Introduction
In this project, we will create a Sudoku game using Python and the Pygame library. The game will generate a Sudoku grid of the specified difficulty level and let players solve the puzzle by filling in the empty cells with numbers. The game will provide features like selecting difficulty, highlighting selected cells, and checking if the grid is complete.
👀 Preview
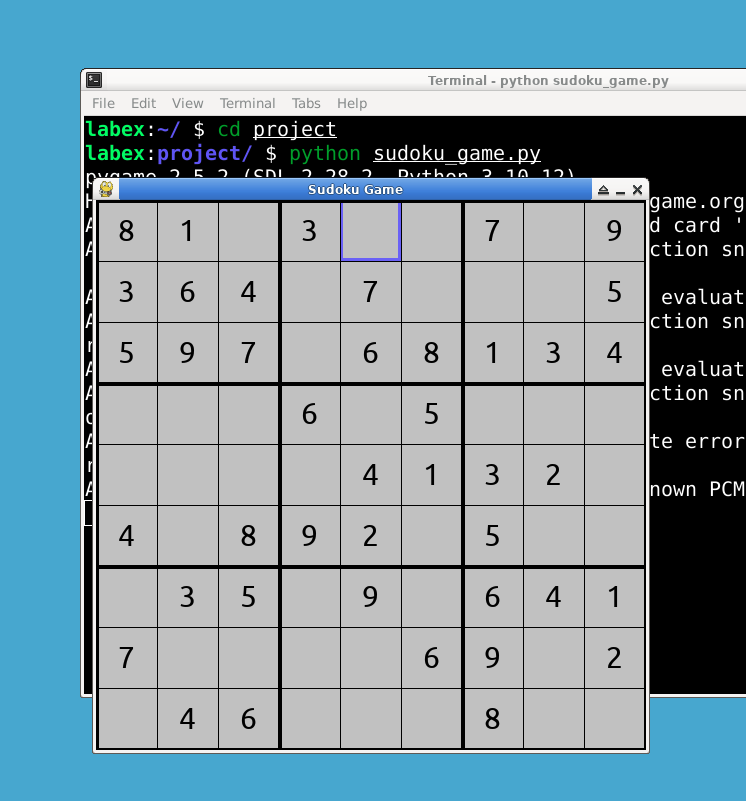
🎯 Tasks
In this project, you will learn:
- How to import required libraries
- How to initialize PyGame
- How to define colors
- How to set game window dimensions and title
- How to create the game window
- How to load fonts
- How to generate a Sudoku grid
- How to solve the Sudoku grid using backtracking algorithm
- How to remove numbers from the grid based on difficulty
- How to draw the Sudoku grid on the game window
- How to check if the grid is fully filled
- How to get the cell coordinates under mouse position
- How to select the difficulty level
- How to implement the main game loop
🏆 Achievements
After completing this project, you will be able to:
- Use the Pygame library for game development in Python
- Generate a Sudoku grid of a specified difficulty level
- Solve a Sudoku grid using the backtracking algorithm
- Handle mouse and keyboard events in Pygame
- Draw shapes and text on the game window
- Implement the main game loop in Pygame