Introduction
In this project, you will learn how to build a login functionality using Vue.js and Vuex. The project aims to help you understand the integration of Vue.js components with a Vuex-based state management system.
👀 Preview
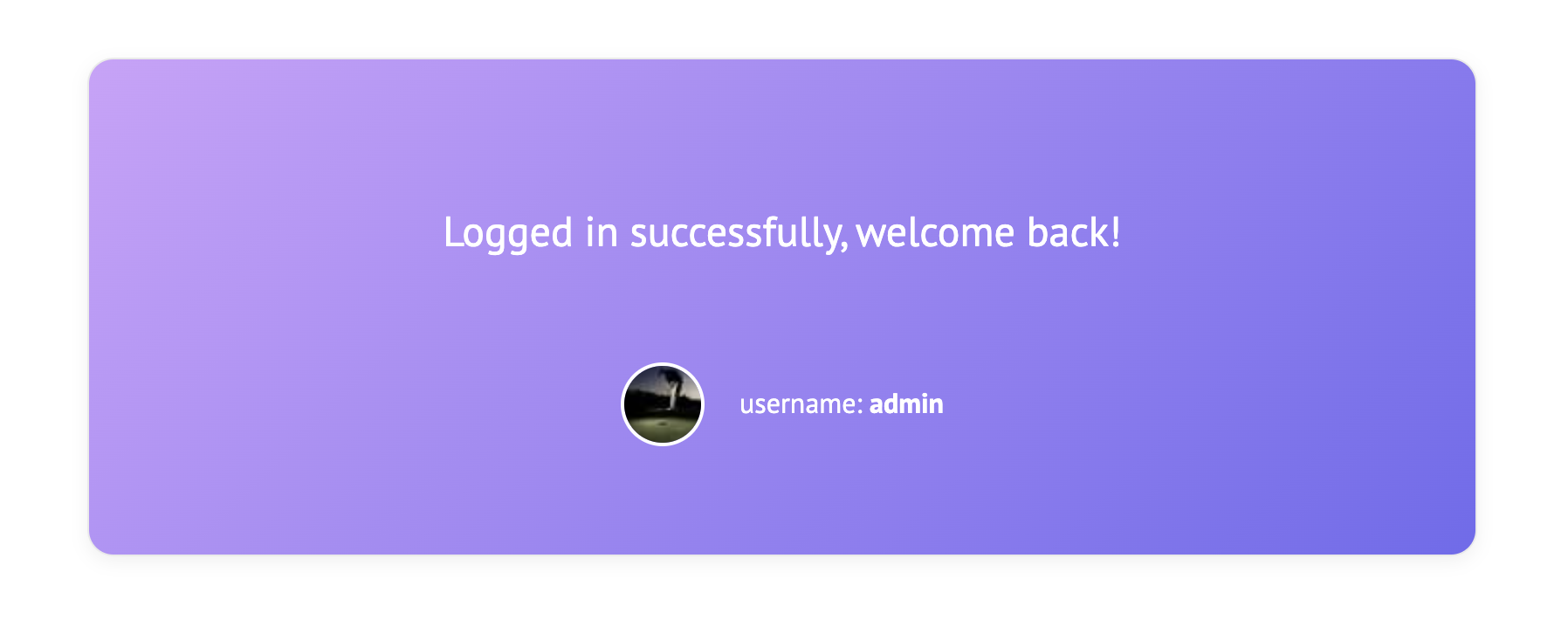
🎯 Tasks
In this project, you will learn:
- How to understand the project structure and the role of each file
- How to identify the issue in the login function
- How to fix the login function by modifying the Vuex store and the Vue component
🏆 Achievements
After completing this project, you will be able to:
- Understand how to structure a Vue.js project with Vuex
- Apply Vuex to manage the application state
- Troubleshoot and fix issues in a Vue.js application
- Integrate Vuex with Vue.js components