Introduction
In this project, you'll learn how to create a simple 2048 game using Python and the Tkinter library for the graphical user interface. 2048 is a popular sliding puzzle game where you combine tiles to reach the tile with a value of 2048. While this project won't create the most modern and beautiful user interface, it will provide a solid foundation that you can build upon to enhance the aesthetics.
👀 Preview
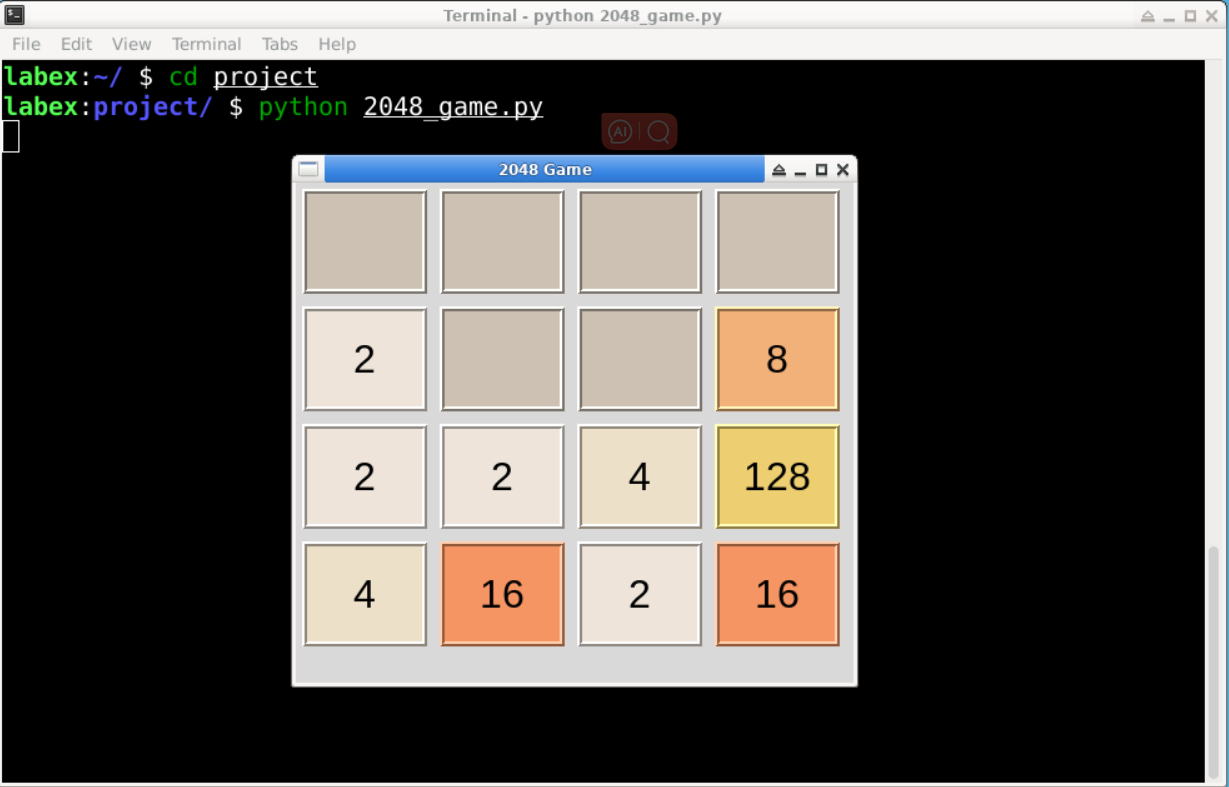
🎯 Tasks
In this project, you will learn:
- How to import necessary libraries for the game
- How to create the Game2048 class to handle the game's logic and user interface
- How to draw the game grid using Tkinter
- How to spawn the initial tiles on the grid
- How to update the user interface to reflect the current state of the game grid
- How to define tile colors based on their values
- How to handle key presses to move the tiles
- How to define methods to move tiles in different directions
- How to check if the game is over
🏆 Achievements
After completing this project, you will be able to:
- Use the Tkinter library to create a graphical user interface
- Handle key presses and trigger corresponding actions
- Update the user interface based on the game state
- Implement game logic for tile movements and merging
- Check if a game is over