Introduction
In this project, you will learn how to create a Linux system monitor using Python and the Tkinter library. The system monitor will display real-time information about the CPU, RAM, disk usage, and network statistics.
ð Preview
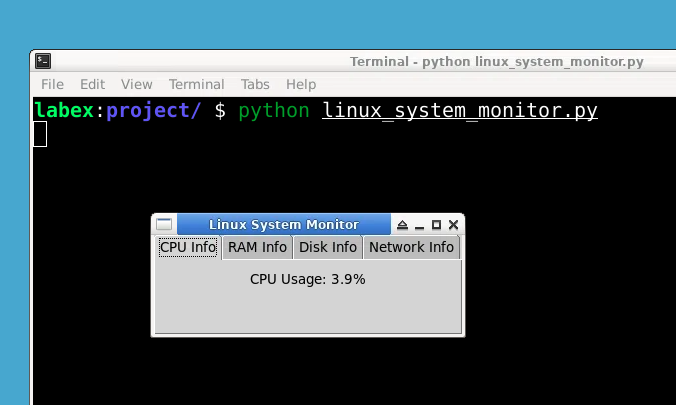
ðŊ Tasks
In this project, you will learn:
- How to create a graphical user interface (GUI) using Tkinter.
- How to use the
psutil
library to get real-time system information. - How to display CPU usage, RAM usage, disk usage, and network statistics.
ð Achievements
After completing this project, you will be able to:
- Create a GUI in Python using Tkinter.
- Use the
psutil
library to get system information. - Update labels with real-time data.
- Organize information using frames and notebook widgets.