Introduction
In this project, you will learn how to implement an online shopping cart with drag and drop functionality. This project aims to help you understand and apply the concepts of Vue.js and the drag and drop APIs provided by web browsers.
👀 Preview
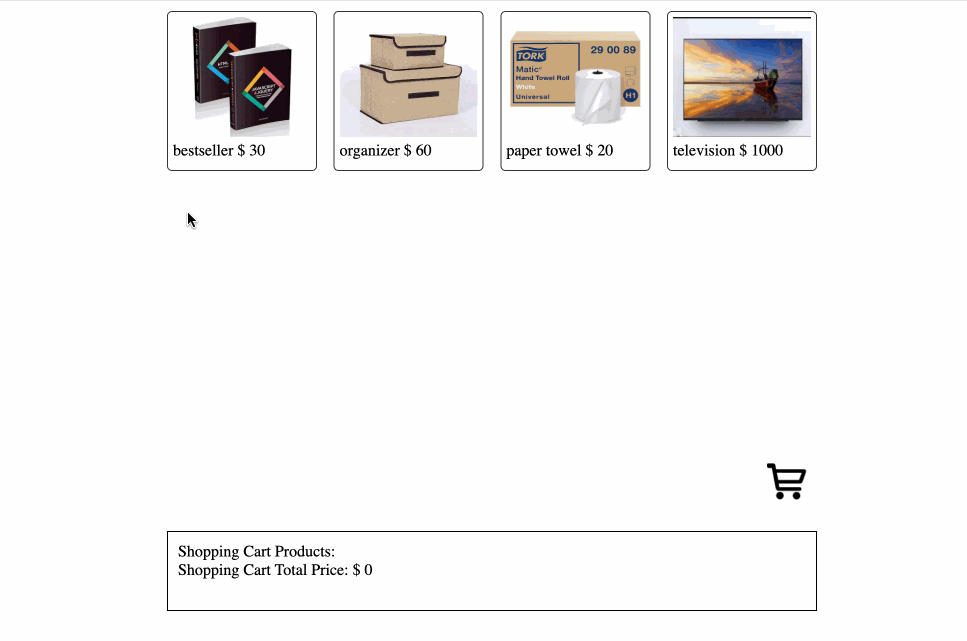
🎯 Tasks
In this project, you will learn:
- How to set up the project and familiarize yourself with the provided files and structure.
- How to implement the drag and drop functionality for the online shopping cart, allowing users to add products to the cart.
- How to display the shopping cart information, including the number of products, the product details, and the total price.
- How to test the online shopping cart and ensure the functionality works as expected.
🏆 Achievements
After completing this project, you will be able to:
- Use Vue.js to build a web application.
- Implement drag and drop functionality using the web browser's built-in APIs.
- Display dynamic data and update the user interface based on user interactions.
- Write clean and maintainable code by organizing your application into components.