Introduction
In this project, you will learn how to configure logging and use batch aliasing for entity classes in a MyBatis-based project.
👀 Preview
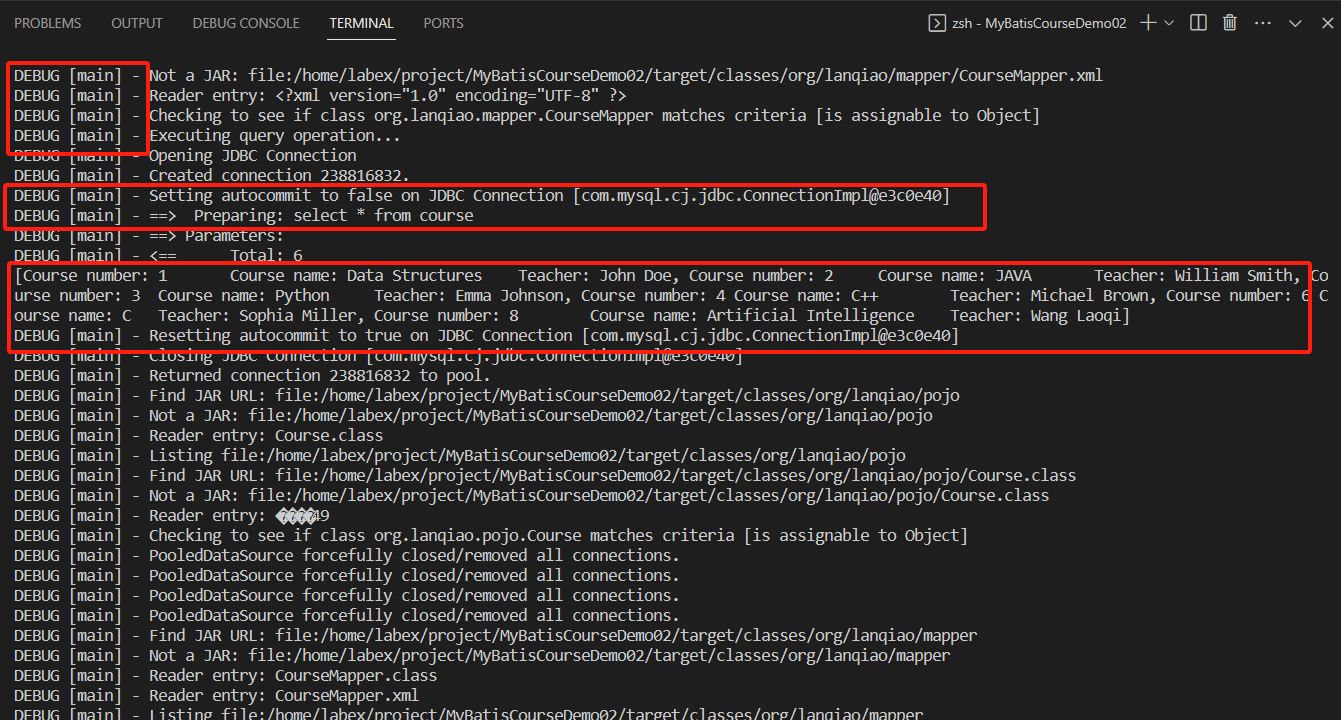
🎯 Tasks
In this project, you will learn:
- How to configure the logging component using the log4j library
- How to add logging dependencies to the project
- How to use the logging component in your code to log messages
- How to implement batch aliasing for entity classes in the MyBatis configuration file
🏆 Achievements
After completing this project, you will be able to:
- Set up and configure the logging component in a Java project
- Use the logging component to log messages at different levels (e.g., DEBUG, INFO, ERROR)
- Apply batch aliasing to simplify the usage of entity classes in your MyBatis code