Introduction
In this project, we will create an image viewer application using Python and Tkinter. The image viewer will allow you to open an image file, display it, and perform actions such as zooming in, zooming out, and rotating the image. We will use the PIL (Python Imaging Library) library to handle image operations and Tkinter for creating the graphical user interface.
ð Preview
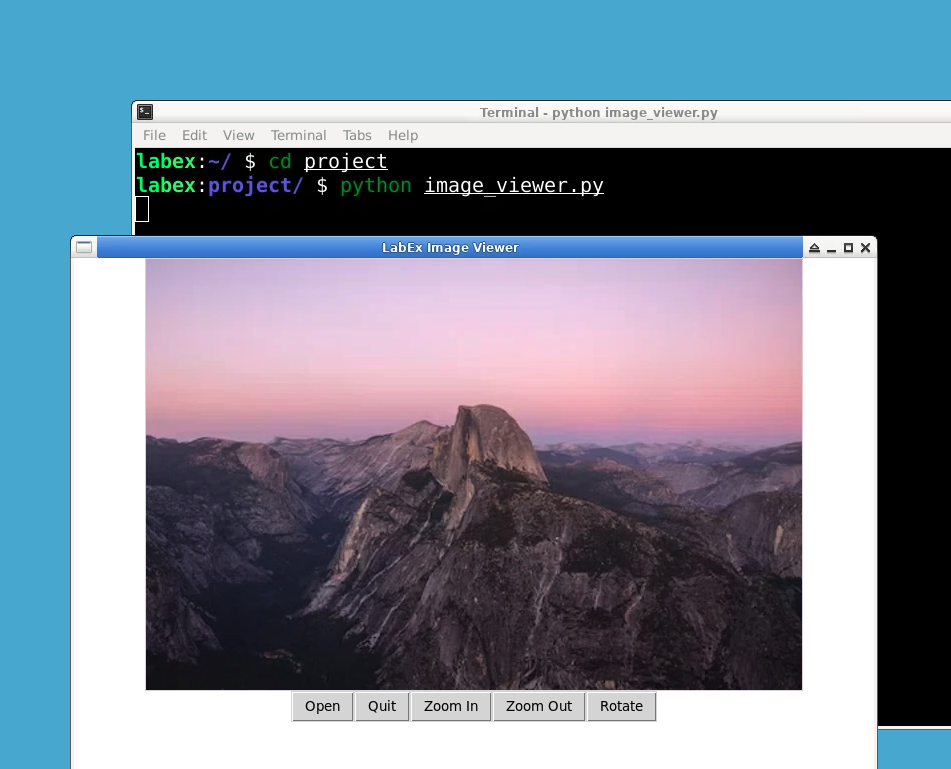
ðŊ Tasks
In this project, you will learn:
- How to create a GUI application using Tkinter.
- How to handle image loading and display using PIL.
- How to implement zoom in, zoom out, and rotate functionality for the displayed image.
ð Achievements
After completing this project, you will be able to:
- Develop a functional image viewer application using Python and Tkinter.
- Integrate image processing capabilities using the PIL library.
- Implement basic image manipulation features such as zooming and rotating.