Introduction
In this project, you will learn how to implement an input search suggestions feature using Vue.js 2.x. This feature is commonly used in web applications to provide users with a list of matching data as they type in the search input.
👀 Preview
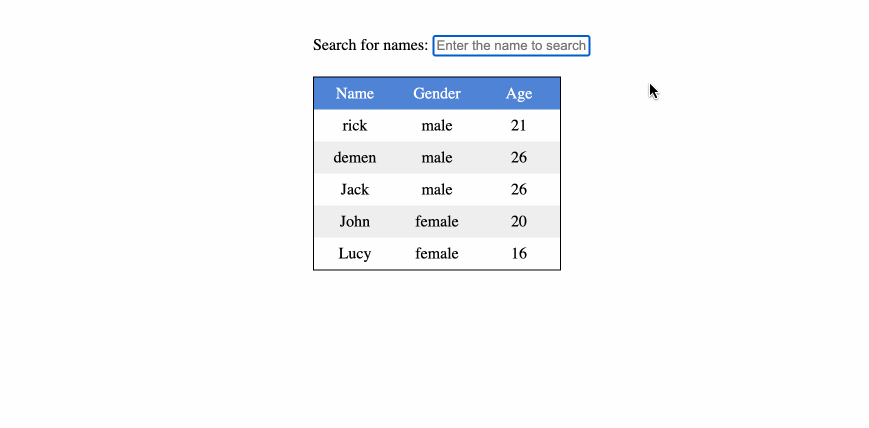
🎯 Tasks
In this project, you will learn:
- How to capitalize the first letter of the table headers in the data table
- How to implement the search suggestions functionality based on the user's input
🏆 Achievements
After completing this project, you will be able to:
- Manipulate the DOM using Vue.js directives like
v-for
andv-model
- Create computed properties in Vue.js to filter and display the search results
- Apply basic styling to the table and search input