Introduction
In this project, you will learn how to implement a virtual scrolling list using Vue.js. Virtual scrolling is a technique that only renders the visible area of a large dataset, rather than rendering or partially rendering the data in the non-visible area. This achieves extremely high rendering performance and is suitable for rendering a large number of data with a small volume.
👀 Preview
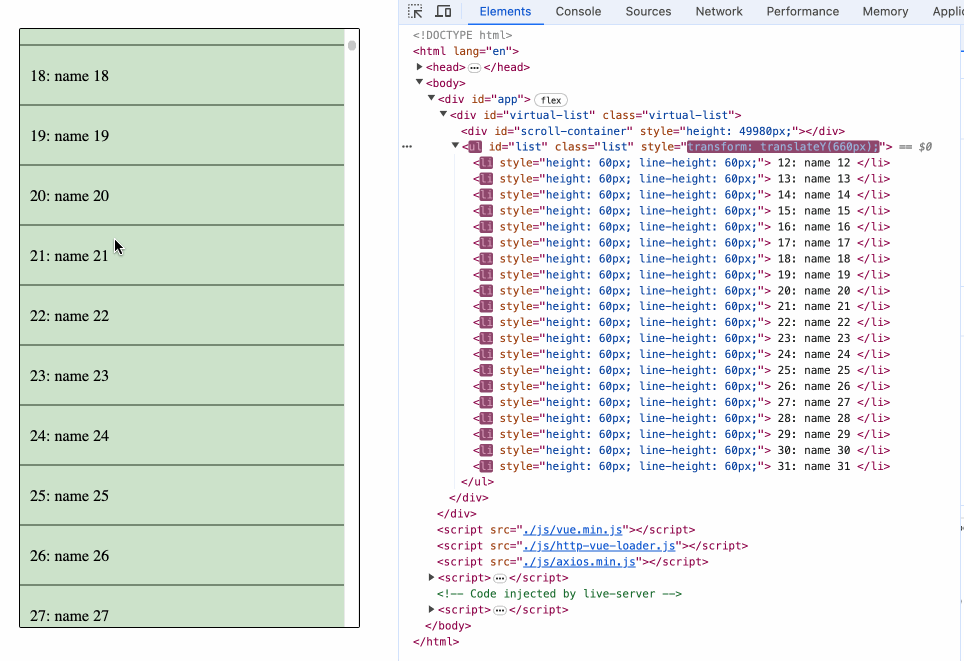
🎯 Tasks
In this project, you will learn:
- How to load and render data asynchronously using the
axios
library - How to implement virtual scrolling functionality to only render the visible list items
- How to calculate the start and end indices of the visible list items based on the current scroll position
- How to optimize the rendering performance by using a buffer to render additional list items outside the visible area
🏆 Achievements
After completing this project, you will be able to:
- Implement virtual scrolling functionality to improve rendering performance
- Load and render data asynchronously in a Vue.js component
- Calculate the visible list items based on the current scroll position
- Use a buffer to prevent a white screen during scrolling