. The project involves fetching secondary menu data from a JSON file, implementing a fuzzy search feature, and displaying the filtered menu tree on the page.
👀 Preview
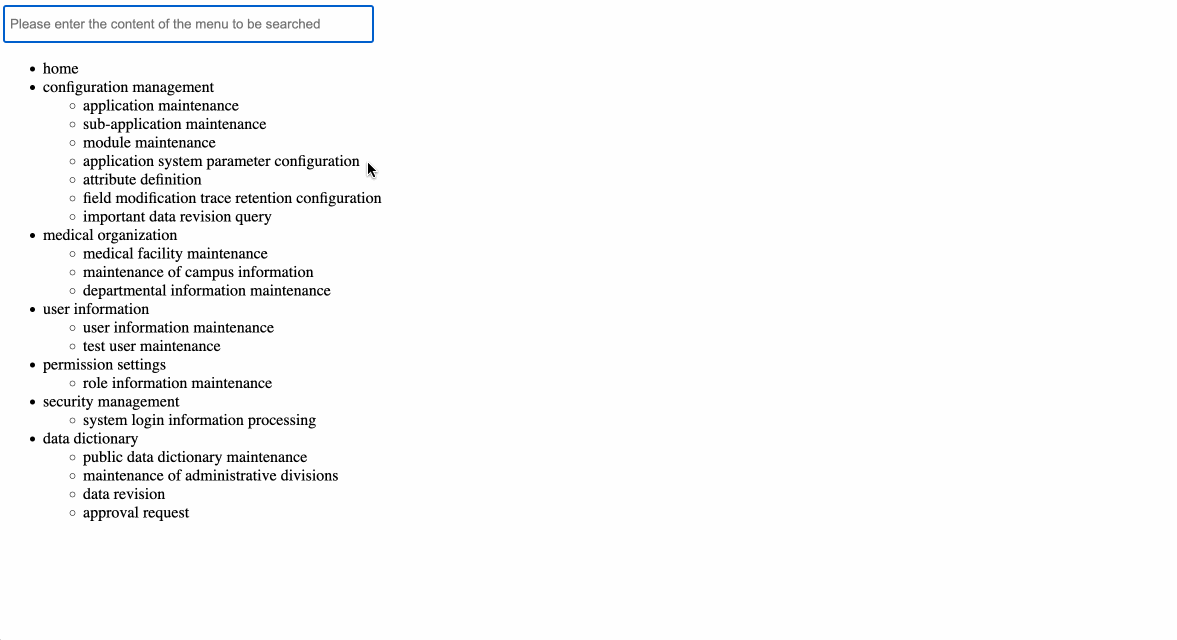
🎯 Tasks
In this project, you will learn:
- How to fetch secondary menu data from a JSON file using the
axios
library - How to implement a search functionality to filter the menu items based on the user's input
- How to display the filtered menu tree on the page, highlighting the matching text
🏆 Achievements
After completing this project, you will be able to:
- Develop a dynamic and interactive user interface using Vue.js
- Fetch data from a JSON file and handle asynchronous data fetching
- Implement a fuzzy search feature to filter data based on user input
- Display a hierarchical menu tree structure and highlight the matching text