Introduction
In this project, you will learn how to implement a login verification feature using AJAX and JSON data transfer. The project involves creating a login form, handling the form submission with AJAX, and validating the user's login credentials on the server-side.
👀 Preview
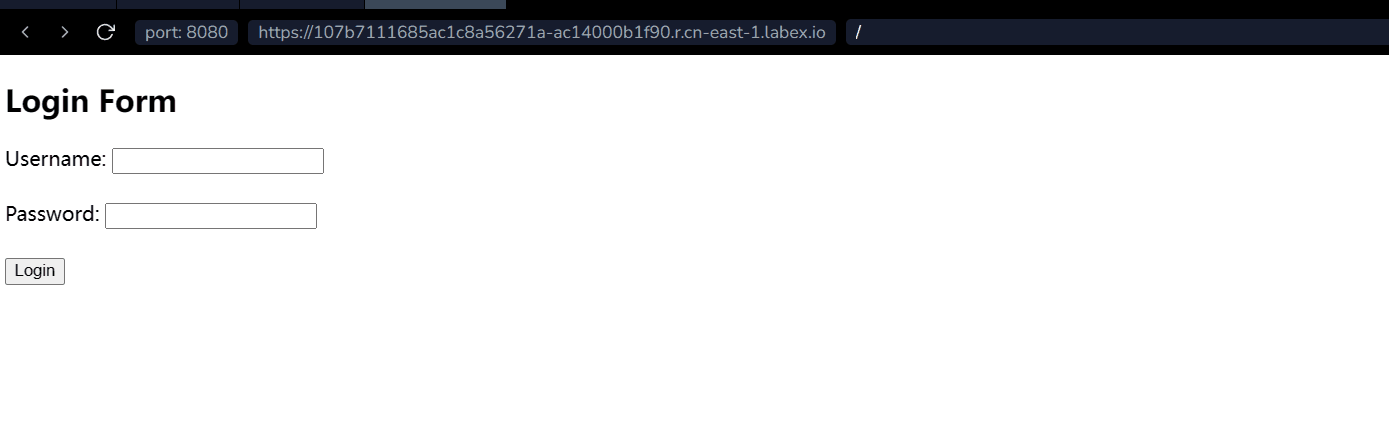
🎯 Tasks
In this project, you will learn:
- How to prepare the project environment and set up the necessary files and dependencies.
- How to implement the login form in the
AjaxJson.jsp
page using HTML. - How to handle the login form submission using jQuery and AJAX.
- How to encapsulate the login data in JSON format and send it to the server.
- How to receive and process the JSON response from the server to determine the login status.
- How to redirect the user to the appropriate page based on the login result.
🏆 Achievements
After completing this project, you will be able to:
- Use AJAX to send data to the server without a full page refresh.
- Work with JSON data format for data transfer between the client and server.
- Handle form submission and user input validation on the client-side.
- Process the server's response and update the user interface accordingly.
- Integrate client-side and server-side components to create a complete login verification feature.