Introduction
In this project, you will learn how to implement a custom form validator using Vue.js. The custom form validator allows you to validate form fields and check the validity of the data before the user submits the form.
👀 Preview
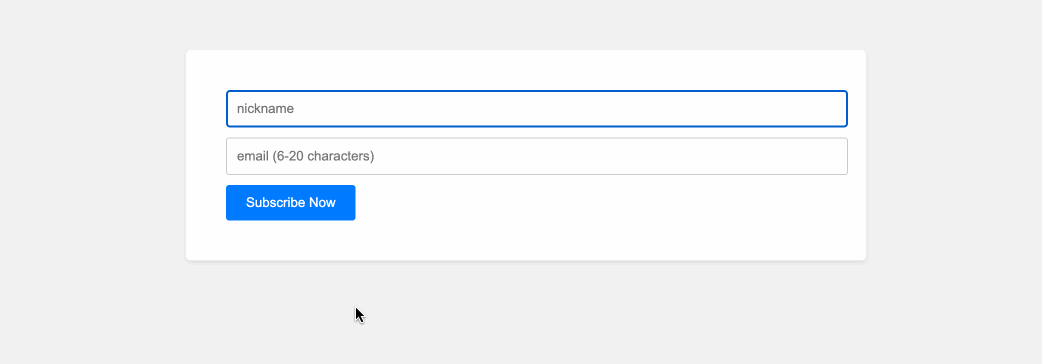
🎯 Tasks
In this project, you will learn:
- How to implement the
FormInput
component to update thev-model
value of the component when the value of the input field changes. - How to implement the
is_email
function to validate the email address based on specific rules. - How to implement the
validateForm
function to validate the form data based on the defined validation rules.
🏆 Achievements
After completing this project, you will be able to:
- Create a custom form validator in Vue.js.
- Validate form fields using custom validation rules.
- Handle form validation errors and display them to the user.